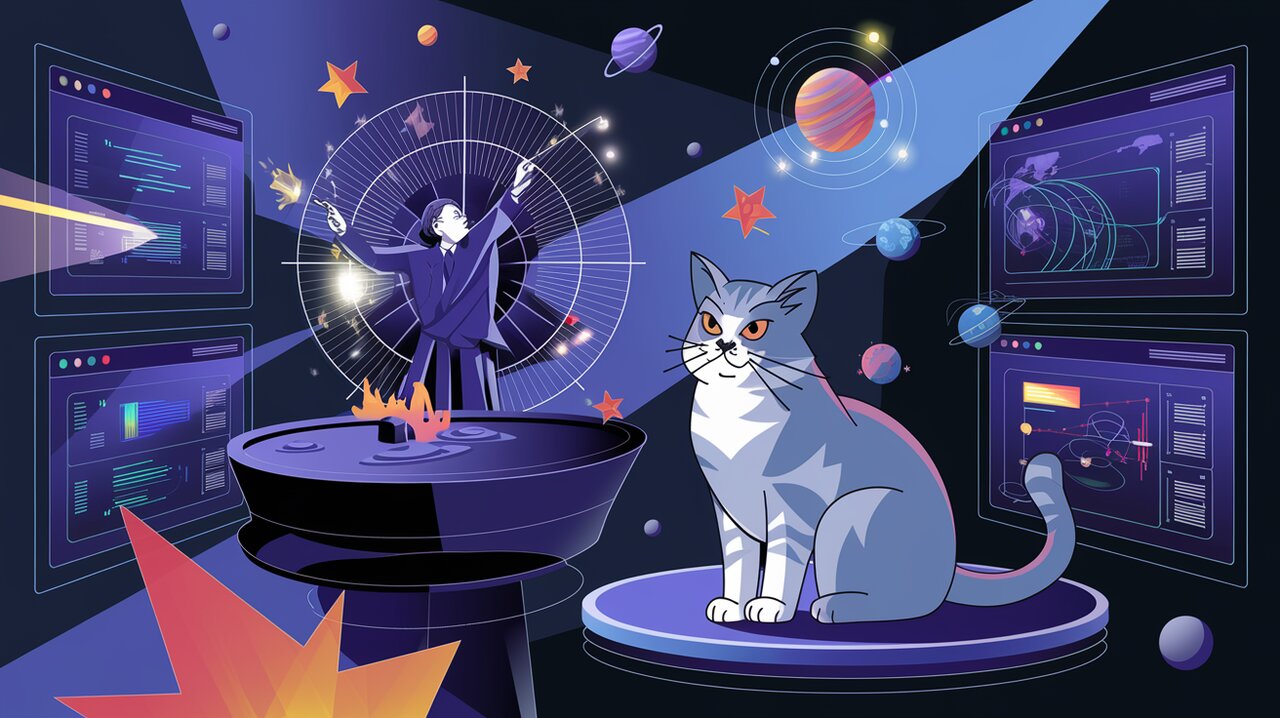
Redux-Observable: Orchestrating Cosmic Symphonies of Async Actions
Redux-Observable is a powerful middleware for Redux that harnesses the might of RxJS to handle complex asynchronous actions and side effects in your React applications. It allows you to compose and cancel async actions with the elegance of reactive programming, turning your data flow into a cosmic symphony of streams and observables.
Features of the Celestial Middleware
- RxJS Integration: Leverage the full power of RxJS operators in your Redux workflow.
- Epics: Use Epics to handle complex async flows and side effects.
- Cancellation: Easily cancel ongoing async operations.
- Composability: Combine multiple Epics to create more complex behaviors.
- Testability: Epics are pure functions, making them highly testable.
Installing the Cosmic Toolkit
To begin your journey with redux-observable, you’ll need to install it along with its peer dependencies. Open your terminal and run:
npm install redux-observable rxjs redux
For yarn enthusiasts:
yarn add redux-observable rxjs redux
Basic Usage: Your First Epic
Let’s start with a simple Epic that listens for a ‘PING’ action and responds with a ‘PONG’ after a brief delay.
The Ping-Pong Cosmic Dance
import { ofType } from 'redux-observable';
import { delay, mapTo } from 'rxjs/operators';
const pingEpic = (action$) => action$.pipe(
ofType('PING'),
delay(1000),
mapTo({ type: 'PONG' })
);
This Epic listens for ‘PING’ actions, waits for 1 second, and then dispatches a ‘PONG’ action. It’s a simple yet effective demonstration of how Epics can transform and delay actions.
Setting Up the Middleware
To use your Epics, you need to set up the redux-observable middleware:
import { createStore, applyMiddleware } from 'redux';
import { createEpicMiddleware } from 'redux-observable';
import { rootEpic } from './epics';
import { rootReducer } from './reducers';
const epicMiddleware = createEpicMiddleware();
const store = createStore(
rootReducer,
applyMiddleware(epicMiddleware)
);
epicMiddleware.run(rootEpic);
This setup creates the Epic middleware, applies it to the Redux store, and then runs your root Epic.
Advanced Usage: Navigating the Observable Universe
Let’s explore some more advanced concepts and patterns with redux-observable.
Fetching Data from the Cosmos
Here’s an Epic that fetches user data from an API:
import { ofType } from 'redux-observable';
import { mergeMap, map, catchError } from 'rxjs/operators';
import { ajax } from 'rxjs/ajax';
import { of } from 'rxjs';
const fetchUserEpic = (action$) => action$.pipe(
ofType('FETCH_USER'),
mergeMap(action => ajax.getJSON(`https://api.example.com/users/${action.payload}`).pipe(
map(response => ({ type: 'FETCH_USER_SUCCESS', payload: response })),
catchError(error => of({ type: 'FETCH_USER_ERROR', payload: error.message }))
))
);
This Epic listens for ‘FETCH_USER’ actions, makes an API call, and dispatches either a success or error action based on the result. The mergeMap
operator allows multiple concurrent requests, while catchError
handles any errors that occur during the API call.
Cancelling Async Operations
Sometimes you need to cancel an ongoing operation. Here’s how you can implement cancellation:
import { ofType } from 'redux-observable';
import { mergeMap, map, takeUntil } from 'rxjs/operators';
import { ajax } from 'rxjs/ajax';
const cancelableFetchUserEpic = (action$) => action$.pipe(
ofType('FETCH_USER'),
mergeMap(action => ajax.getJSON(`https://api.example.com/users/${action.payload}`).pipe(
map(response => ({ type: 'FETCH_USER_SUCCESS', payload: response })),
takeUntil(action$.pipe(ofType('CANCEL_FETCH_USER')))
))
);
This Epic will cancel the ongoing API call if a ‘CANCEL_FETCH_USER’ action is dispatched before the call completes.
Combining Epics
As your application grows, you’ll want to split your Epics into smaller, more manageable pieces. You can combine them using the combineEpics
utility:
import { combineEpics } from 'redux-observable';
const rootEpic = combineEpics(
pingEpic,
fetchUserEpic,
cancelableFetchUserEpic
);
This creates a single root Epic that you can pass to your middleware setup.
Conclusion
Redux-Observable opens up a universe of possibilities for handling complex asynchronous flows in your Redux applications. By leveraging the power of RxJS, you can create elegant, reactive patterns that make even the most intricate side effects manageable.
As you continue your journey through the cosmos of reactive programming, remember that the true power of redux-observable lies in its ability to compose complex behaviors from simple, pure functions. With Epics, you can orchestrate a symphony of actions, transforming your application’s data flow into a harmonious dance of observables.
Whether you’re building a small React application or a large-scale enterprise system, redux-observable provides the tools you need to handle async operations with grace and precision. So go forth, brave developer, and may your streams be ever flowing and your side effects always pure!