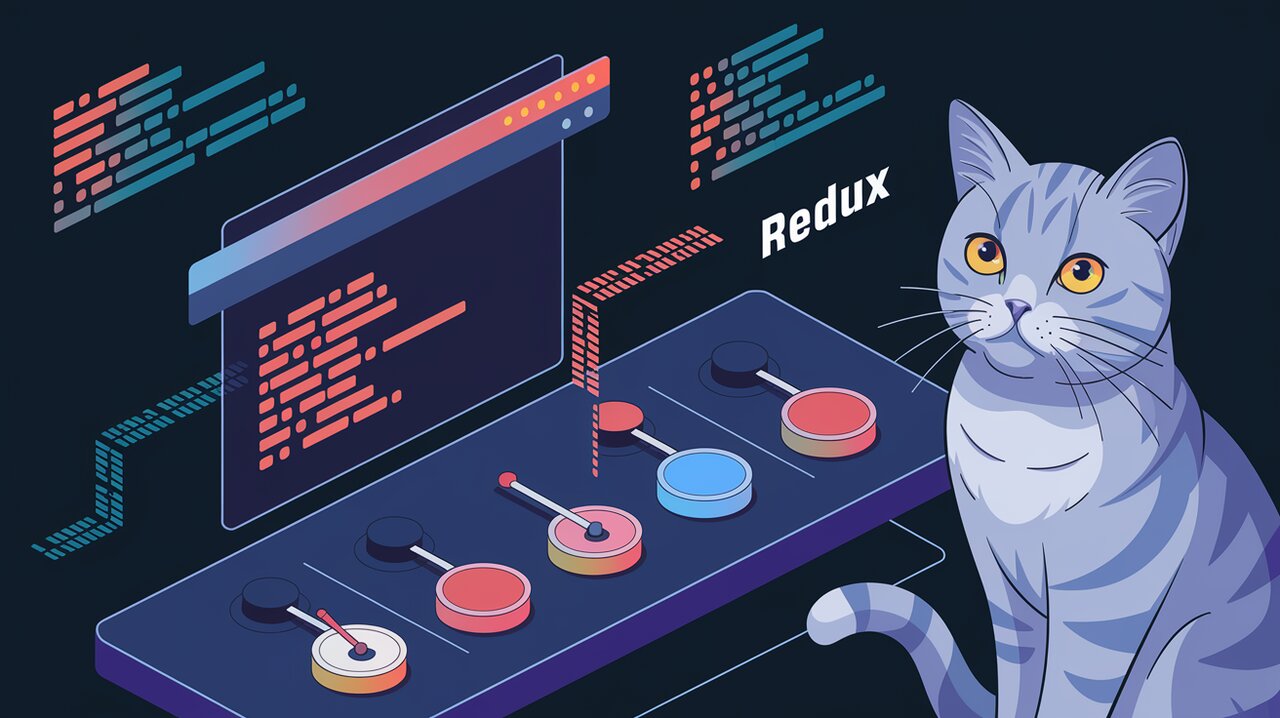
Redux Query Sync: Orchestrating URL and State Harmony
Redux Query Sync is a powerful library that brings harmony to your React applications by synchronizing your Redux state with URL query parameters. This seamless integration allows for creating dynamic, shareable URLs that reflect your application’s current state, enhancing user experience and simplifying state management.
Bridging Redux and URL Parameters
At its core, redux-query-sync treats URL query parameters as exposed variables of your Redux state. This means that changes in your store state are automatically reflected in the URL, and vice versa. For instance, a URL like /mypage.html?p=14
could correspond to a state object containing {pageNumber: 14}
.
Key Features
- Bidirectional Synchronization: Automatically updates URL when state changes and updates state when URL changes.
- Customizable Mapping: Allows defining custom logic for converting between state values and URL parameter strings.
- History Integration: Works with the
history
module, ensuring compatibility with popular routing solutions. - Selective Synchronization: Synchronize only the state properties you choose, keeping your URLs clean and relevant.
Getting Started
Let’s dive into how you can integrate redux-query-sync into your React application.
Installation
First, install the package using npm:
npm install redux-query-sync
Basic Usage
Here’s a simple example of how to use redux-query-sync:
import ReduxQuerySync from 'redux-query-sync';
ReduxQuerySync({
store: yourReduxStore,
params: {
dest: {
selector: state => state.route.destination,
action: value => ({ type: 'setDestination', payload: value }),
},
},
initialTruth: 'location',
});
In this example, we’re synchronizing the dest
query parameter with the route.destination
field in our Redux state.
Advanced Configuration
For more complex scenarios, redux-query-sync offers advanced configuration options:
ReduxQuerySync({
store: yourReduxStore,
params: {
p: {
selector: state => state.pageNumber,
action: value => ({ type: 'setPageNumber', payload: value }),
stringToValue: string => Number.parseInt(string) || 1,
valueToString: value => `${value}`,
defaultValue: 1,
},
},
initialTruth: 'location',
replaceState: true,
});
This configuration adds type conversion, default values, and uses replaceState
for smoother browser history management.
Practical Applications
Redux Query Sync shines in scenarios where you want to create shareable application states. Some common use cases include:
- Filtered Lists: Synchronize filter parameters with the URL for easy sharing of specific views.
- Pagination: Keep the current page number in the URL for bookmarkable paginated content.
- Search Results: Reflect search queries and options in the URL for shareable search results.
Integration with React Router
While redux-query-sync works great on its own, it can also be used alongside React Router. When using both, ensure you use the Router
component (not BrowserRouter
) and pass the same history
instance to both libraries.
import { createBrowserHistory } from 'history';
import { Router } from 'react-router-dom';
const history = createBrowserHistory();
// Pass history to ReduxQuerySync
ReduxQuerySync({
store: yourReduxStore,
params: { /* your params */ },
history,
});
// Use the same history with Router
ReactDOM.render(
<Router history={history}>
<App />
</Router>,
document.getElementById('root')
);
Enhancer Approach
For those who prefer a more declarative setup, redux-query-sync offers an enhancer that can be used when creating your Redux store:
import { createStore } from 'redux';
import ReduxQuerySync from 'redux-query-sync';
const storeEnhancer = ReduxQuerySync.enhancer({
params: { /* your params */ },
initialTruth: 'location',
});
const store = createStore(rootReducer, initialState, storeEnhancer);
This approach integrates redux-query-sync directly into your store creation process, providing a clean and centralized configuration.
Conclusion
Redux Query Sync offers a elegant solution for keeping your application’s state in sync with the URL. By leveraging this library, you can create more user-friendly, shareable, and bookmarkable React applications. Whether you’re building a complex dashboard, an e-commerce platform, or any application where state persistence in the URL is beneficial, redux-query-sync provides the tools to achieve this with minimal overhead.
As you explore the possibilities of URL state synchronization, you might also be interested in other state management solutions. Check out our articles on Zustand: Simplifying React State Management for a lightweight alternative to Redux, or Jotai: Primitive and flexible state management for React for an atom-based approach to state management.
Remember, the key to effective state management is choosing the right tool for your specific needs. Redux Query Sync offers a powerful solution for URL-state synchronization, but always consider your project’s requirements and complexity when selecting your state management strategy.