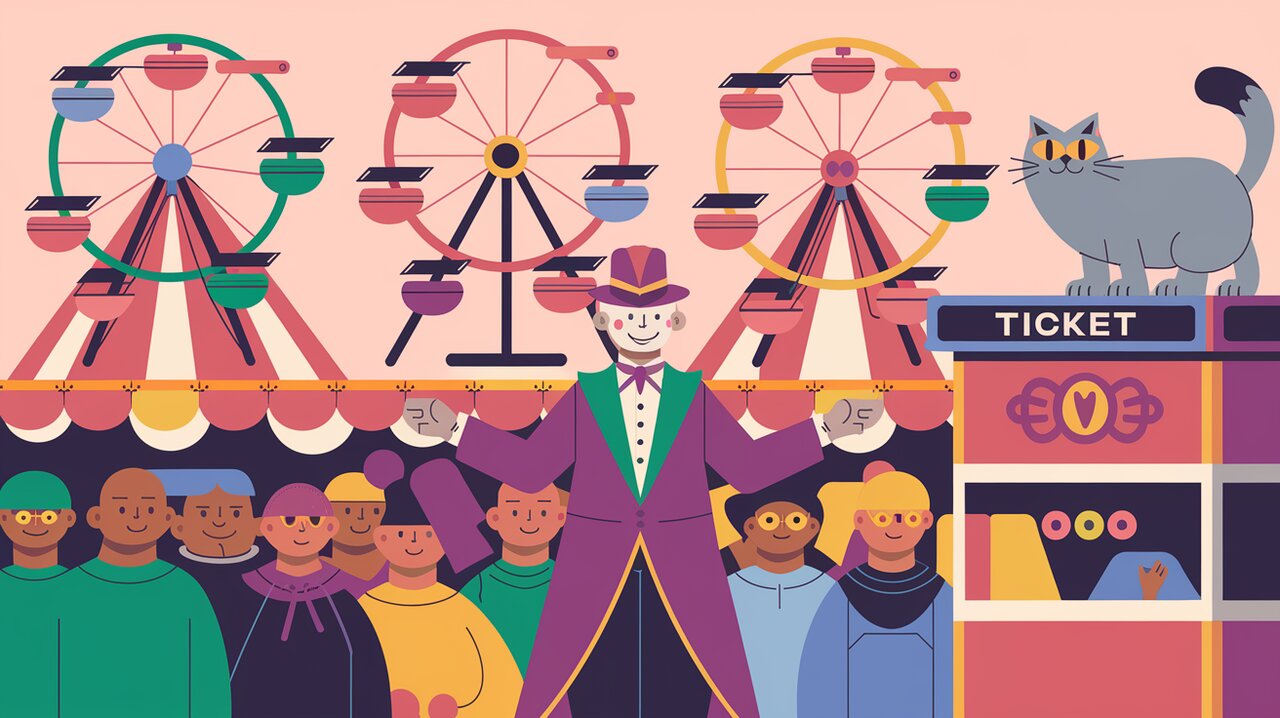
Redux-Multi: The Action Dispatch Party Planner
In the grand circus of Redux state management, action creators often find themselves performing solo acts, dispatching one action at a time. But what if your Redux application needs to execute a series of actions in quick succession? Enter Redux Multi, the middleware that transforms your action creators into a well-coordinated ensemble, capable of dispatching multiple actions with a single function call.
The Multi-Action Magic Show
Redux Multi brings two main attractions to your Redux carnival:
- Simplified Action Creators: Say goodbye to cluttered action creators that dispatch multiple actions through complex chains or async operations.
- Improved Readability: Your action logic becomes more transparent and easier to understand at a glance.
Let’s set up our Redux circus tent and see how Redux Multi can dazzle your audience!
Setting the Stage
Before we can start our multi-action performance, we need to install Redux Multi in our project. Grab your package manager of choice, and let’s get the show on the road!
Using npm:
npm install redux-multi
Or if you prefer yarn:
yarn add redux-multi
The Grand Opening: Basic Setup
To kick off our spectacular show, we need to apply the Redux Multi middleware to our Redux store. Here’s how we prepare the stage:
import { createStore, applyMiddleware } from 'redux';
import multi from 'redux-multi';
import rootReducer from './reducers';
const store = createStore(rootReducer, applyMiddleware(multi));
With this setup, our Redux store is now equipped to handle multiple actions dispatched from a single action creator. It’s like giving our Redux ringmaster the ability to juggle multiple balls at once!
The Main Act: Dispatching Multiple Actions
Now that our stage is set, let’s see Redux Multi in action. Imagine we’re creating a user signup process where we need to show a loading indicator and then create the user. Traditionally, we’d need to dispatch these actions separately, but with Redux Multi, we can combine them into a single, elegant performance:
function signupUser(userData: UserData): Action[] {
return [
{ type: 'SET_LOADING', payload: true },
{ type: 'CREATE_USER', payload: userData }
];
}
// Usage in a component
dispatch(signupUser(newUserData));
In this act, signupUser
returns an array of actions. Redux Multi takes this array and dispatches each action in order, creating a seamless flow of state updates. It’s like watching a perfectly choreographed dance routine!
Advanced Tricks: Async Actions and Conditionals
Redux Multi isn’t just about simple sequences. It can handle more complex routines as well. Let’s up the ante with some async actions and conditional logic:
The Disappearing User Trick
function deleteUserWithConfirmation(userId: string) {
return async (dispatch: Dispatch) => {
const confirmDelete = await showConfirmDialog('Are you sure?');
if (confirmDelete) {
return dispatch([
{ type: 'SET_LOADING', payload: true },
await deleteUserFromAPI(userId),
{ type: 'REMOVE_USER', payload: userId },
{ type: 'SET_LOADING', payload: false }
]);
}
return dispatch({ type: 'DELETE_CANCELLED' });
};
}
In this more elaborate performance, we first show a confirmation dialog. If the user confirms, we dispatch a series of actions: setting a loading state, calling an API, updating our local state, and finally turning off the loading indicator. If the user cancels, we dispatch a single action. It’s like a magic trick where the outcome depends on the audience’s choice!
The Levitating State Update
function updateUserProfile(userId: string, updates: Partial<UserProfile>) {
return (dispatch: Dispatch, getState: () => RootState) => {
const currentUser = getState().users.byId[userId];
if (currentUser) {
return dispatch([
{ type: 'UPDATE_USER', payload: { id: userId, updates } },
updates.email ? { type: 'SEND_VERIFICATION_EMAIL', payload: updates.email } : null,
{ type: 'LOG_PROFILE_UPDATE', payload: { userId, timestamp: Date.now() } }
].filter(Boolean));
}
return dispatch({ type: 'USER_NOT_FOUND', payload: userId });
};
}
This trick showcases how Redux Multi can handle conditional actions within an array. We update the user profile, conditionally send a verification email if the email was changed, and always log the update. The filter(Boolean)
at the end removes any null
actions, ensuring our performance is smooth and error-free.
The Grand Finale
As the curtains fall on our Redux Multi extravaganza, we can see how this simple yet powerful middleware transforms the way we handle complex action dispatching in Redux. By allowing us to group related actions together, Redux Multi helps create more readable, maintainable, and efficient code.
Whether you’re managing a small state circus or a full-scale Redux carnival, Redux Multi provides the tools to keep your action creators organized and your state updates synchronized. So why juggle multiple dispatch calls when you can orchestrate a symphony of actions with Redux Multi? Give it a try in your next Redux project, and watch your state management routines transform from a solo act into a spectacular ensemble performance!