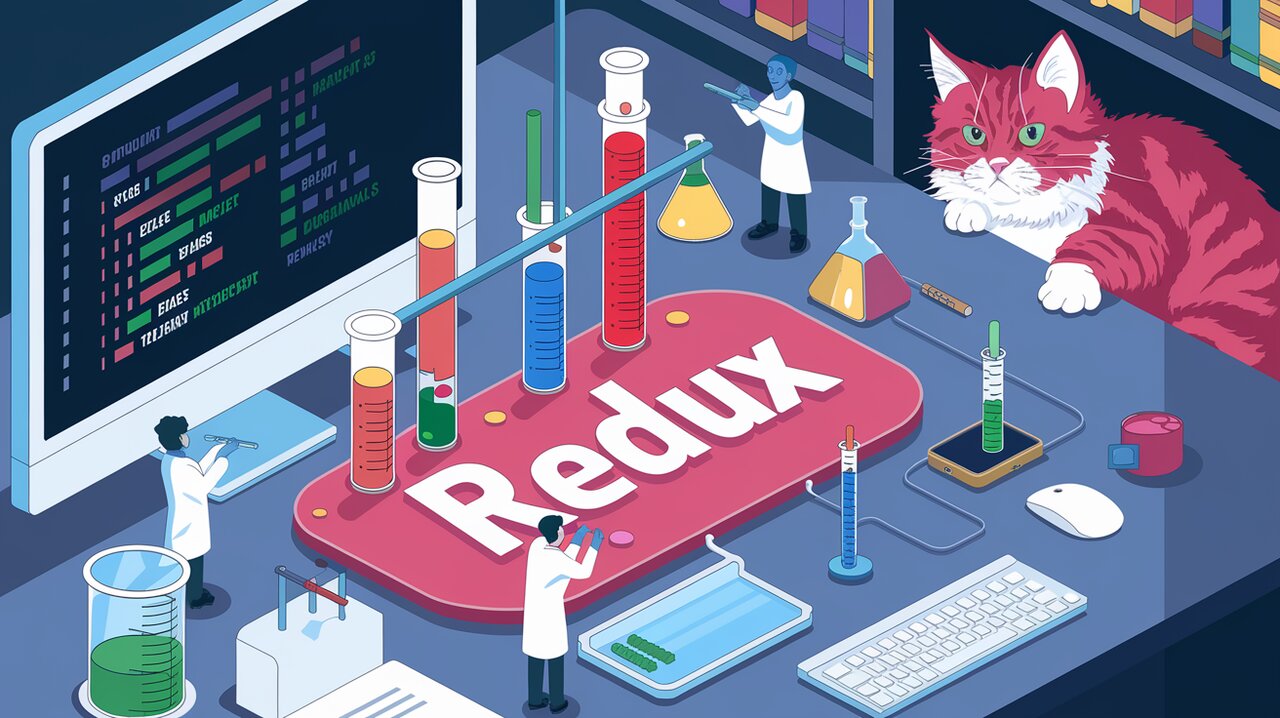
Tame Your Redux Tests with redux-test-reducer: A Developer's Best Friend
In the ever-evolving world of React development, Redux remains a popular choice for state management. However, testing Redux reducers can often feel like a daunting task, requiring verbose setups and repetitive code. Enter redux-test-reducer, a lightweight library designed to simplify and streamline the process of testing Redux reducers. This powerful tool allows developers to write cleaner, more maintainable tests with less boilerplate, ultimately leading to more robust and reliable applications.
Key Features of redux-test-reducer
redux-test-reducer comes packed with several features that make Redux testing a breeze:
- Simplified Test Creation: Write tests with minimal setup, focusing on the logic rather than boilerplate.
- Type Safety: Full TypeScript support ensures type consistency throughout your tests.
- Chainable API: Fluent interface allows for intuitive and readable test compositions.
- State Snapshots: Easily create and verify state snapshots at any point in your test chain.
- Custom Assertions: Extend the library with your own assertion functions for tailored testing needs.
Getting Started with redux-test-reducer
Installation
To begin using redux-test-reducer in your project, you can install it via npm or yarn:
npm install --save-dev redux-test-reducer
# or
yarn add --dev redux-test-reducer
Setting Up Your First Test
Let’s dive into how you can use redux-test-reducer to test a simple counter reducer. First, we’ll define our reducer:
import { createSlice, PayloadAction } from '@reduxjs/toolkit';
interface CounterState {
value: number;
}
const initialState: CounterState = {
value: 0,
};
const counterSlice = createSlice({
name: 'counter',
initialState,
reducers: {
increment: (state) => {
state.value += 1;
},
decrement: (state) => {
state.value -= 1;
},
incrementByAmount: (state, action: PayloadAction<number>) => {
state.value += action.payload;
},
},
});
export const { increment, decrement, incrementByAmount } = counterSlice.actions;
export default counterSlice.reducer;
Now, let’s write a test for this reducer using redux-test-reducer:
import { createReducerTests } from 'redux-test-reducer';
import counterReducer, { increment, decrement, incrementByAmount } from './counterSlice';
describe('counter reducer', () => {
const test = createReducerTests(counterReducer);
it('should handle initial state', () => {
test.expectInitialState({ value: 0 });
});
it('should handle increment', () => {
test
.givenInitialState({ value: 0 })
.whenActionIsDispatched(increment())
.thenStateShouldEqual({ value: 1 });
});
it('should handle decrement', () => {
test
.givenInitialState({ value: 2 })
.whenActionIsDispatched(decrement())
.thenStateShouldEqual({ value: 1 });
});
it('should handle incrementByAmount', () => {
test
.givenInitialState({ value: 0 })
.whenActionIsDispatched(incrementByAmount(5))
.thenStateShouldEqual({ value: 5 });
});
});
In this example, we use the createReducerTests
function to create a test instance for our counter reducer. The chainable API allows us to easily set up the initial state, dispatch actions, and assert the resulting state.
Advanced Usage and Techniques
Testing Multiple Actions
redux-test-reducer shines when testing complex scenarios involving multiple actions:
it('should handle a sequence of actions', () => {
test
.givenInitialState({ value: 0 })
.whenActionIsDispatched(increment())
.whenActionIsDispatched(increment())
.whenActionIsDispatched(decrement())
.whenActionIsDispatched(incrementByAmount(10))
.thenStateShouldEqual({ value: 11 });
});
This test chains multiple actions together, demonstrating how the state evolves through a series of operations.
Custom Assertions
You can extend redux-test-reducer with custom assertions to suit your specific testing needs:
import { createReducerTests, TestInstance } from 'redux-test-reducer';
interface CounterState {
value: number;
}
const isEven = (test: TestInstance<CounterState>) => {
const state = test.getState();
expect(state.value % 2).toBe(0);
return test;
};
const test = createReducerTests(counterReducer);
it('should result in an even number', () => {
test
.givenInitialState({ value: 0 })
.whenActionIsDispatched(incrementByAmount(2))
.then(isEven);
});
This example defines a custom assertion isEven
that checks if the counter value is even, demonstrating how you can create domain-specific assertions.
Working with Async Actions
While redux-test-reducer primarily focuses on synchronous reducer testing, you can still use it effectively with async actions by testing the reducer’s response to the action creators’ synchronous parts:
import { createAsyncThunk } from '@reduxjs/toolkit';
const fetchUserById = createAsyncThunk(
'users/fetchByIdStatus',
async (userId: string) => {
const response = await fetch(`https://api.example.com/users/${userId}`);
return response.json();
}
);
const userSlice = createSlice({
name: 'user',
initialState: { user: null, status: 'idle' },
reducers: {},
extraReducers: (builder) => {
builder
.addCase(fetchUserById.pending, (state) => {
state.status = 'loading';
})
.addCase(fetchUserById.fulfilled, (state, action) => {
state.status = 'succeeded';
state.user = action.payload;
});
},
});
// In your test file
it('should handle fetchUserById.pending', () => {
test
.givenInitialState({ user: null, status: 'idle' })
.whenActionIsDispatched(fetchUserById.pending)
.thenStateShouldEqual({ user: null, status: 'loading' });
});
it('should handle fetchUserById.fulfilled', () => {
const mockUser = { id: '1', name: 'John Doe' };
test
.givenInitialState({ user: null, status: 'loading' })
.whenActionIsDispatched(fetchUserById.fulfilled(mockUser, 'requestId', '1'))
.thenStateShouldEqual({ user: mockUser, status: 'succeeded' });
});
This approach allows you to test how your reducer responds to different stages of an async action without dealing with the complexities of asynchronous testing.
Wrapping Up
redux-test-reducer offers a powerful and intuitive way to test Redux reducers in React applications. By simplifying the testing process, it allows developers to focus on writing meaningful tests rather than wrestling with boilerplate code. The library’s chainable API, combined with its support for custom assertions and complex scenarios, makes it an invaluable tool for ensuring the reliability of your Redux state management.
As you incorporate redux-test-reducer into your testing workflow, you’ll likely find that your tests become more readable, maintainable, and comprehensive. This, in turn, can lead to more robust applications and a smoother development process overall. Whether you’re working on a small project or a large-scale application, redux-test-reducer can help you tame the complexities of Redux testing and build with confidence.