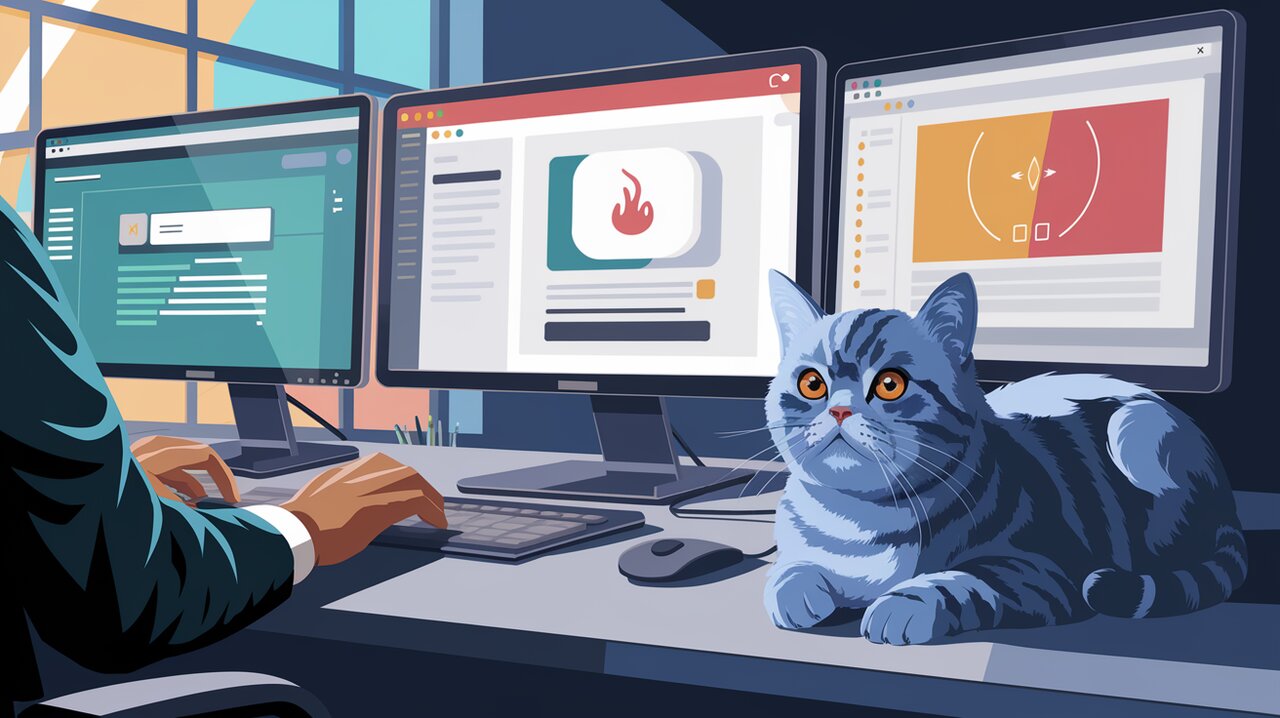
Orchestrating Scrolls with rc-scrollbars: A React Scrolling Symphony
In the ever-evolving landscape of web development, even the smallest details can make a significant impact on user experience. One such detail that often goes unnoticed but plays a crucial role is the humble scrollbar. Enter rc-scrollbars, a powerful React library that elevates the scrolling experience to new heights.
Unveiling the Scrolling Symphony
rc-scrollbars is a rejuvenated project based on the popular react-custom-scrollbars library. It brings a host of features that make it an indispensable tool for React developers looking to enhance their applications’ scrolling capabilities.
Key Features That Strike a Chord
- Frictionless Native Browser Scrolling: Enjoy smooth, native-like scrolling without compromising on performance.
- Mobile-Friendly: Automatically switches to native scrollbars on mobile devices for optimal user experience.
- Fully Customizable: Tailor the scrollbars to match your application’s design language perfectly.
- Auto-hide Functionality: Keep your interface clean with scrollbars that appear only when needed.
- Auto-height Support: Adapt to content dynamically, ensuring a seamless layout.
- Universal Rendering: Works flawlessly on both client and server-side, perfect for SSR applications.
- Performance-Driven: Utilizes
requestAnimationFrame
for buttery-smooth 60fps scrolling. - Lightweight: No extra stylesheets required, keeping your bundle size in check.
- Battle-Tested: Boasts 100% code coverage, ensuring reliability and stability.
Composing Your Scrollbar Masterpiece
Let’s dive into how you can integrate rc-scrollbars into your React project and start creating beautiful, functional scrollbars.
Installation: The First Note
Begin by installing the library using your preferred package manager:
npm install rc-scrollbars --save
# OR
yarn add rc-scrollbars
Basic Usage: A Simple Melody
To get started, import the Scrollbars
component and wrap it around your content:
import React from 'react';
import { Scrollbars } from 'rc-scrollbars';
const App: React.FC = () => {
return (
<Scrollbars style={{ width: 500, height: 300 }}>
<p>Your content goes here...</p>
</Scrollbars>
);
};
export default App;
This basic setup provides a scrollable container with default styling. The style
prop allows you to set the dimensions of the scrollable area.
Advanced Composition: Orchestrating Complex Scrollbars
For more control over your scrollbars, rc-scrollbars offers a wide array of props and customization options:
import React from 'react';
import { Scrollbars } from 'rc-scrollbars';
const CustomScrollbars: React.FC = () => {
const handleScroll = () => {
console.log('Scrolling');
};
const renderView = ({ style, ...props }) => {
const viewStyle = {
...style,
backgroundColor: '#f0f0f0',
};
return <div style={viewStyle} {...props} />;
};
const renderThumb = ({ style, ...props }) => {
const thumbStyle = {
...style,
backgroundColor: '#6b6b6b',
borderRadius: '4px',
};
return <div style={thumbStyle} {...props} />;
};
return (
<Scrollbars
onScroll={handleScroll}
renderView={renderView}
renderThumbHorizontal={renderThumb}
renderThumbVertical={renderThumb}
autoHide
autoHideTimeout={1000}
autoHideDuration={200}
thumbMinSize={30}
universal={true}
>
<div style={{ width: 1000, height: 1000 }}>
<h1>Scrollable Content</h1>
<p>Lots of content here...</p>
</div>
</Scrollbars>
);
};
export default CustomScrollbars;
In this advanced example, we’ve customized the scrollbar’s behavior and appearance:
- The
onScroll
prop allows you to handle scroll events. - Custom rendering functions (
renderView
,renderThumbHorizontal
,renderThumbVertical
) enable complete control over the scrollbar’s look. autoHide
,autoHideTimeout
, andautoHideDuration
create a sleek auto-hiding scrollbar.thumbMinSize
ensures the scrollbar thumb remains usable even with large content.- The
universal
prop enables server-side rendering support.
Harmonizing with Other React Libraries
rc-scrollbars plays well with other React libraries. For instance, you can combine it with state management solutions like Redux for more complex applications. Check out our article on Blue Chip React State Management Symphony for insights on integrating state management with UI components like scrollbars.
Additionally, if you’re working on a project that requires both custom scrollbars and dynamic layouts, you might find our guide on Crafting Responsive Layouts with React Schematic helpful in creating a harmonious user interface.
The Final Crescendo
rc-scrollbars offers a powerful yet flexible solution for implementing custom scrollbars in your React applications. Its blend of performance, customization, and ease of use makes it an excellent choice for developers looking to enhance their user interfaces.
By leveraging the library’s features, you can create scrolling experiences that are not only functional but also aesthetically pleasing and perfectly tailored to your application’s needs. Whether you’re building a complex dashboard, a content-rich blog, or any application that demands smooth scrolling, rc-scrollbars provides the tools you need to orchestrate a perfect scrolling symphony.
Remember, in the world of web development, it’s often the subtle details that elevate a good user experience to a great one. With rc-scrollbars, you have the power to make every scroll a delight for your users.