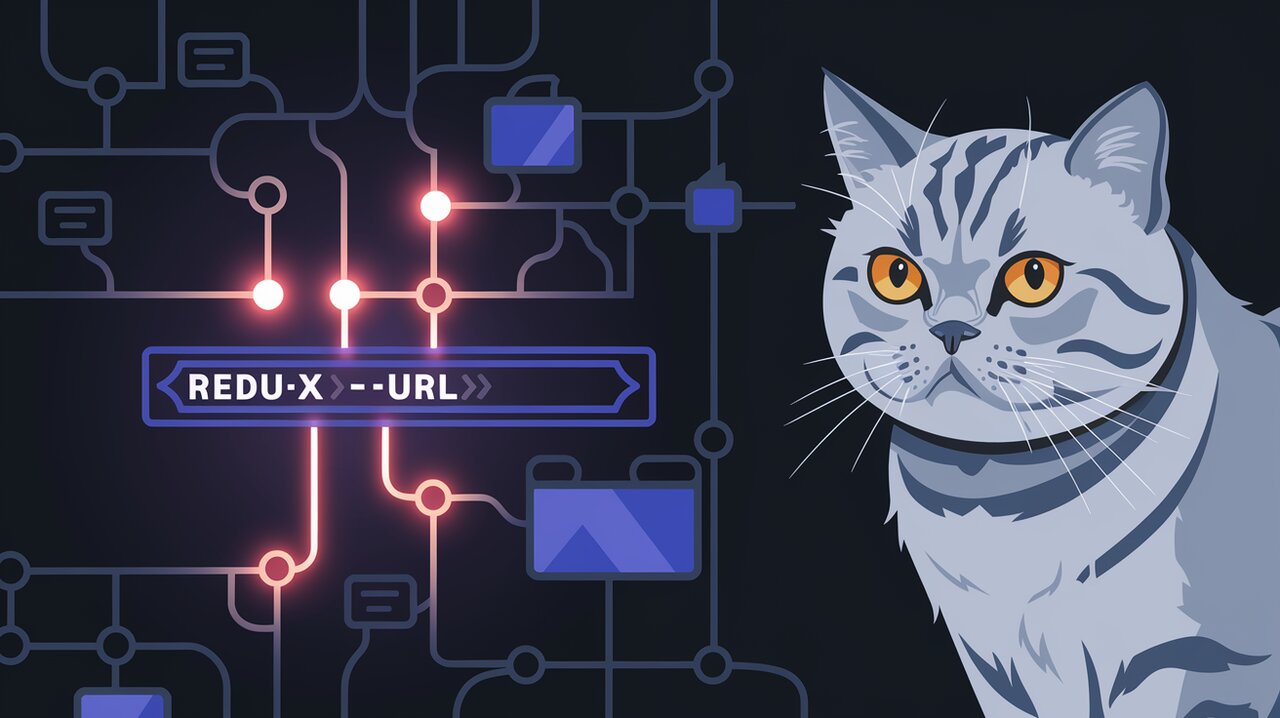
Redux-URL: Orchestrating URL and State Harmony
Redux-URL is a powerful middleware that orchestrates a harmonious symphony between your Redux store and URL parameters. This ingenious library simplifies the often complex task of synchronizing application state with the browser’s URL, enabling developers to create more intuitive and shareable React applications.
The Overture: Understanding Redux-URL
At its core, redux-url serves as a conductor, ensuring that your Redux store and URL parameters are always in perfect harmony. It provides a set of action creators for modifying the URL and dispatches actions when the URL matches user-defined routes. This bidirectional synchronization allows for a seamless integration of routing and state management in your React applications.
Installation: Setting the Stage
To begin our redux-url symphony, let’s first install the necessary components:
npm install --save redux-url history
This command installs both redux-url and the history
library, which is required for managing browser history.
Basic Usage: The First Movement
Let’s dive into the basic usage of redux-url:
import { createBrowserHistory } from 'history';
import { createStore, applyMiddleware } from 'redux';
import { createRouter, navigate } from 'redux-url';
// Define your routes
const routes = {
'/': 'HOME',
'/todos/:id': ({ id }, query) => ({
type: 'CHANGE_TODO',
payload: id,
query
}),
'*': 'NOT_FOUND'
};
// Create the router middleware
const router = createRouter(routes, createBrowserHistory());
// Create your Redux store with the router middleware
const store = createStore(
reducer,
applyMiddleware(router)
);
// Sync the initial URL state
router.sync();
// Navigate programmatically
store.dispatch(navigate('/todos/123'));
In this example, we define a set of routes, create a router middleware, and apply it to our Redux store. The sync()
method ensures that the initial URL state is reflected in the store, while the navigate()
action creator allows for programmatic navigation.
Advanced Techniques: The Second Movement
Custom Action Creators
Redux-URL allows you to define custom action creators for more complex routing scenarios:
const routes = {
'/products/:category/:id': ({ category, id }, query) => ({
type: 'VIEW_PRODUCT',
payload: {
category,
id,
filters: query.filters
}
})
};
This approach enables you to transform URL parameters and query strings into rich action objects that can be easily consumed by your reducers.
Handling Navigation Events
To react to navigation events in your components, you can listen for specific action types:
const ProductView = ({ category, id, filters }) => {
useEffect(() => {
// Fetch product data based on category, id, and filters
}, [category, id, filters]);
// Render your component
};
const mapStateToProps = (state) => ({
category: state.currentProduct.category,
id: state.currentProduct.id,
filters: state.currentProduct.filters
});
export default connect(mapStateToProps)(ProductView);
This pattern allows your components to stay in sync with the URL without directly coupling them to the routing logic.
Integrating with React Router: The Third Movement
While redux-url can be used standalone, it also plays well with popular routing libraries like React Router. Here’s how you can integrate the two:
import { Router } from 'react-router-dom';
import { createBrowserHistory } from 'history';
import { createRouter } from 'redux-url';
const history = createBrowserHistory();
const router = createRouter(routes, history);
const App = () => (
<Provider store={store}>
<Router history={history}>
{/* Your app components */}
</Router>
</Provider>
);
This integration allows you to leverage the powerful features of React Router while still benefiting from the state synchronization provided by redux-url.
The Finale: Benefits and Considerations
Redux-URL offers several advantages:
- Simplified State Management: By treating the URL as part of your application state, you can reduce the complexity of managing navigation and deep linking.
- Enhanced User Experience: Users can easily share and bookmark specific application states, improving the overall usability of your app.
- Time Travel Debugging: The integration with Redux DevTools allows you to replay user journeys and debug navigation-related issues more effectively.
However, it’s important to consider the following:
- Ensure that your URL structure is well-designed and reflects your application’s state model.
- Be mindful of performance when dealing with large amounts of data in URL parameters.
- Consider security implications when storing sensitive information in the URL.
Encore: Related Concepts
To further enhance your understanding of state management and routing in React applications, consider exploring these related articles:
- Navigating Redux Seas with React Router: Learn how to integrate React Router with Redux for more advanced routing scenarios.
- Taming Async Actions with Redux Promise Middleware: Discover how to handle asynchronous actions in Redux, which can complement your URL-based state management.
By mastering redux-url, you’re adding a powerful tool to your React development arsenal. This middleware simplifies the complex task of keeping your application state in sync with the URL, enabling you to create more intuitive and shareable web applications. As you continue to explore the world of React and Redux, remember that redux-url is there to help you orchestrate a perfect harmony between your application’s state and its URL.