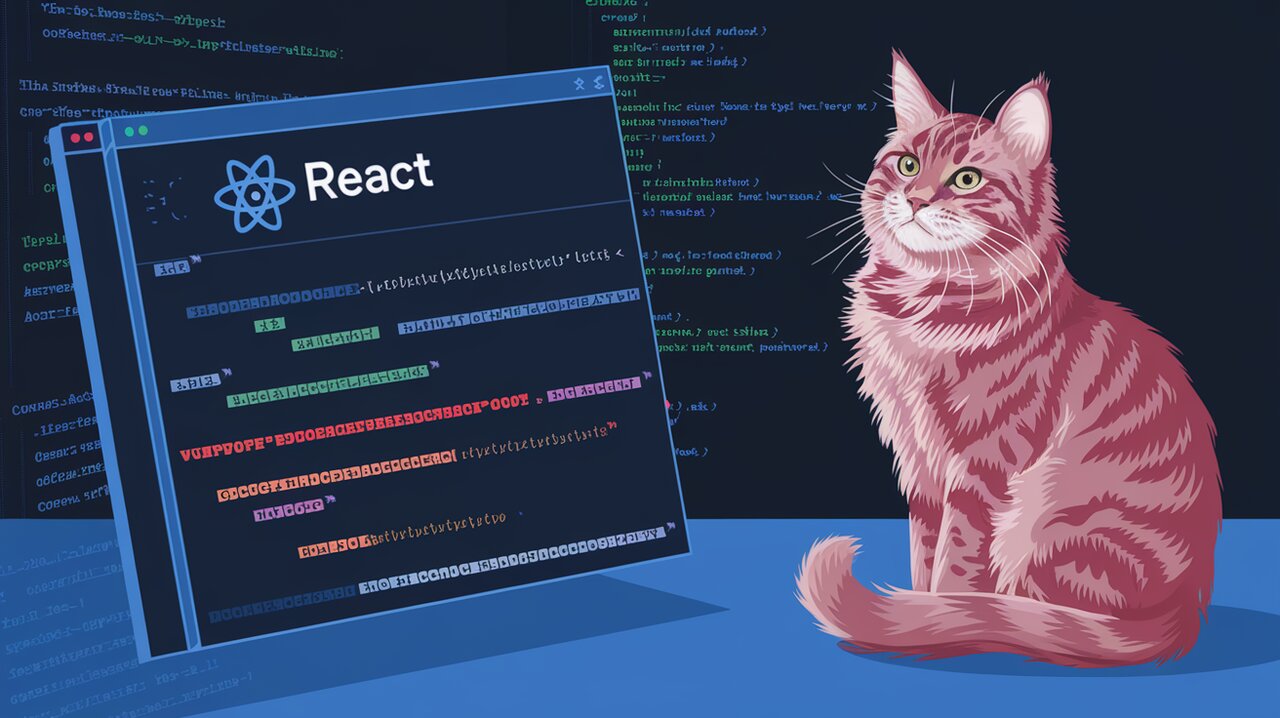
Rich Textarea: Orchestrating a Colorful Text Symphony in React
In the ever-evolving landscape of web development, creating engaging and interactive user interfaces is paramount. For React developers seeking to enhance their text input components, rich-textarea emerges as a powerful ally. This small yet mighty library transforms the humble textarea into a canvas for creativity, allowing developers to craft rich text experiences with ease.
Painting with Words: Features that Make rich-textarea Shine
rich-textarea isn’t just another text input component. It’s a Swiss Army knife for text editing in React applications. Let’s explore the palette of features that make this library stand out:
- Styleable texts: Go beyond simple highlighting. With rich-textarea, you can colorize, decorate, and style text in ways that bring your content to life.
- Event-driven interactivity: Easily capture caret positions and mouse events, opening up possibilities for context-sensitive UI elements.
- Textarea compatibility: While offering advanced features, rich-textarea maintains compatibility with native textarea behavior, ensuring a familiar user experience.
- Seamless integration: Whether you prefer controlled or uncontrolled components, rich-textarea plays nicely with popular form libraries and frameworks like Formik, react-hook-form, and even server-side rendering setups.
- IME support: International users rejoice! rich-textarea handles Input Method Editor (IME) events with grace, ensuring a smooth experience across languages.
- Lightweight footprint: At just ~3kB gzipped, rich-textarea packs a punch without weighing down your application.
Getting Started: Installing Your Text Editing Palette
Before we dive into the colorful world of rich-textarea, let’s set up our canvas. Installation is a breeze with npm or yarn:
npm install rich-textarea
# or
yarn add rich-textarea
Remember, rich-textarea requires React version 16.14 or higher. If you’re using ESM and webpack 5, make sure you’re on React 18 to avoid any react/jsx-runtime
errors.
Basic Usage: Your First Strokes
Let’s start with a simple example to see rich-textarea in action. We’ll create a controlled component that alternates the color of each character:
import React, { useState } from 'react';
import { RichTextarea } from 'rich-textarea';
const ColorfulTextarea: React.FC = () => {
const [text, setText] = useState('Type something colorful!');
return (
<RichTextarea
value={text}
style={{ width: '400px', height: '200px' }}
onChange={(e) => setText(e.target.value)}
>
{(value) => value.split('').map((char, index) => (
<span key={index} style={{ color: index % 2 === 0 ? 'blue' : 'red' }}>
{char}
</span>
))}
</RichTextarea>
);
};
export default ColorfulTextarea;
In this example, we’ve created a textarea where each character alternates between blue and red. The RichTextarea
component accepts a function as its child, which receives the current value and returns the styled content.
Advanced Techniques: Mastering the Art
Now that we’ve got our feet wet, let’s explore some more advanced techniques to truly harness the power of rich-textarea.
Regex-Powered Highlighting
rich-textarea provides a handy helper for regex-based styling. This is perfect for scenarios like syntax highlighting or emphasizing specific patterns:
import { RichTextarea, createRegexRenderer } from 'rich-textarea';
const SyntaxHighlighter: React.FC = () => {
const renderer = createRegexRenderer([
[/\b(const|let|var)\b/g, { color: 'blue', fontWeight: 'bold' }],
[/\b(function)\b/g, { color: 'purple', fontStyle: 'italic' }],
[/".*?"/g, { color: 'green' }],
]);
return (
<RichTextarea defaultValue="const greeting = 'Hello, world!';\nfunction sayHello() {\n console.log(greeting);\n}">
{renderer}
</RichTextarea>
);
};
This example creates a simple syntax highlighter for JavaScript, coloring keywords, functions, and strings differently.
Autocomplete Magic
One of the most powerful features of rich-textarea is its ability to implement autocomplete functionality. Here’s a basic example of how you might implement a mention system:
import React, { useState } from 'react';
import { RichTextarea } from 'rich-textarea';
const users = ['Alice', 'Bob', 'Charlie', 'David'];
const MentionTextarea: React.FC = () => {
const [text, setText] = useState('');
const [suggestions, setSuggestions] = useState<string[]>([]);
const handleChange = (e: React.ChangeEvent<HTMLTextAreaElement>) => {
const newText = e.target.value;
setText(newText);
const match = newText.match(/@(\w*)$/);
if (match) {
const prefix = match[1].toLowerCase();
setSuggestions(users.filter(user => user.toLowerCase().startsWith(prefix)));
} else {
setSuggestions([]);
}
};
return (
<div>
<RichTextarea
value={text}
onChange={handleChange}
style={{ width: '400px', height: '100px' }}
>
{(value) => value.split(' ').map((word, index) => (
word.startsWith('@') ? (
<span key={index} style={{ color: 'blue', fontWeight: 'bold' }}>{word}</span>
) : word
))}
</RichTextarea>
{suggestions.length > 0 && (
<ul>
{suggestions.map(user => (
<li key={user}>{user}</li>
))}
</ul>
)}
</div>
);
};
This example demonstrates a basic mention system where typing ’@’ followed by characters will show a list of matching users and highlight the mentions in blue.
Harmonizing with Other Libraries
rich-textarea’s flexibility allows it to play well with other React libraries. For instance, you could combine it with a library like react-syntax-highlighter
for more advanced code highlighting, or react-emoji-picker
to add emoji support to your text area.
If you’re working on a project that requires both rich text editing and form management, you might find our article on Formsy Material UI: Elevating React Forms helpful for integrating rich-textarea into a larger form ecosystem.
Conclusion: Your Masterpiece Awaits
rich-textarea opens up a world of possibilities for creating engaging, interactive text input experiences in React applications. From simple styling to complex features like autocomplete and syntax highlighting, this library provides the tools you need to craft textareas that are both functional and visually appealing.
As you continue to explore the capabilities of rich-textarea, remember that the key to creating truly outstanding user interfaces lies in balancing functionality with user experience. Don’t be afraid to experiment with different styles and features to find what works best for your specific use case.
For those looking to further enhance their React UI toolkit, you might also be interested in exploring React Toastify for Dynamic Notifications, which can complement your rich text inputs with sleek, customizable notifications.
With rich-textarea in your arsenal, you’re well-equipped to create text input components that not only meet user expectations but exceed them, turning ordinary forms into extraordinary experiences. Happy coding, and may your text areas be ever rich and engaging!