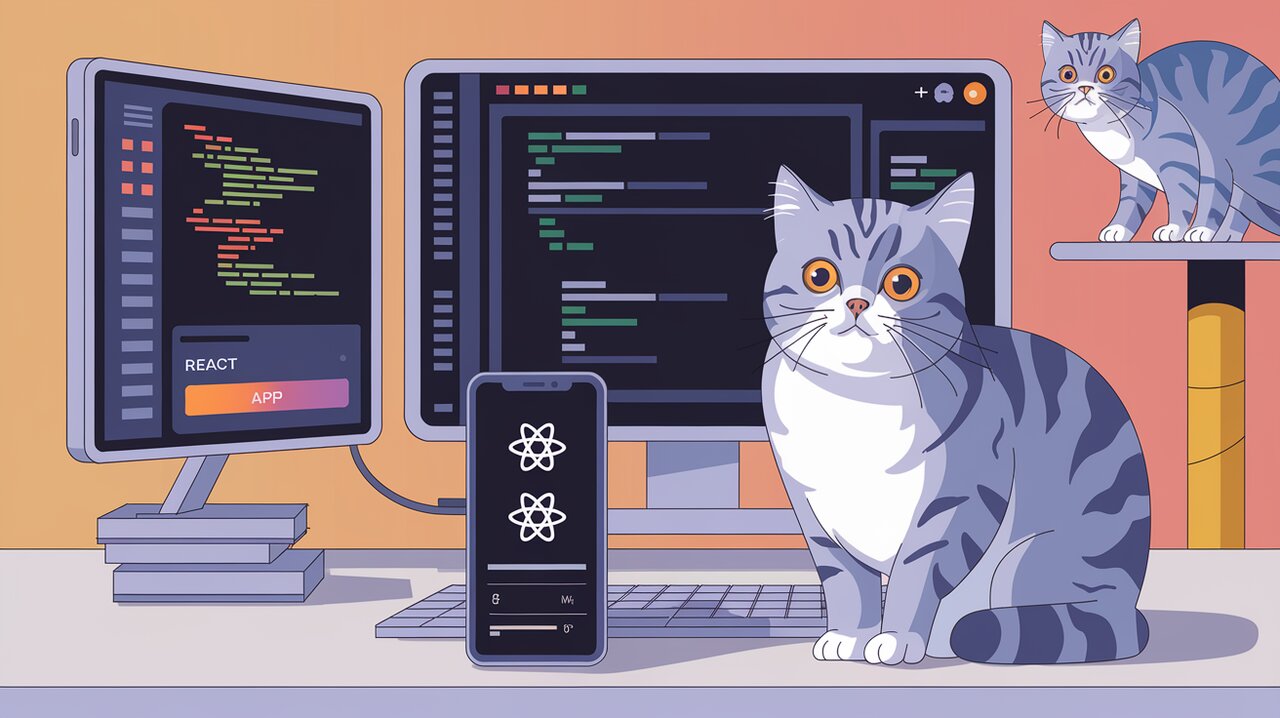
Debugging Redux Remotely in React Native with Remote Redux DevTools
Debugging Redux in React Native applications can be challenging without access to browser-based developer tools. Fortunately, the remote-redux-devtools library provides a powerful solution for remotely debugging Redux state and actions in React Native apps. This article will guide you through setting up and using remote Redux DevTools to enhance your React Native debugging experience.
Why Remote Redux DevTools?
When developing React Native apps with Redux, you lose access to the Redux DevTools browser extension. remote-redux-devtools bridges this gap by allowing you to monitor and debug your Redux store remotely, whether your app is running on a physical device or emulator.
Setting Up Remote Redux DevTools
Let’s walk through the process of integrating remote Redux DevTools into your React Native project.
Installation
First, install the necessary packages:
npm install --save-dev remote-redux-devtools
For Windows users, it’s recommended to use version 0.5.0 due to compatibility issues:
npm install --save-dev remote-redux-devtools@0.5.0
Basic Configuration
To enable remote debugging, you’ll need to modify your Redux store configuration. Here’s a basic example:
import { createStore } from 'redux';
import devToolsEnhancer from 'remote-redux-devtools';
import rootReducer from './reducers';
const store = createStore(
rootReducer,
devToolsEnhancer({ realtime: true })
);
This setup adds the remote Redux DevTools enhancer to your store, enabling real-time monitoring.
Advanced Configuration with Middleware
If your store uses middleware or other enhancers, you’ll need to use the composeWithDevTools
function to ensure proper integration:
import { createStore, applyMiddleware } from 'redux';
import { composeWithDevTools } from 'remote-redux-devtools';
import thunk from 'redux-thunk';
import rootReducer from './reducers';
const composeEnhancers = composeWithDevTools({ realtime: true, port: 8000 });
const store = createStore(
rootReducer,
composeEnhancers(
applyMiddleware(thunk)
// other store enhancers if any
)
);
This configuration ensures that actions dispatched from Redux DevTools will flow through your middleware correctly.
Using Remote Redux DevTools
Once configured, you can use various monitoring tools to inspect and interact with your Redux store:
- Redux DevTools Extension: Use the “Remote” button in the Redux DevTools browser extension.
- Standalone DevTools App: Use the remotedev-app to create a custom monitoring solution.
- Command Line Interface: Use redux-dispatch-cli for Redux remote dispatch via the terminal.
Local Server Communication
For faster connections and offline development, you can run a local server using remotedev-server:
var remotedev = require('remotedev-server');
remotedev({ hostname: 'localhost', port: 8000 });
This setup allows you to debug without an internet connection and can improve performance.
Customization Options
Remote Redux DevTools offers several configuration options to tailor the debugging experience:
name
: Set a custom name for your Redux instance.hostname
andport
: Specify the host and port for the remotedev-server.secure
: Enable HTTPS for secure connections.maxAge
: Limit the number of actions stored in history.actionSanitizer
andstateSanitizer
: Customize how actions and state are displayed.
Conclusion
Remote Redux DevTools is an invaluable tool for debugging Redux in React Native applications. By enabling remote monitoring and interaction with your Redux store, it significantly improves the development experience for React Native developers working with Redux.
For more insights on Redux and React Native development, check out our articles on mastering React Native bottom tabs and simplifying React Native storage with MMKV.
By integrating remote Redux DevTools into your workflow, you’ll gain deeper insights into your app’s state management and be better equipped to build robust React Native applications.