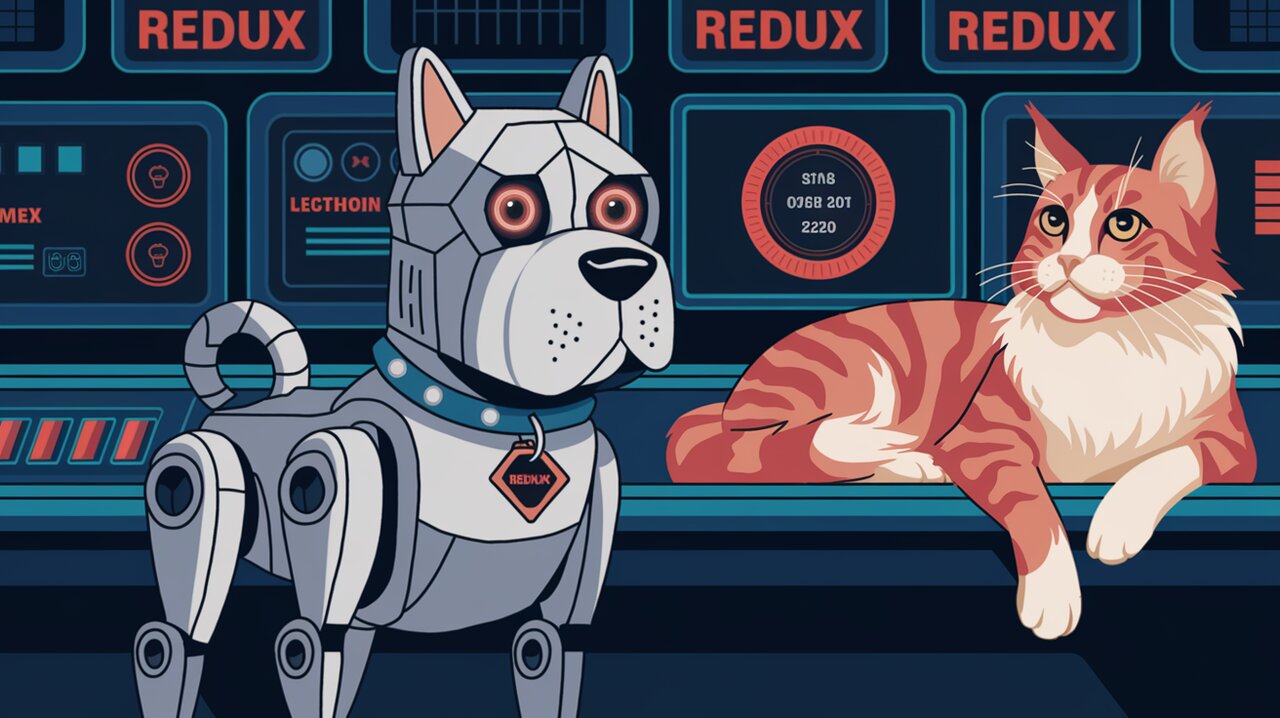
Taming Time with redux-timeout: Your Action Watchdog for Redux
In the fast-paced world of web applications, timing can make or break user experience. Whether it’s logging out an inactive user or automatically saving changes, managing time-based events is crucial. Enter redux-timeout, a powerful middleware that brings time management to your Redux-powered React applications.
Unleashing the Power of Time in Redux
redux-timeout is a specialized middleware for Redux that allows you to execute functions when specific actions haven’t been dispatched within a set time frame. It’s like having a vigilant watchdog for your actions, ready to bark (or in this case, trigger a function) when things get too quiet.
Key Features of redux-timeout
- Action-based Timeouts: Set timeouts based on specific Redux actions or groups of actions.
- Flexible Timing: Customize timeout durations for different scenarios.
- Automatic Reset: Timeouts automatically reset when watched actions are dispatched.
- Global Watching: Option to watch all actions in your Redux store.
Setting Up Your Timeout Watchdog
Let’s start by adding this time-savvy companion to your project. You can install redux-timeout using npm or yarn:
npm install --save redux-timeout
# or
yarn add redux-timeout
Once installed, it’s time to integrate the middleware into your Redux store:
import { createStore, applyMiddleware } from 'redux';
import { reduxTimeout } from 'redux-timeout';
import rootReducer from './reducers';
const store = createStore(
rootReducer,
applyMiddleware(reduxTimeout())
);
With this setup, your Redux store is now equipped to handle time-based actions!
Watchdog in Action: Basic Usage
Keeping an Eye on User Activity
Let’s implement a common scenario: logging out a user after 30 minutes of inactivity.
import { addTimeout } from 'redux-timeout';
import { LOGOUT_USER, USER_ACTION } from './actionTypes';
// In your component
useEffect(() => {
dispatch(addTimeout(
1800000, // 30 minutes in milliseconds
USER_ACTION,
() => dispatch({ type: LOGOUT_USER })
));
}, [dispatch]);
In this example, if no USER_ACTION
is dispatched for 30 minutes, the logout function will be triggered.
Auto-Save Feature: A Writer’s Best Friend
Now, let’s create an auto-save feature for a document editor:
import { addTimeout } from 'redux-timeout';
import { EDIT_DOCUMENT, SAVE_DOCUMENT } from './actionTypes';
// In your document editor component
useEffect(() => {
dispatch(addTimeout(
2000, // 2 seconds
EDIT_DOCUMENT,
() => dispatch({ type: SAVE_DOCUMENT })
));
}, [dispatch]);
This setup will automatically save the document 2 seconds after the last edit action, providing a smooth auto-save experience.
Advanced Techniques: Mastering Time Management
Watching Multiple Actions
redux-timeout allows you to watch multiple actions with a single timeout:
dispatch(addTimeout(
5000,
[MOUSE_MOVE, KEY_PRESS, SCROLL],
() => dispatch({ type: USER_ACTIVE })
));
This code will consider the user active if any of the specified actions occur within 5 seconds.
The All-Seeing Eye: Watching All Actions
For scenarios where you need to monitor all activity, use the WATCH_ALL
constant:
import { addTimeout, WATCH_ALL } from 'redux-timeout';
dispatch(addTimeout(
600000, // 10 minutes
WATCH_ALL,
() => dispatch({ type: SHOW_INACTIVITY_WARNING })
));
This will trigger a warning if no action is dispatched for 10 minutes, regardless of the action type.
Cleaning Up: Removing Timeouts
When your component unmounts or you no longer need a timeout, it’s crucial to remove it:
import { removeTimeout } from 'redux-timeout';
// In your component's cleanup function
useEffect(() => {
// ... add timeout logic
return () => {
dispatch(removeTimeout(ACTION_TO_WATCH));
};
}, [dispatch]);
This ensures that you don’t have lingering timeouts that could cause unexpected behavior.
Wrapping Up: The Time-Savvy Redux Developer
redux-timeout brings a new dimension to Redux applications, allowing developers to create more responsive and user-friendly experiences. From auto-save features to inactivity logouts, the possibilities are vast.
By leveraging this powerful middleware, you can ensure that your application not only responds to user actions but also to the absence of actions, creating a more dynamic and intelligent user interface.
Remember, in the world of web applications, timing isn’t just about speed—it’s about creating meaningful interactions that respect both the user’s time and the application’s needs. With redux-timeout, you’re well-equipped to master the art of time in your Redux applications.
For more insights on enhancing your Redux applications, check out our articles on Redux Async Queue: Orchestrating Asynchronous Actions and Redux Batched Updates: Performance Boost. Happy coding, and may your timeouts always be perfectly timed!