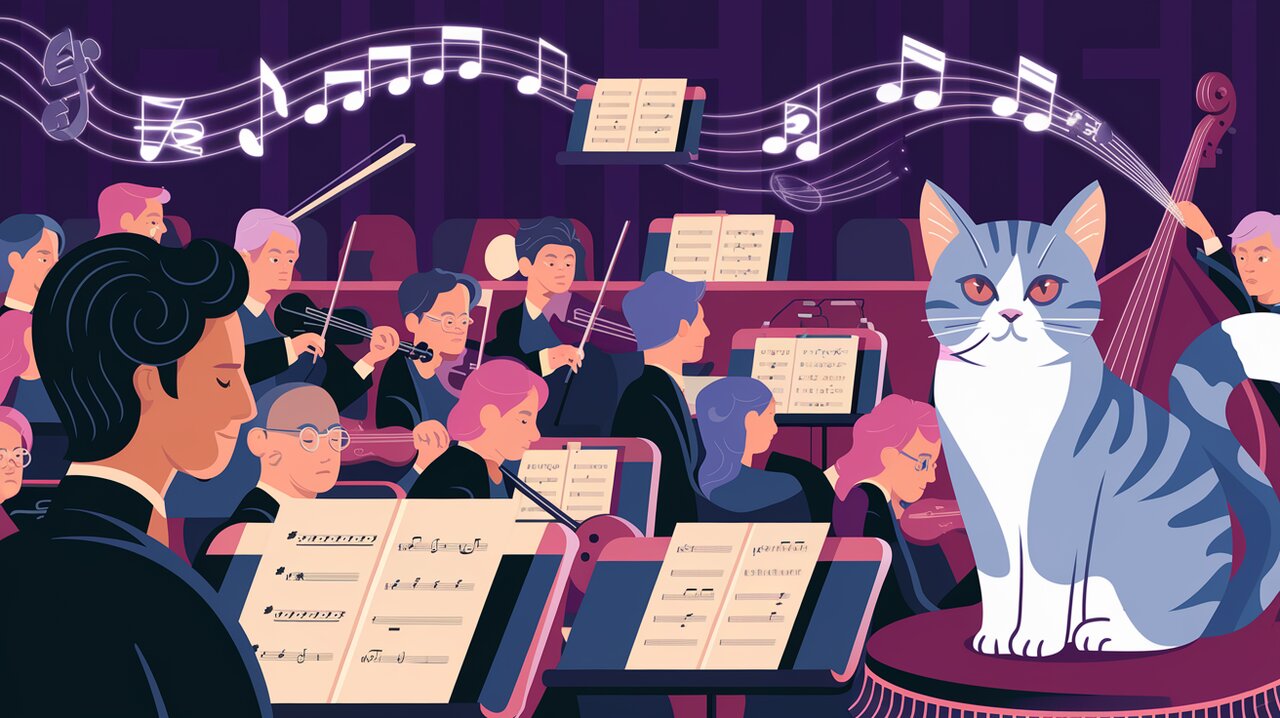
Orchestrating Redux Actions with redux-observable-middleware
Redux is a powerful state management library for JavaScript applications, but handling asynchronous actions can sometimes feel like conducting a complex symphony. Enter redux-observable-middleware, a harmonious solution that brings the elegance of observables to your Redux orchestra. This middleware allows you to subscribe to observables in your action creators, creating a more reactive and maintainable flow of data in your application.
Key Features of redux-observable-middleware
- Seamless integration with Redux and observables
- Automatic action dispatching for observable events
- Flexible action type configuration
- Support for any object with a
subscribe
method
Installing the Middleware
To start using redux-observable-middleware in your project, you’ll need to install it via npm or yarn. Here’s how you can do it:
Using npm:
npm install redux-observable-middleware
Using yarn:
yarn add redux-observable-middleware
Basic Usage: Your First Observable Action
Let’s dive into how you can use redux-observable-middleware in your Redux application. We’ll start with a basic example that demonstrates the core functionality.
Setting Up the Middleware
First, you need to apply the middleware to your Redux store:
import { createStore, applyMiddleware } from 'redux';
import observableMiddleware from 'redux-observable-middleware';
import rootReducer from './reducers';
const store = createStore(
rootReducer,
applyMiddleware(observableMiddleware)
);
This setup allows the middleware to intercept actions containing observables and handle them appropriately.
Creating an Observable Action
Now, let’s create an action that uses an observable:
import { Observable } from 'rxjs';
const INTERVAL_ACTION = 'INTERVAL';
const intervalAction = {
type: INTERVAL_ACTION,
observable: Observable.interval(1000).take(5)
};
store.dispatch(intervalAction);
In this example, we’re dispatching an action with an observable that emits a value every second, for a total of five emissions.
Handling Observable Actions in the Reducer
Your reducer needs to handle the different action types that redux-observable-middleware will dispatch:
function reducer(state = null, action) {
switch (action.type) {
case `${INTERVAL_ACTION}_ON_NEXT`:
return action.data;
case `${INTERVAL_ACTION}_ON_ERROR`:
return state;
case `${INTERVAL_ACTION}_ON_COMPLETED`:
return state;
default:
return state;
}
}
The middleware will automatically dispatch actions with _ON_NEXT
, _ON_ERROR
, and _ON_COMPLETED
suffixes, allowing you to handle different stages of the observable lifecycle.
Advanced Usage: Customizing Action Types
redux-observable-middleware offers flexibility in how you define action types for different observable events. Let’s explore some advanced usage scenarios.
Object-based Action Types
Instead of using a string for the action type, you can use an object to specify different action types for each observable event:
const customAction = {
type: {
onSubscribe: 'CUSTOM_SUBSCRIBE',
onNext: 'CUSTOM_NEXT',
onError: 'CUSTOM_ERROR',
onCompleted: 'CUSTOM_COMPLETED'
},
observable: Observable.of(1, 2, 3)
};
store.dispatch(customAction);
This approach gives you fine-grained control over the action types dispatched for each stage of the observable lifecycle.
Partial Action Type Definitions
You don’t need to specify all action types. The middleware will only dispatch actions for the types you define:
const partialAction = {
type: {
onNext: 'PARTIAL_NEXT',
onCompleted: 'PARTIAL_COMPLETED'
},
observable: Observable.of('Hello', 'World')
};
store.dispatch(partialAction);
In this case, only PARTIAL_NEXT
and PARTIAL_COMPLETED
actions will be dispatched, ignoring the subscribe and error events.
Handling Complex Asynchronous Flows
redux-observable-middleware shines when dealing with complex asynchronous flows. Let’s look at a more advanced example involving API calls and error handling.
Fetching Data with Observables
Here’s how you might use the middleware to handle a data fetching scenario:
import { Observable } from 'rxjs';
import { ajax } from 'rxjs/ajax';
import { map, catchError } from 'rxjs/operators';
const fetchUserAction = {
type: 'FETCH_USER',
observable: ajax.getJSON('https://api.example.com/user').pipe(
map(response => ({ type: 'FETCH_USER_SUCCESS', payload: response })),
catchError(error => Observable.of({ type: 'FETCH_USER_ERROR', error }))
)
};
store.dispatch(fetchUserAction);
This action uses RxJS’s ajax
utility to make an HTTP request. The map
operator transforms the successful response into a success action, while catchError
handles any errors by emitting an error action.
Combining Multiple Observables
You can also combine multiple observables to create more complex workflows:
import { Observable } from 'rxjs';
import { mergeMap } from 'rxjs/operators';
const complexAction = {
type: 'COMPLEX_OPERATION',
observable: Observable.of(1, 2, 3).pipe(
mergeMap(value =>
Observable.timer(1000).map(() => ({ type: 'STEP_COMPLETED', value }))
)
)
};
store.dispatch(complexAction);
This example demonstrates how you can chain observables to create a sequence of delayed actions, each emitting a ‘STEP_COMPLETED’ action after a one-second delay.
Conclusion: Harmonizing Your Redux Symphony
redux-observable-middleware provides a powerful way to integrate observables into your Redux workflow. By allowing you to handle asynchronous actions with the elegance and flexibility of observables, it helps you create more reactive and maintainable applications.
Whether you’re dealing with simple intervals, complex API calls, or intricate asynchronous workflows, this middleware offers the tools you need to orchestrate your Redux actions beautifully. As you become more familiar with its capabilities, you’ll find that it opens up new possibilities for managing state and side effects in your React applications.
Remember, like any powerful tool, it’s important to use redux-observable-middleware judiciously. Start with simple use cases and gradually incorporate more complex patterns as you become comfortable with the library. Happy coding, and may your Redux symphony always be in perfect harmony!