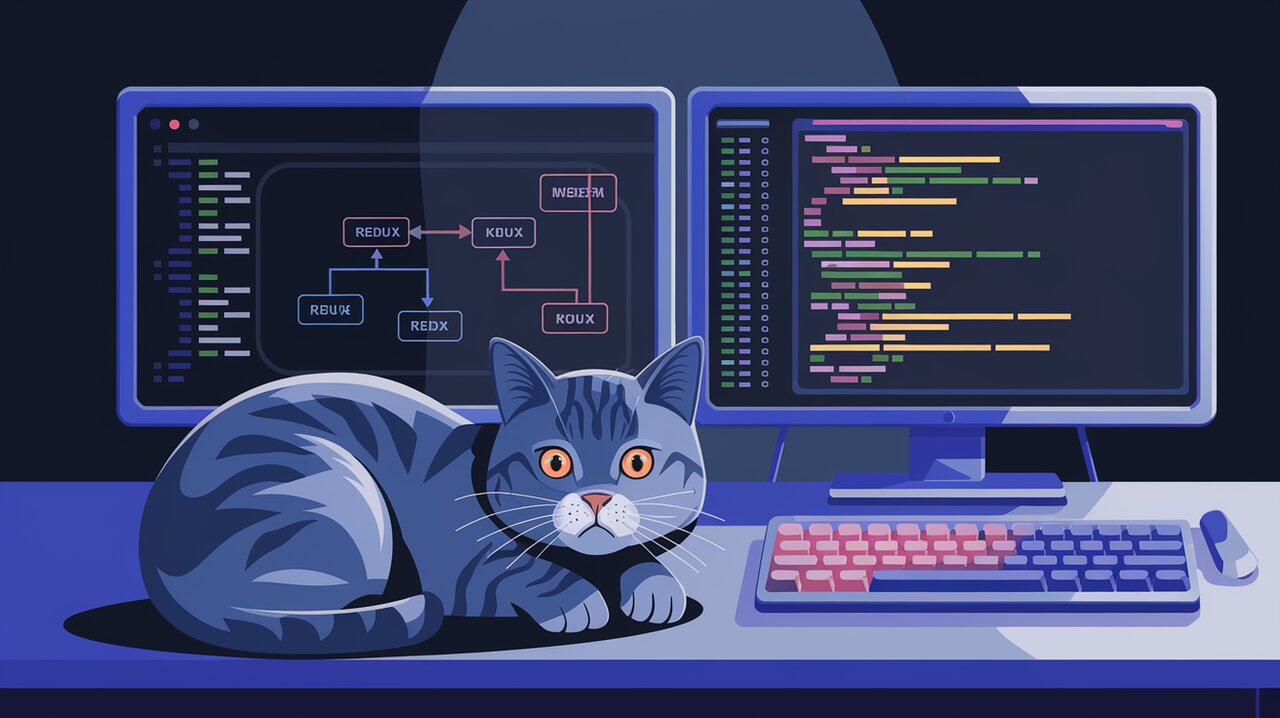
Redux-Node-Logger: Console Wizardry for Node-based Redux Apps
In the realm of Redux-powered Node.js applications, keeping track of state changes and action flows can be a daunting task. Enter Redux-Node-Logger, a nifty middleware that acts as your personal console wizard, illuminating the inner workings of your Redux store with colorful, formatted logs. This tool is especially valuable for developers working on server-side Redux applications, providing a clear window into the state mutations and action dispatches that occur during runtime.
Illuminating Features
Redux-Node-Logger comes packed with a set of features designed to enhance your debugging experience:
- Colorful console output for improved readability
- Customizable log formatting with arrow icons
- Detailed logging of previous state, action, and next state
- Support for Immutable.js data structures
- Predicate function for conditional logging
Summoning the Logger
To bring this magical middleware into your project, you’ll need to invoke the following incantation in your terminal:
npm install --save-dev redux-node-logger
Or if you prefer the yarn scrolls:
yarn add --dev redux-node-logger
Casting Your First Spell
Once you’ve acquired the Redux-Node-Logger grimoire, it’s time to integrate it into your Redux setup. Here’s a basic enchantment to get you started:
import { createStore, applyMiddleware, compose } from 'redux';
import createNodeLogger from 'redux-node-logger';
import rootReducer from './reducers';
const configureStore = () => {
if (process.env.NODE_ENV === 'development' && !process.env.BROWSER) {
const nodeLogger = createNodeLogger();
const enhancer = compose(applyMiddleware(nodeLogger));
return createStore(rootReducer, enhancer);
}
return createStore(rootReducer);
};
export default configureStore;
In this incantation, we’re creating a store enhancer that applies the Redux-Node-Logger middleware only in a Node.js development environment. This ensures that our logging spells don’t affect production performance.
Customizing Your Logging Rituals
Redux-Node-Logger allows you to tailor its output to your liking. You can adjust colors, change arrow icons, and even decide when logging should occur. Here’s how you can customize your logging rituals:
const options = {
downArrow: '▼',
rightArrow: '▶',
messageColor: 'bright-yellow',
prevColor: 'grey',
actionColor: 'bright-blue',
nextColor: 'green',
predicate: (getState, action) => action.type !== 'IGNORE_ME'
};
const nodeLogger = createNodeLogger(options);
This spell configures the logger with custom colors and arrows, and adds a predicate function to filter out specific actions from being logged.
Advanced Incantations
Taming Immutable Beasts
If your Redux store houses Immutable.js data structures, fear not! Redux-Node-Logger has been trained to handle these magical beasts. It automatically converts Immutable objects to plain JavaScript objects during logging, ensuring readable output:
import { Map } from 'immutable';
const initialState = Map({ wizardName: 'Merlin', spellCount: 42 });
// Your reducer
const rootReducer = (state = initialState, action) => {
switch (action.type) {
case 'CAST_SPELL':
return state.update('spellCount', count => count + 1);
default:
return state;
}
};
When an action is dispatched, Redux-Node-Logger will display the Immutable state in a friendly, readable format.
Selective Logging Rituals
For more granular control over your logging, you can use the predicate option to create sophisticated filtering spells:
const nodeLogger = createNodeLogger({
predicate: (getState, action) => {
const state = getState();
// Only log actions when the wizard's spell count is even
return state.get('spellCount') % 2 === 0;
}
});
This advanced incantation will only log actions when the wizard’s spell count is even, allowing you to focus on specific state conditions during debugging.
Concluding the Ritual
Redux-Node-Logger serves as an invaluable companion in the quest to master Redux in Node.js environments. By providing clear, colorful insights into your application’s state changes and action flows, it empowers developers to debug with confidence and ease. Whether you’re battling complex state management issues or simply seeking to understand your app’s behavior better, this middleware stands ready to illuminate the path forward.
Remember, while Redux-Node-Logger is a powerful ally in development, it’s wise to disable it in production to maintain optimal performance. Use it wisely, and may your Redux journeys be filled with clarity and insight!
For more enchanting tools to enhance your React and Redux development, don’t forget to explore our articles on Redux Devtools Time Travel Symphony and Redux Logger Detective: Tracking State Changes. These complementary artifacts will further empower your debugging arsenal and help you craft even more robust Redux applications.