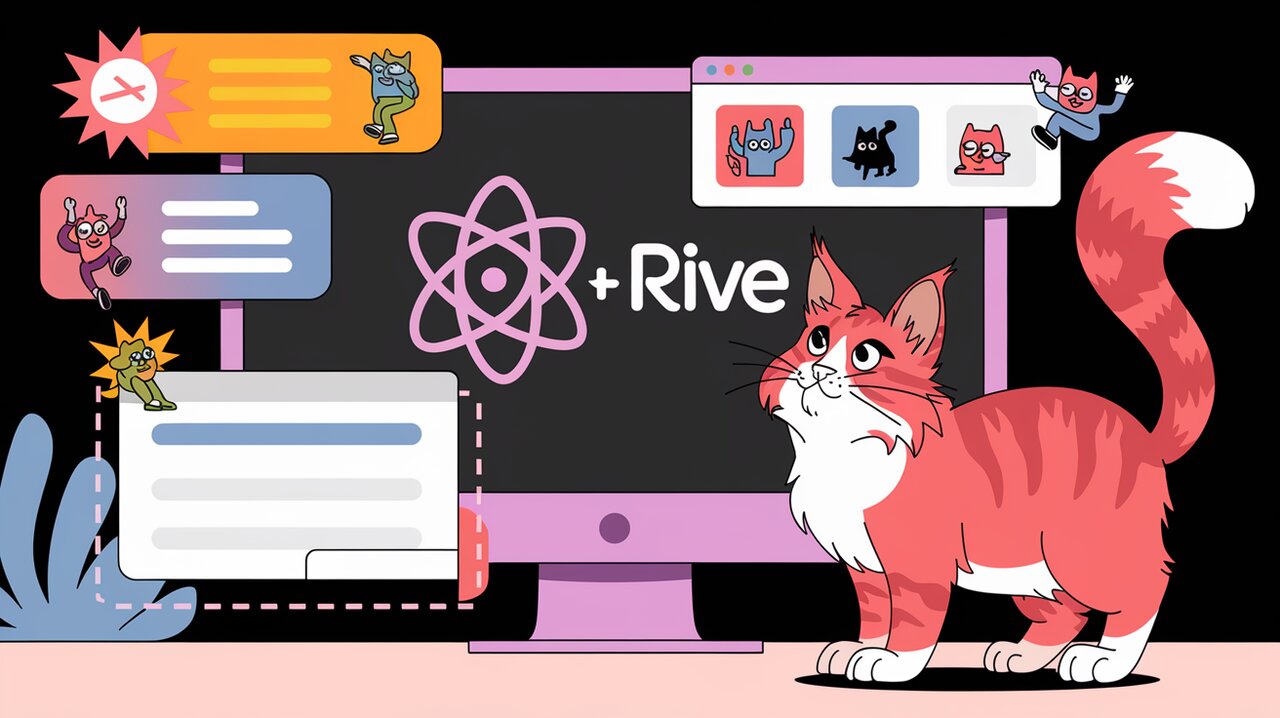
Rive-react is a powerful library that brings the magic of interactive animations to your React applications. By leveraging the capabilities of Rive, a real-time interactive design and animation tool, developers can create engaging user interfaces that respond dynamically to user inputs and state changes. In this comprehensive guide, we’ll explore how to harness the full potential of rive-react to elevate your React projects.
Features
Rive-react offers a rich set of features that make it an invaluable tool for React developers:
- Seamless integration with React components
- Support for both canvas and WebGL rendering
- State machine integration for complex interactions
- Responsive layouts and sizing options
- Event handling and animation controls
- TypeScript support for enhanced development experience
Installation
To get started with rive-react, you’ll need to install the package in your React project. The library offers different versions based on your rendering needs:
# For canvas rendering (recommended)
npm install @rive-app/react-canvas
# For WebGL rendering
npm install @rive-app/react-webgl
# For a lightweight version without Rive Text support
npm install @rive-app/react-canvas-lite
For most applications, the canvas renderer (@rive-app/react-canvas
) is recommended due to its balance of performance and file size.
Basic Usage
Rendering a Simple Animation
Let’s start with a basic example of how to render a Rive animation in your React component:
import Rive from '@rive-app/react-canvas';
const SimpleAnimation = () => (
<Rive
src="https://cdn.rive.app/animations/vehicles.riv"
stateMachines="bumpy"
style={{ width: 400, height: 300 }}
/>
);
This code snippet creates a Rive component that loads an animation from a URL and plays a state machine named “bumpy”. The style
prop sets the dimensions of the animation container.
Understanding the Rendered Output
When you use the Rive component, it creates a <div>
element containing a <canvas>
. The <div>
wrapper helps control the sizing of the animation. By default, it has a width and height of 100%, but you can override these styles by passing a className
or style
prop to the Rive component.
Advanced Usage
Using the useRive Hook
For more control over your Rive animations, you can use the useRive
hook. This hook provides access to the Rive instance, allowing you to manipulate animations programmatically:
import { useRive } from '@rive-app/react-canvas';
const AdvancedAnimation = () => {
const { rive, RiveComponent } = useRive({
src: 'https://cdn.rive.app/animations/vehicles.riv',
stateMachines: 'bumpy',
autoplay: false,
});
return (
<div>
<RiveComponent
onMouseEnter={() => rive && rive.play()}
onMouseLeave={() => rive && rive.pause()}
/>
<button onClick={() => rive && rive.reset()}>Reset Animation</button>
</div>
);
};
In this example, we use the useRive
hook to get access to the rive
instance and the RiveComponent
. We can then control the animation playback based on user interactions.
Working with State Machines
Rive’s state machines allow for complex, interactive animations. Here’s how you can interact with a state machine:
import { useStateMachineInput, useRive } from '@rive-app/react-canvas';
const InteractiveAnimation = () => {
const { rive, RiveComponent } = useRive({
src: 'https://cdn.rive.app/animations/skills.riv',
stateMachines: 'Designer',
autoplay: true,
});
const levelInput = useStateMachineInput(rive, 'Designer', 'Level');
return (
<div>
<RiveComponent />
<input
type="range"
min="0"
max="100"
onChange={(e) => levelInput && levelInput.value = parseInt(e.target.value)}
/>
</div>
);
};
This example demonstrates how to use the useStateMachineInput
hook to connect a slider input to a state machine parameter in the Rive animation.
Handling Events
Rive animations can trigger custom events that you can listen to in your React components:
import { useRive } from '@rive-app/react-canvas';
const EventHandlingAnimation = () => {
const { rive, RiveComponent } = useRive({
src: 'https://cdn.rive.app/animations/game.riv',
stateMachines: 'Game',
onStateChange: (event) => {
console.log('State changed:', event.data);
},
});
return <RiveComponent />;
};
This setup allows you to respond to state changes in your Rive animation, enabling you to synchronize your React application state with the animation state.
Conclusion
Rive-react opens up a world of possibilities for creating engaging, interactive animations in your React applications. From simple animated components to complex, state-driven interfaces, this library provides the tools you need to bring your UI to life.
By leveraging the power of Rive’s real-time animations and React’s component-based architecture, you can create truly dynamic and responsive user experiences. Whether you’re building a simple animated button or a full-fledged interactive game, rive-react offers the flexibility and performance to meet your needs.
As you continue to explore the capabilities of rive-react, remember to check the official documentation for the most up-to-date information and advanced usage scenarios. Happy animating!