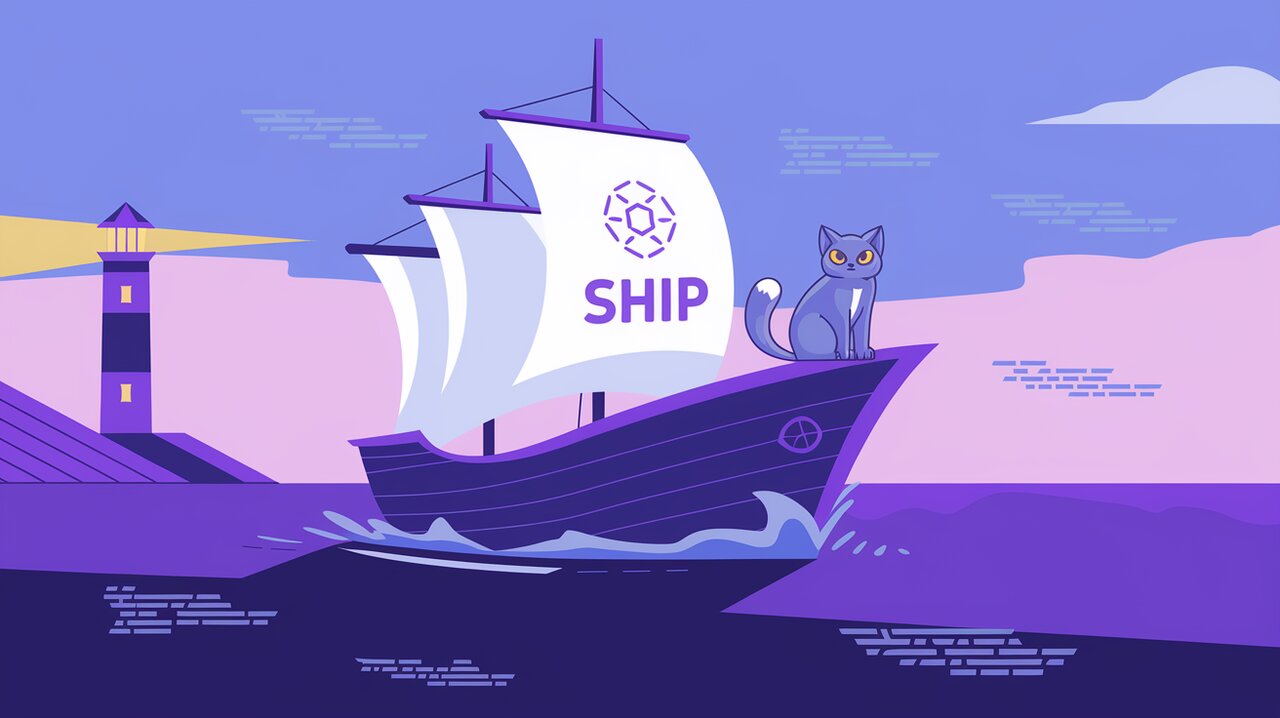
Redux Ship: Sailing the Smooth Seas of Side Effects
In the vast ocean of state management, Redux Ship emerges as a beacon of hope for developers navigating the treacherous waters of side effects. This powerful library, designed to work seamlessly with Redux, offers a unique approach to handling side effects that promises to make your development journey smoother and more enjoyable.
Charting the Course: Key Features of Redux Ship
Redux Ship brings several innovative features to the table, making it a valuable addition to any Redux developer’s toolkit:
- Snapshot System: Built-in snapshots allow for live debugging and simplified unit testing.
- Serialized Side Effects: Using generators, Redux Ship serializes all side effects, including the control flow of your code.
- Free Monads Approach: Leverages the concept of free monads for a more functional and composable side effect handling.
- Composition Mechanism: Provides a way to compose sub-stores with effects, enhancing modularity.
- Full Typing Support: Offers comprehensive typing support (currently for Flow), improving code reliability.
Setting Sail: Installation and Setup
To embark on your journey with Redux Ship, you’ll first need to install it in your project. You can do this using npm or yarn:
npm install --save redux-ship
or
yarn add redux-ship
Once installed, you’re ready to integrate Redux Ship into your Redux application.
Navigating the Basics: Getting Started with Redux Ship
Defining Side Effects
Let’s start by looking at how to define side effects using Redux Ship. Here’s a simple example that fetches movies for R2D2 from the Star Wars API:
import * as Ship from 'redux-ship';
import * as Effect from './effects';
function* fetchR2D2Movies() {
// Check if we already have the movies in the Redux store
const currentMovies = yield* Ship.getState(state => state.movies);
if (!currentMovies) {
// Notify Redux that we're starting to load movies
yield* Ship.commit({ type: 'LOAD_START' });
// Fetch R2D2's data
const r2d2 = yield* Effect.httpRequest('https://swapi.co/api/people/3/');
// Fetch each movie
const movies = yield* Ship.all(JSON.parse(r2d2).films.map(function* (movieUrl) {
const movie = yield* Effect.httpRequest(movieUrl);
return JSON.parse(movie).title;
}));
// Update the Redux store with the fetched movies
yield* Ship.commit({ type: 'LOAD_SUCCESS', movies });
}
}
In this example, we use Redux Ship’s getState
, commit
, and all
functions to handle our side effects. The yield*
syntax is used to delegate to other generator functions, allowing for a clean and readable flow of asynchronous operations.
Explaining the Code
Let’s break down what’s happening in this code:
- We first check if we already have the movies in our Redux store using
Ship.getState
. - If we don’t have the movies, we dispatch a
LOAD_START
action usingShip.commit
. - We then make an HTTP request to fetch R2D2’s data using a custom
Effect.httpRequest
function. - For each movie URL in R2D2’s data, we make another HTTP request to fetch the movie details.
- Finally, we dispatch a
LOAD_SUCCESS
action with the fetched movies usingShip.commit
.
This approach allows us to handle complex asynchronous flows in a synchronous-looking manner, making our code easier to reason about and debug.
Charting Advanced Waters: Snapshots and Debugging
One of Redux Ship’s most powerful features is its snapshot system. This system allows you to capture a detailed view of your side effects as they occur, making debugging a breeze.
Capturing Snapshots
When you run your side effect function with Redux Ship’s devtools, you get a serialized snapshot of the execution. Here’s what a snapshot might look like for our R2D2 movies example:
[
{
"type": "GetState",
"state": null
},
{
"type": "Commit",
"commit": {
"type": "LOAD_START"
}
},
{
"type": "Effect",
"effect": {
"type": "HttpRequest",
"url": "https://swapi.co/api/people/3/"
},
"result": "{ \"name\": \"R2-D2\", ... }"
},
{
"type": "All",
"snapshots": [
[
{
"type": "Effect",
"effect": {
"type": "HttpRequest",
"url": "https://swapi.co/api/films/2/"
},
"result": "{ \"title\": \"The Empire Strikes Back\", ... }"
}
],
// ... more movie requests
]
},
{
"type": "Commit",
"commit": {
"type": "LOAD_SUCCESS",
"movies": [
"The Empire Strikes Back",
// ... more movie titles
]
}
}
]
This snapshot provides a detailed view of every step in your side effect execution, including state accesses, Redux actions, and external effects like API calls.
Leveraging Snapshots for Debugging
With these snapshots, you can easily trace the flow of your side effects and identify where issues might be occurring. The Redux Ship devtools provide a visual interface for exploring these snapshots, allowing you to step through each action and inspect the state at each point.
Sailing into the Sunset: Conclusion
Redux Ship offers a powerful and intuitive way to handle side effects in your Redux applications. By providing a serializable representation of your side effects, it simplifies debugging and testing, two of the most challenging aspects of managing complex state and asynchronous operations.
With its snapshot system, composition mechanisms, and TypeScript support, Redux Ship equips you with the tools you need to navigate the often turbulent waters of state management and side effects. Whether you’re building a small application or a large-scale enterprise system, Redux Ship can help you chart a course to cleaner, more maintainable code.
As you continue your journey with Redux Ship, remember that mastering side effects is key to building robust and scalable applications. So hoist the sails, chart your course, and set off on your adventure with Redux Ship – smooth sailing awaits!