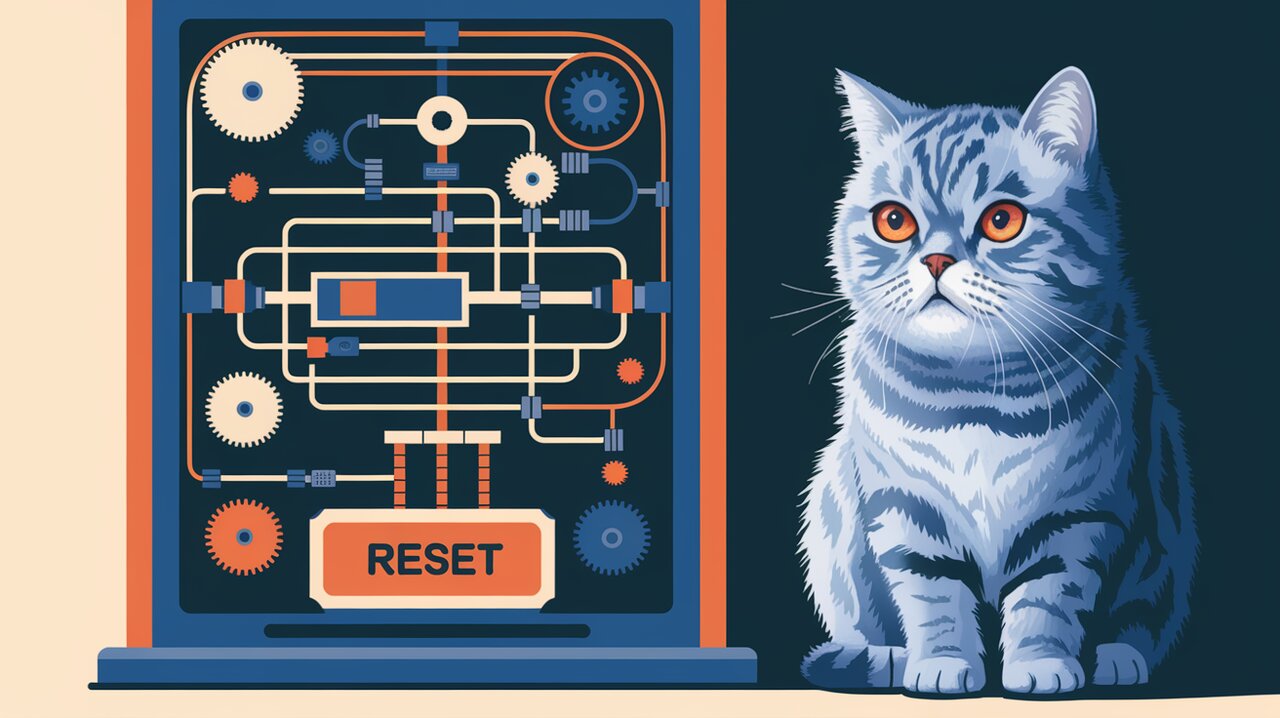
In the world of React and Redux, managing state can sometimes feel like juggling while riding a unicycle. Enter Redux-Recycle, a nifty little library that acts as your state management safety net. This powerful tool allows you to reset your Redux state on specific actions, giving you more control and flexibility in your applications.
Unveiling the Magic of Redux-Recycle
Redux-Recycle is a higher-order reducer that enhances your existing Redux setup. It provides a recycleState
function that wraps around your reducer, allowing you to specify actions that will trigger a state reset. This can be incredibly useful in scenarios such as user logout, where you want to clear all user-related data, or when implementing a “reset to defaults” feature in your application.
Key Features
- Selective State Reset: Reset state based on specific actions.
- Flexible Initial State: Use a static value or a function to determine the reset state.
- Custom Reset Action: Optionally specify a custom action type for the reset.
- TypeScript Support: Fully typed for use in TypeScript projects.
Setting Up Your Redux-Recycle Spell Book
Before we dive into the magical world of state recycling, let’s get our environment set up. You can install Redux-Recycle using npm or yarn:
npm install --save redux-recycle
Or if you prefer yarn:
yarn add redux-recycle
Basic Incantations: Getting Started with Redux-Recycle
Let’s start with some basic usage of Redux-Recycle. We’ll create a simple counter reducer and enhance it with the ability to reset.
Creating a Recyclable Counter
First, let’s import the necessary functions and create our counter reducer:
import { createSlice, PayloadAction } from '@reduxjs/toolkit';
import recycleState from 'redux-recycle';
const counterSlice = createSlice({
name: 'counter',
initialState: 0,
reducers: {
increment: (state) => state + 1,
decrement: (state) => state - 1,
},
});
const { increment, decrement } = counterSlice.actions;
Now, let’s enhance our reducer with Redux-Recycle:
const RESET_ACTION = 'counter/reset';
const recyclableCounterReducer = recycleState(
counterSlice.reducer,
[RESET_ACTION],
0
);
In this example, we’ve wrapped our counter reducer with recycleState
. The second argument is an array of action types that will trigger a reset, and the third argument is the initial state to reset to.
Using Our Recyclable Counter
Now that we have our recyclable counter, let’s see how we can use it in our Redux store:
import { configureStore } from '@reduxjs/toolkit';
const store = configureStore({
reducer: {
counter: recyclableCounterReducer,
},
});
// Usage
store.dispatch(increment()); // Counter: 1
store.dispatch(increment()); // Counter: 2
store.dispatch({ type: RESET_ACTION }); // Counter: 0
This simple example demonstrates how Redux-Recycle allows us to easily reset our state to its initial value when a specific action is dispatched.
Advanced Spells: Mastering Redux-Recycle
Now that we’ve covered the basics, let’s explore some more advanced uses of Redux-Recycle.
Dynamic Initial State
Sometimes, you might want to reset your state to a value that depends on the current state or the action that triggered the reset. Redux-Recycle allows you to pass a function as the initial state:
const dynamicRecyclableCounterReducer = recycleState(
counterSlice.reducer,
[RESET_ACTION],
(state, action) => {
if (action.payload && action.payload.resetTo) {
return action.payload.resetTo;
}
return state > 10 ? 10 : 0;
}
);
In this example, we’re using a function to determine the reset state. If the reset action includes a resetTo
value in its payload, we use that. Otherwise, we cap the reset value at 10 if the current state is higher than 10.
Custom Reset Action Type
By default, Redux-Recycle uses a generic action type when resetting the state. However, you can specify a custom action type:
const CUSTOM_RESET = 'counter/customReset';
const customResetCounterReducer = recycleState(
counterSlice.reducer,
[RESET_ACTION],
0,
{ recycleActionType: CUSTOM_RESET }
);
This can be useful if you want to trigger additional side effects when the state is reset.
Multiple Reset Triggers
You’re not limited to a single reset action. You can specify multiple actions that will trigger a reset:
const multiResetCounterReducer = recycleState(
counterSlice.reducer,
['counter/reset', 'user/logout', 'app/clearAll'],
0
);
This is particularly useful in larger applications where you might want to reset certain parts of your state in response to various events.
Wrapping Up Our Redux-Recycle Spell Book
Redux-Recycle provides a powerful and flexible way to manage state resets in your Redux applications. By allowing you to specify reset actions and customize the reset behavior, it can significantly simplify your state management logic.
Remember, with great power comes great responsibility. While Redux-Recycle makes it easy to reset your state, it’s important to use it judiciously. Overusing state resets can lead to unexpected behavior and make your application harder to reason about.
As you continue your journey with React and Redux, keep Redux-Recycle in your toolkit. It’s a magical little library that can save you from many state management headaches. Happy coding, and may your states always reset smoothly!