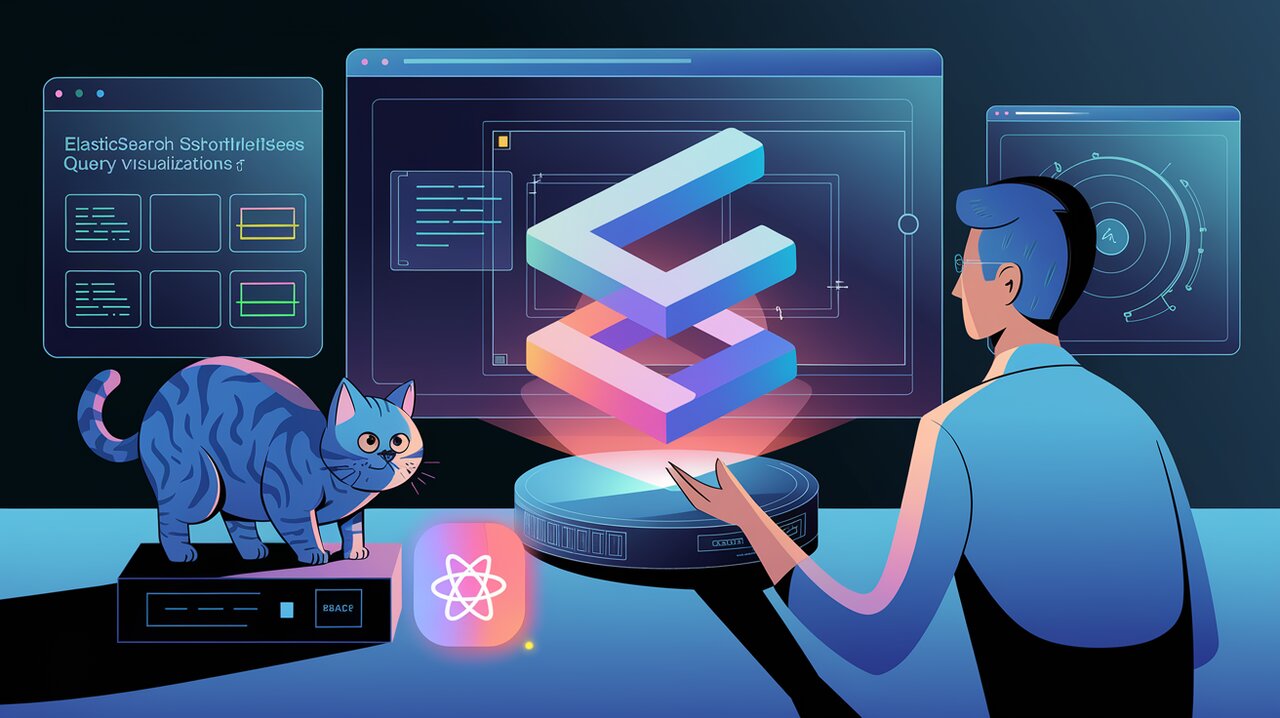
Unleashing the Power of Searchkit Elastic UI for React: A Developer's Dream
Searchkit Elastic UI is a powerful suite of React components that integrates seamlessly with Elasticsearch to create stunning search experiences. Built on top of the @elastic/eui UI framework, this library empowers developers to rapidly implement feature-rich search interfaces without sacrificing customization or performance.
Unveiling Searchkit’s Arsenal
Searchkit Elastic UI comes packed with an array of features designed to streamline the development process:
React-Ready Components: A comprehensive set of pre-built components tailored for search interfaces. Elasticsearch Integration: Seamless connection with Elasticsearch, leveraging its powerful search capabilities. Customizable UI: Built on @elastic/eui, allowing for consistent and customizable designs. Responsive Design: Components that adapt to various screen sizes and devices. Advanced Search Functionality: Support for facets, filters, pagination, and more out of the box.
Setting Up Your Search Adventure
To embark on your Searchkit journey, start by installing the necessary packages:
npm install @searchkit/elastic-ui @elastic/eui @elastic/datemath @emotion/react
For those who prefer Yarn:
yarn add @searchkit/elastic-ui @elastic/eui @elastic/datemath @emotion/react
Crafting Your First Search Experience
Let’s dive into creating a basic search interface using Searchkit Elastic UI:
Initializing Searchkit
First, set up your Searchkit instance:
import Searchkit from "searchkit";
const searchkit = new Searchkit({
connection: {
host: "http://localhost:9200",
index: "my-index"
},
search_settings: {
search_attributes: ["title", "description"],
result_attributes: ["title", "description", "url"]
}
});
This configuration establishes a connection to your Elasticsearch instance and defines the fields to be searched and displayed.
Creating the Search Interface
Now, let’s build a simple search interface:
import React from "react";
import { EuiPage, EuiPageBody, EuiPageContent } from "@elastic/eui";
import { SearchkitProvider, SearchBar, Hits } from "@searchkit/elastic-ui";
const App = () => (
<SearchkitProvider searchkit={searchkit}>
<EuiPage>
<EuiPageBody>
<EuiPageContent>
<SearchBar loading={false} />
<Hits />
</EuiPageContent>
</EuiPageBody>
</EuiPage>
</SearchkitProvider>
);
export default App;
This code snippet creates a basic search page with a search bar and results display.
Enhancing Your Search Experience
Searchkit Elastic UI offers various components to refine and improve your search interface:
Adding Faceted Search
Implement faceted navigation to allow users to filter results:
import { RefinementList } from "@searchkit/elastic-ui";
// Inside your component
<RefinementList field="category" title="Categories" />
Pagination for Large Result Sets
Handle large result sets with ease using the Pagination component:
import { Pagination } from "@searchkit/elastic-ui";
// Inside your component
<Pagination />
Advanced Customization
Searchkit Elastic UI provides flexibility for advanced customization:
Custom Result Rendering
Create a custom hit component to control how each result is displayed:
const CustomHitComponent = ({ hit }) => (
<div>
<h3>{hit.title}</h3>
<p>{hit.description}</p>
</div>
);
// Use in your Hits component
<Hits hitComponent={CustomHitComponent} />
Query Customization
Tailor the Elasticsearch query to your specific needs:
const customQuery = (query, search_attributes) => ({
multi_match: {
query,
fields: search_attributes,
type: "best_fields"
}
});
// Use in your Searchkit configuration
const searchkit = new Searchkit({
// ... other config
getQuery: customQuery
});
Conclusion
Searchkit Elastic UI for React revolutionizes the way developers create search interfaces for Elasticsearch. With its rich set of components, seamless integration, and high customizability, it stands as a powerful tool in any React developer’s arsenal. Whether you’re building a simple search page or a complex search application, Searchkit Elastic UI provides the building blocks to create engaging and efficient search experiences.
By leveraging this library, developers can focus on crafting the perfect user experience while Searchkit handles the intricacies of Elasticsearch integration. As you continue to explore Searchkit Elastic UI, you’ll discover even more ways to enhance and refine your search interfaces, making your applications more powerful and user-friendly.