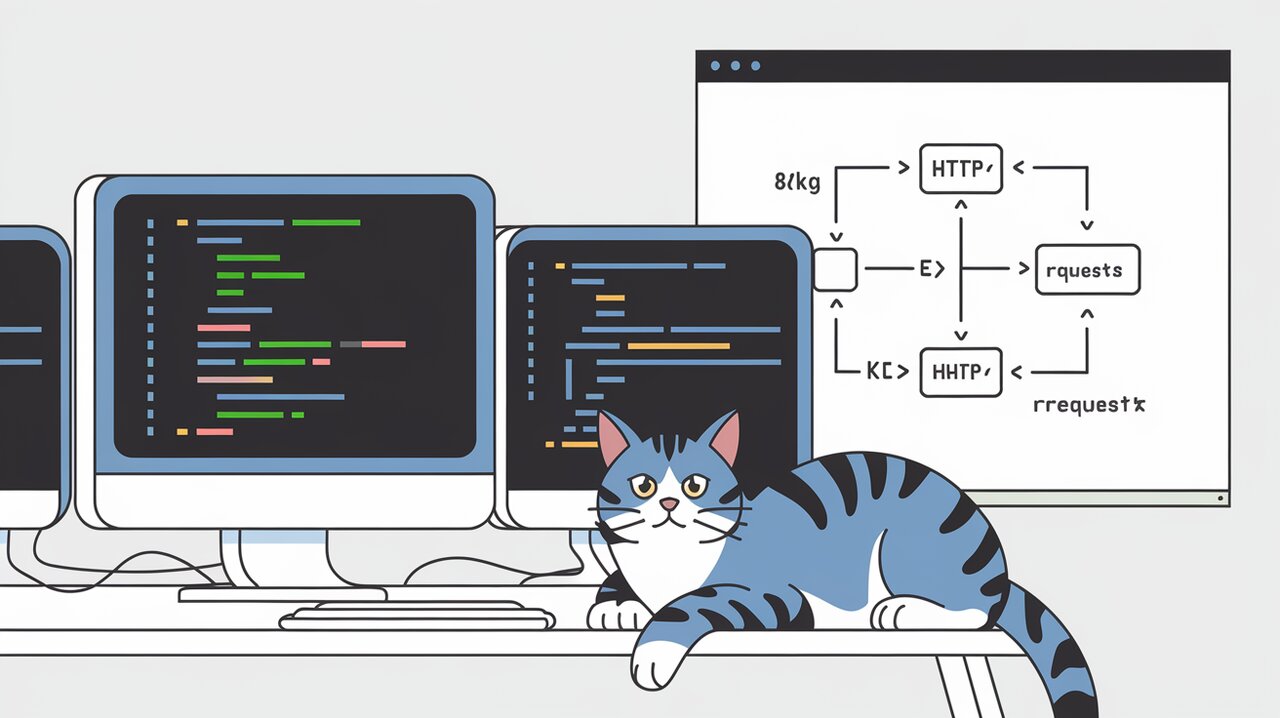
Redux-Requests: Taming the Async Beast in Your React Apps
Redux has long been a go-to solution for state management in React applications. However, when it comes to handling asynchronous operations, especially HTTP requests, things can get complicated quickly. Enter redux-requests, a library designed to simplify this process and make your Redux-powered React applications more efficient.
The Problem with Duplicate Requests
Imagine you have two components in your React application that need the same data from an API. Without proper management, you might end up making two identical HTTP requests when rendering these components. This not only wastes bandwidth but can also lead to unnecessary updates to your Redux store.
Redux-requests solves this problem elegantly by keeping track of pending requests and preventing duplicate API calls. Let’s explore how this library can transform your async logic.
Key Features of redux-requests
- Automatic Request Deduplication: Prevents multiple identical requests from being sent simultaneously.
- Simple Integration: Works seamlessly with existing Redux setups.
- Flexible Configuration: Allows customization to fit your specific use cases.
- Improved Performance: Reduces unnecessary network traffic and state updates.
Getting Started with redux-requests
First, let’s install the library:
npm install --save redux-requests
Now, let’s see how to integrate redux-requests into your Redux setup:
import { createStore, applyMiddleware, combineReducers } from 'redux';
import { requestsReducer, createRequestMiddleware } from 'redux-requests';
import thunkMiddleware from 'redux-thunk';
const rootReducer = combineReducers({
requests: requestsReducer,
// ... your other reducers
});
const store = createStore(
rootReducer,
applyMiddleware(thunkMiddleware, createRequestMiddleware())
);
In this setup, we’ve added the requestsReducer
to our root reducer and included the createRequestMiddleware
in our middleware chain.
Making Requests with redux-requests
Now, let’s look at how to use redux-requests in your action creators:
import { attemptRequest } from 'redux-requests';
export function fetchUserData(userId: string) {
return (dispatch) => {
const url = `https://api.example.com/users/${userId}`;
attemptRequest(
url,
{
begin: () => ({ type: 'FETCH_USER_BEGIN', payload: { userId } }),
success: (response) => ({ type: 'FETCH_USER_SUCCESS', payload: { userId, data: response } }),
failure: (error) => ({ type: 'FETCH_USER_FAILURE', payload: { userId, error } })
},
() => fetch(url).then(response => response.json()),
dispatch
);
};
}
In this example, attemptRequest
manages the entire request lifecycle. It dispatches the appropriate actions based on the request’s state and ensures that duplicate requests are not made.
Advanced Usage: Custom Middleware Configuration
For more complex scenarios, you can customize the middleware:
const customMiddleware = createRequestMiddleware({
getRequestState: state => state.customRequestsSlice,
shouldMakeRequest: (state, url) => {
// Custom logic to determine if a request should be made
return !state.customRequestsSlice[url];
}
});
This configuration allows you to specify where the request state is stored and add custom logic for determining when requests should be made.
Conclusion
Redux-requests is a powerful tool for managing asynchronous operations in Redux applications. By preventing duplicate requests and simplifying the management of in-flight HTTP calls, it helps you write cleaner, more efficient code.
As you continue to explore state management in React, you might also be interested in learning about other Redux-related libraries. Check out our articles on redux-future for handling async actions, or redux-bees for another approach to API interactions in Redux.
By incorporating redux-requests into your toolkit, you’re taking a significant step towards taming the async beast in your React applications. Happy coding!