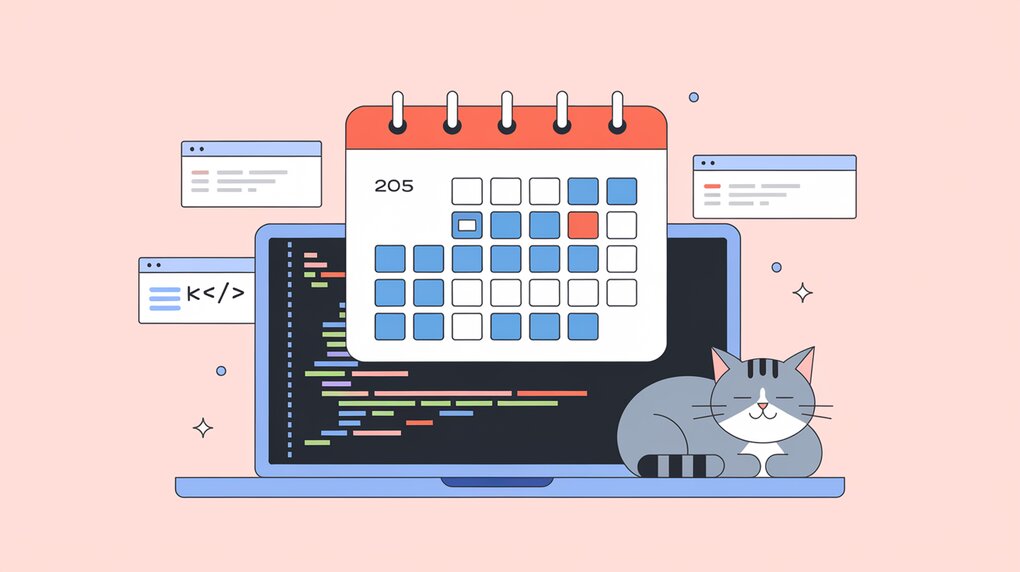
Revolutionize Date Picking in React with react-tailwindcss-datepicker
React Tailwindcss Datepicker is a powerful and flexible date range picker component designed for React applications. It leverages the popular Tailwind CSS framework and dayjs library to provide a modern, customizable solution for handling date selections in your projects. Whether you need a simple date picker or a complex date range selector, this library has you covered with its extensive features and easy integration.
Features
React Tailwindcss Datepicker comes packed with a variety of features to enhance your date picking experience:
- Single Date and Date Range Selection: Choose between picking a single date or a range of dates to suit your application’s needs.
- Customizable Appearance: Tailor the look and feel of the datepicker to match your project’s design using Tailwind CSS classes.
- Responsive Design: The component adapts seamlessly to different screen sizes, ensuring a consistent user experience across devices.
- Localization Support: Easily integrate with various languages and date formats using dayjs localization.
- Flexible Configuration: Adjust settings like min/max dates, disabled dates, and custom date formatting to meet specific requirements.
- Keyboard Navigation: Enhance accessibility with built-in keyboard navigation support.
- Plugin System: Extend functionality with plugins for features like week numbers display.
Installation
To get started with React Tailwindcss Datepicker, you’ll need to install the package along with its peer dependencies. Use one of the following commands based on your preferred package manager:
Using npm:
npm install react-tailwindcss-datepicker
Using yarn:
yarn add react-tailwindcss-datepicker
Basic Usage
Let’s dive into a simple example to demonstrate how to use React Tailwindcss Datepicker in your React application:
import React, { useState } from "react";
import Datepicker from "react-tailwindcss-datepicker";
const MyComponent: React.FC = () => {
const [value, setValue] = useState({
startDate: new Date(),
endDate: new Date().setMonth(11)
});
const handleValueChange = (newValue) => {
console.log("newValue:", newValue);
setValue(newValue);
}
return (
<Datepicker
value={value}
onChange={handleValueChange}
/>
);
}
export default MyComponent;
In this example, we import the Datepicker component and use it within a functional component. The value
state holds the selected date range, and the handleValueChange
function updates this state when a new date range is selected.
Advanced Usage
React Tailwindcss Datepicker offers numerous props to customize its behavior and appearance. Let’s explore some advanced use cases:
Custom Date Formatting
You can specify custom date formats for display and parsing:
<Datepicker
value={value}
onChange={handleValueChange}
displayFormat={"DD/MM/YYYY"}
readOnly={true}
inputClassName="w-full rounded-md focus:ring-0 font-normal bg-green-100 dark:bg-green-900"
containerClassName="relative mt-8"
/>
This example sets a custom display format, makes the input read-only, and applies custom CSS classes to the input and container elements.
Single Date Selection
To use the datepicker for single date selection:
<Datepicker
value={value}
onChange={handleValueChange}
asSingle={true}
useRange={false}
/>
Customizing the Calendar
You can customize various aspects of the calendar, such as the start day of the week:
<Datepicker
value={value}
onChange={handleValueChange}
startWeekOn="mon"
showShortcuts={true}
primaryColor={"fuchsia"}
/>
This configuration starts the week on Monday, displays shortcut buttons, and sets the primary color to fuchsia.
Using Plugins
React Tailwindcss Datepicker supports plugins to extend its functionality. Here’s how to use the week numbers plugin:
import { WeekNumbers } from "react-tailwindcss-datepicker/plugin";
<Datepicker
value={value}
onChange={handleValueChange}
plugins={[
<WeekNumbers />
]}
/>
Localization
To change the language and date format:
import dayjs from "dayjs";
import "dayjs/locale/de";
dayjs.locale("de");
<Datepicker
value={value}
onChange={handleValueChange}
displayFormat={"DD.MM.YYYY"}
readOnly={true}
inputClassName="w-full rounded-md focus:ring-0 font-normal bg-green-100 dark:bg-green-900"
containerClassName="relative mt-8"
/>
This example sets the locale to German and adjusts the display format accordingly.
Conclusion
React Tailwindcss Datepicker offers a powerful and flexible solution for implementing date and date range selection in React applications. With its extensive customization options, responsive design, and integration with Tailwind CSS, it provides developers with a versatile tool to enhance user experience in projects requiring date input. Whether you’re building a booking system, a reporting dashboard, or any application that deals with dates, this library simplifies the process while offering the flexibility to tailor the component to your specific needs.