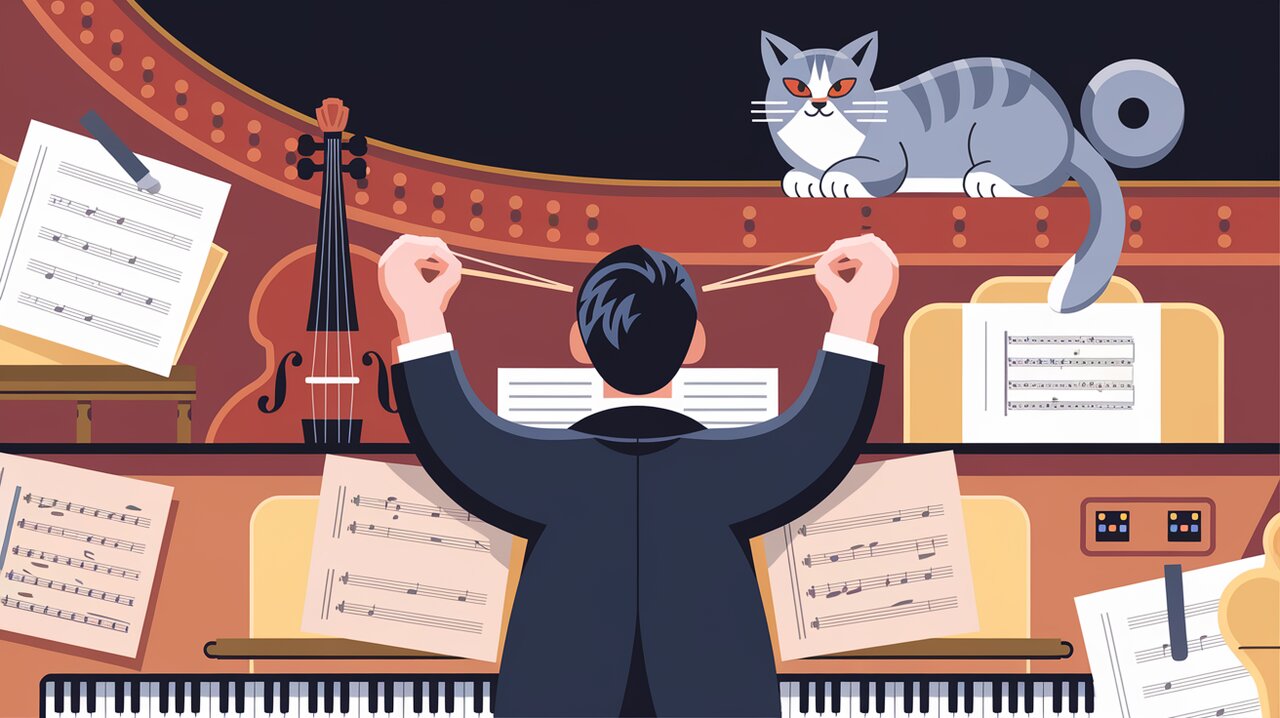
Orchestrating Redux Symphony with Redux-ViewModel
Redux-ViewModel is a powerful library designed to simplify and beautify your code when working with React and Redux. It introduces a new approach to state management by allowing developers to write ‘ViewModel’ classes, effectively reorganizing reducer implementations in Redux. With Redux-ViewModel, you can bid farewell to the tedious task of writing action factories and switch-cases to identify actions.
Key Features of Redux-ViewModel
Redux-ViewModel comes packed with features that streamline your development process:
- ViewModel Classes: Define reducer functions as methods within ViewModel classes.
- Simplified Action Dispatching: Use
viewModel.dispatch(methodName, ...args)
to trigger state updates. - Hierarchical State Management: Create sub-view-models for nested state structures.
- ListViewModel Support: Efficiently manage array states with built-in key handling.
- React Integration: Seamlessly integrate with React components using the Provider component.
Getting Started with Redux-ViewModel
To begin using Redux-ViewModel in your project, you first need to install it. You can do this using npm or yarn:
npm install redux-viewmodel
# or
yarn add redux-viewmodel
Once installed, you can import the necessary components from the library:
import { ViewModel, Provider } from 'redux-viewmodel';
Basic Usage: Creating a Counter
Let’s dive into a simple example to demonstrate how Redux-ViewModel works. We’ll create a basic counter application:
Defining the ViewModel
First, we’ll define our ViewModel classes:
class CounterViewModel extends ViewModel {
static get defaultState() {
return 0;
}
increment(state: number, val: number = 1) {
return state + val;
}
decrement(state: number, val: number = 1) {
return state - val;
}
}
class RootViewModel extends ViewModel {
static get defaultState() {
return {
counter: CounterViewModel.defaultState
};
}
get counter() {
return this._counter = this._counter || this.getSubViewModel('counter', CounterViewModel);
}
}
In this example, CounterViewModel
defines the behavior of our counter, while RootViewModel
serves as the entry point for our application state.
Creating React Components
Next, let’s create the React components that will use our ViewModels:
import React from 'react';
import { Provider } from 'redux-viewmodel';
const NumbericView: React.FC<{ value: number }> = ({ value }) => (
<span>{value}</span>
);
const rootViewModel = new RootViewModel();
const AppView: React.FC = () => (
<div>
<NumbericView value={rootViewModel.counter.state} />
<button onClick={() => rootViewModel.counter.dispatch('increment', 1)}>Inc</button>
<button onClick={() => rootViewModel.counter.dispatch('decrement', 1)}>Dec</button>
</div>
);
const App: React.FC = () => (
<Provider viewModel={rootViewModel} viewClass={AppView} />
);
This setup creates a simple counter interface with increment and decrement buttons. The Provider
component ensures that our ViewModel is properly connected to the React component tree.
Advanced Usage: ListViewModel and Sub-ViewModels
Redux-ViewModel shines when dealing with more complex state structures. Let’s explore how to use ListViewModel and sub-ViewModels:
Managing Lists with ListViewModel
ListViewModel is perfect for handling array states:
class TodoListViewModel extends ViewModel {
static get defaultState() {
return [];
}
addTodo(state: Todo[], text: string) {
return [...state, { id: Date.now(), text, completed: false }];
}
toggleTodo(state: Todo[], id: number) {
return state.map(todo =>
todo.id === id ? { ...todo, completed: !todo.completed } : todo
);
}
}
Creating Sub-ViewModels
You can create sub-ViewModels to manage different parts of your state tree:
class UserViewModel extends ViewModel {
static get defaultState() {
return { name: '', email: '' };
}
updateName(state: User, name: string) {
return { ...state, name };
}
updateEmail(state: User, email: string) {
return { ...state, email };
}
}
class AppViewModel extends ViewModel {
static get defaultState() {
return {
todos: TodoListViewModel.defaultState,
user: UserViewModel.defaultState
};
}
get todos() {
return this._todos = this._todos || this.getSubViewModel('todos', TodoListViewModel);
}
get user() {
return this._user = this._user || this.getSubViewModel('user', UserViewModel);
}
}
This structure allows you to manage complex state trees with ease, keeping your code organized and maintainable.
Integrating with React Router
Redux-ViewModel can be seamlessly integrated with React Router for state management across different routes:
import { Router, Route } from 'react-router';
import { createHistory } from 'history';
const history = createHistory();
const Site: React.FC = ({ children }) => (
<Provider
viewFactory={(props, vm) => (
<div>
<NavBar />
{children}
<Footer />
</div>
)}
viewModel={RootViewModel.instance}
/>
);
const App: React.FC = () => (
<Router history={history}>
<Route path="/" component={Site}>
<Route path="todos" component={TodoList} />
<Route path="profile" component={UserProfile} />
</Route>
</Router>
);
This setup allows you to use Redux-ViewModel’s state management capabilities across different routes in your application.
Conclusion
Redux-ViewModel offers a fresh and intuitive approach to state management in React applications. By leveraging ViewModel classes, it simplifies the process of creating and managing complex state structures, reducing boilerplate and improving code organization. Whether you’re building a simple counter or a complex application with nested state and list management, Redux-ViewModel provides the tools to make your development process smoother and more enjoyable.
As you explore Redux-ViewModel further, you might find it helpful to check out related articles on state management in React, such as “Mastering Jotai React State” or “Zustand: Simplifying React State Management”. These articles can provide additional context and alternative approaches to state management in the React ecosystem.
By embracing Redux-ViewModel, you’re not just adopting a library; you’re orchestrating a symphony of state management that harmonizes with React’s component-based architecture. Happy coding!