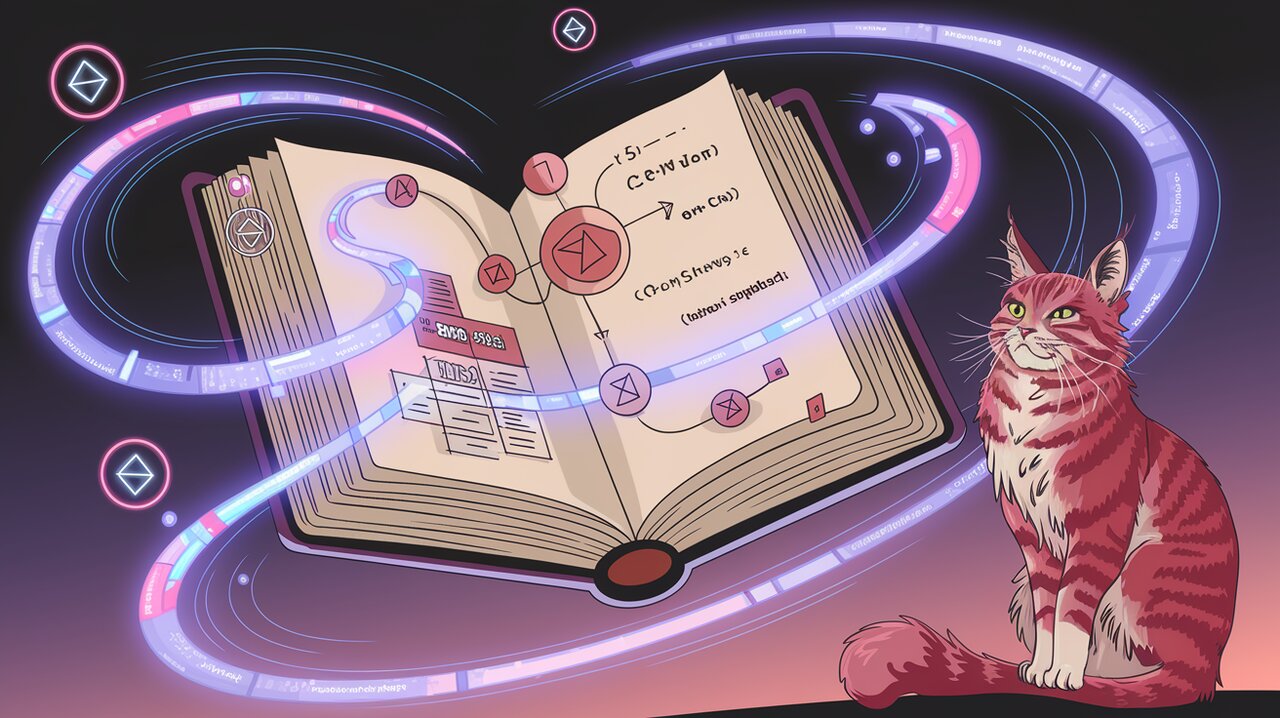
Redux Simple Promise: Conjuring Async Action Magic
Redux Simple Promise is a powerful middleware that brings the magic of simplified asynchronous action handling to your Redux-powered React applications. By leveraging the Flux Standard Action (FSA) pattern, this library allows developers to effortlessly manage promises and optimize state management with minimal boilerplate. Let’s dive into the enchanting world of redux-simple-promise
and discover how it can transform your async workflows.
Summoning the Power of Redux Simple Promise
To begin our journey into the realm of asynchronous action sorcery, we first need to install the redux-simple-promise
library. Open your terminal and cast the following spell:
npm install --save redux-simple-promise
For those who prefer the mystical arts of Yarn, you can use this incantation instead:
yarn add redux-simple-promise
Configuring the Middleware
Once you’ve acquired the power of Redux Simple Promise, it’s time to integrate it into your Redux store. Import the middleware and include it in your store configuration:
import { createStore, applyMiddleware } from 'redux';
import promiseMiddleware from 'redux-simple-promise';
import rootReducer from './reducers';
const store = createStore(
rootReducer,
applyMiddleware(promiseMiddleware())
);
Notice that we call promiseMiddleware
as a function. This allows for custom configuration, which we’ll explore later in our magical journey.
Casting Async Action Spells
With Redux Simple Promise in place, you can now create action creators that harness the power of promises. Here’s an example of how to craft such an enchantment:
function fetchUserData(userId: string) {
return {
type: 'FETCH_USER_DATA',
payload: {
promise: api.fetchUser(userId),
userId
}
};
}
In this spell, we include a promise
property within the payload
. The middleware will recognize this and work its magic, dispatching actions at different stages of the promise lifecycle.
The Lifecycle of an Async Action
When you dispatch an action with a promise, Redux Simple Promise performs a series of enchantments:
- It immediately dispatches a pending action with the original type.
- If the promise resolves, it dispatches a resolved action.
- If the promise rejects, it dispatches a rejected action.
Let’s see how this translates to actual dispatched actions:
// When dispatched
dispatch(fetchUserData('123'));
// Immediately dispatched
{ type: 'FETCH_USER_DATA', payload: { userId: '123' } }
// If promise resolves
{
type: 'FETCH_USER_DATA_RESOLVED',
payload: { id: '123', name: 'Alice' },
meta: { userId: '123' }
}
// If promise rejects
{
type: 'FETCH_USER_DATA_REJECTED',
payload: Error,
meta: { userId: '123' }
}
Crafting Reducers for Async Actions
To handle these magical actions in your reducers, Redux Simple Promise provides utility functions that make your code more readable and maintainable:
import { resolve, reject } from 'redux-simple-promise';
function userReducer(state = {}, action) {
switch (action.type) {
case 'FETCH_USER_DATA':
return { ...state, loading: true };
case resolve('FETCH_USER_DATA'):
return { ...state, ...action.payload, loading: false };
case reject('FETCH_USER_DATA'):
return { ...state, error: action.payload, loading: false };
default:
return state;
}
}
The resolve
and reject
functions allow you to easily match the resolved and rejected action types without hardcoding the suffixes.
Advanced Incantations
Custom Suffixes
You can customize the suffixes used for resolved and rejected actions when initializing the middleware:
const customPromiseMiddleware = promiseMiddleware('_SUCCESS', '_FAILURE');
Now, resolved actions will end with _SUCCESS
and rejected actions with _FAILURE
.
Optimistic Updates
Redux Simple Promise allows for optimistic updates by including additional data in the action payload:
function updateUserName(userId: string, newName: string) {
return {
type: 'UPDATE_USER_NAME',
payload: {
promise: api.updateUserName(userId, newName),
optimisticData: { name: newName }
}
};
}
The optimisticData
will be included in the initial pending action, allowing you to update the UI immediately before the API call completes.
Synergy with Other Redux Libraries
Redux Simple Promise works harmoniously with other Redux enchantments. For instance, you can combine it with redux-thunk for more complex async flows or use it alongside redux-persist to maintain state across sessions.
Conclusion
Redux Simple Promise brings a touch of magic to asynchronous action handling in Redux. By simplifying the process of working with promises, it allows developers to focus on crafting powerful and responsive React applications. Whether you’re battling complex API interactions or seeking to optimize your state management, this middleware proves to be a valuable ally in your Redux arsenal.
As you continue your journey through the mystical lands of React and Redux, remember that Redux Simple Promise is but one of many powerful tools at your disposal. Explore other enchantments like redux-observable for reactive programming or redux-saga for more advanced async flow control.
May your async actions be ever resolving and your state management eternally optimized!