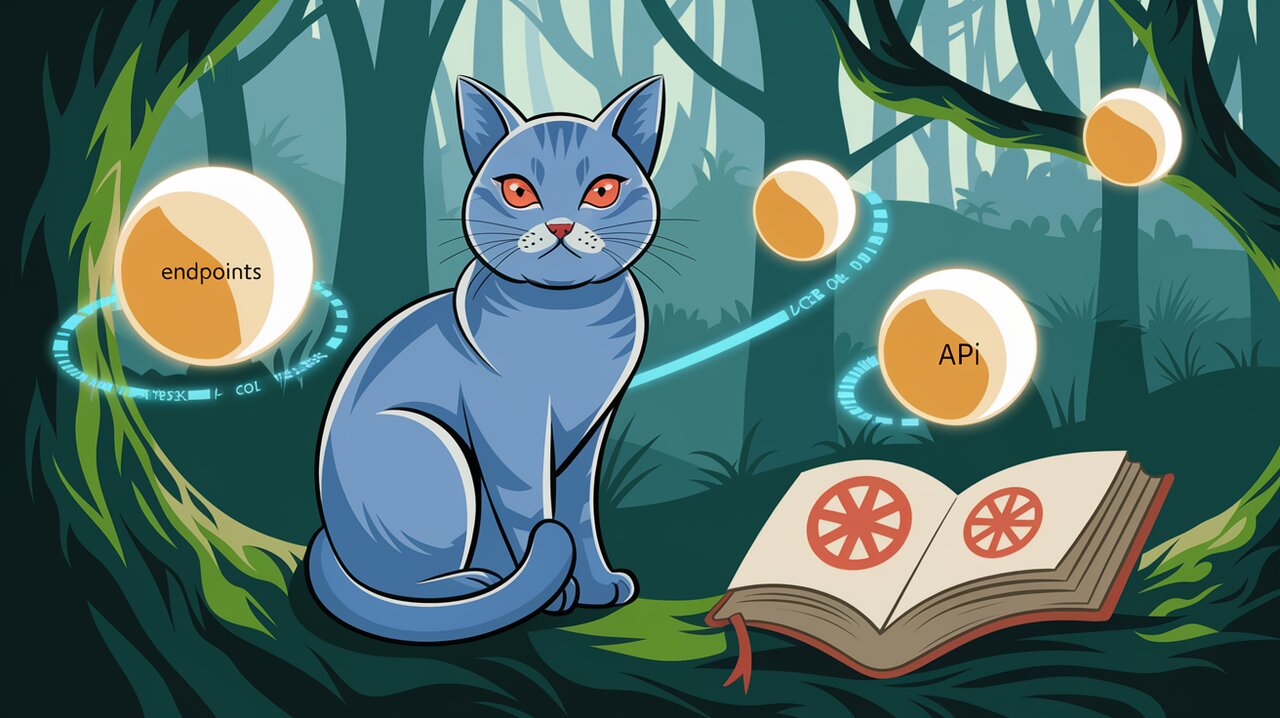
Conjuring REST APIs with Redux-Rest: A Magical Journey
Redux-Rest is a powerful enchantment that simplifies the integration of REST APIs into your Redux applications. With its magical abilities, you can banish the boilerplate code that often plagues API management and focus on crafting truly remarkable user experiences. Let’s embark on a journey through this mystical library and discover how it can transform your Redux workflow.
Summoning the Redux-Rest Spell
To begin our magical adventure, we must first summon Redux-Rest into our project. Open your cauldron (or terminal) and chant the following incantation:
npm install redux-rest
With the spell components in place, we’re ready to weave our first enchantment.
Crafting Your API Grimoire
The first step in mastering Redux-Rest is to create a grimoire of your API endpoints. This is done by describing your API as a simple object:
const myAPI = {
users: '/api/users/',
posts: '/api/posts/',
comments: '/api/comments/'
};
This grimoire serves as a map for Redux-Rest, guiding its magic to the correct endpoints of your API realm.
Conjuring the API Instance
With our grimoire prepared, we can now conjure the main artifact of our spell - the API instance:
import API from 'redux-rest';
const api = new API(myAPI);
This simple incantation creates a powerful artifact that automatically generates action creators and reducers for each endpoint in your grimoire. No more tedious scribing of repetitive code!
Wielding Action Creators
Redux-Rest bestows upon you a set of action creators for each API endpoint. These magical functions allow you to interact with your API with ease:
// Fetch all users
api.actionCreators.users.list();
// Create a new user
api.actionCreators.users.create({ username: 'Merlin', email: 'merlin@camelot.com' });
// Update a user
api.actionCreators.users.update(1, { username: 'Arthur' });
// Retrieve a specific user
api.actionCreators.users.retrieve(1);
Each of these spells dispatches the appropriate action and handles the API request, allowing you to focus on the higher-level magic of your application.
The Alchemy of Reducers
Redux-Rest doesn’t stop at action creators. It also conjures reducers to manage the state of your API data:
import { combineReducers } from 'redux';
const rootReducer = combineReducers(api.reducers);
These reducers manage the state for each endpoint, handling actions like ‘pending’, ‘success’, and ‘failure’ for your API requests. They even manage optimistic updates, allowing your UI to respond instantly to user actions while the API request is still in flight.
Scrying the State
With Redux-Rest’s reducers in place, you can easily peer into the state of your API data:
function UserList({ users }) {
return (
<ul>
{users.map(user => (
<li key={user.id}>{user.username}</li>
))}
</ul>
);
}
function mapStateToProps(state) {
return {
users: state.users_items
};
}
export default connect(mapStateToProps)(UserList);
The state is structured intuitively, with _items
suffixes for collections and _item
for individual resources.
Advanced Incantations
Redux-Rest offers more advanced magic for those willing to delve deeper. You can customize the behavior of your API calls, handle authentication, and even extend the library to fit your specific needs.
For example, you can add custom headers to your API requests:
const api = new API(myAPI, {
headers: {
'Authorization': 'Bearer your-token-here'
}
});
Conclusion
Redux-Rest is a powerful artifact in the Redux ecosystem, capable of greatly simplifying your API integration. By automating the creation of action creators and reducers, it allows you to focus on the unique aspects of your application rather than the repetitive incantations of API management.
As you continue your journey through the realms of Redux, remember that Redux-Rest is but one tool in your magical arsenal. Consider exploring other enchanted libraries like Redux-Saga for more complex asynchronous workflows, or Redux-Observable for reactive programming patterns.
May your code be bug-free and your APIs responsive as you wield the power of Redux-Rest in your applications!