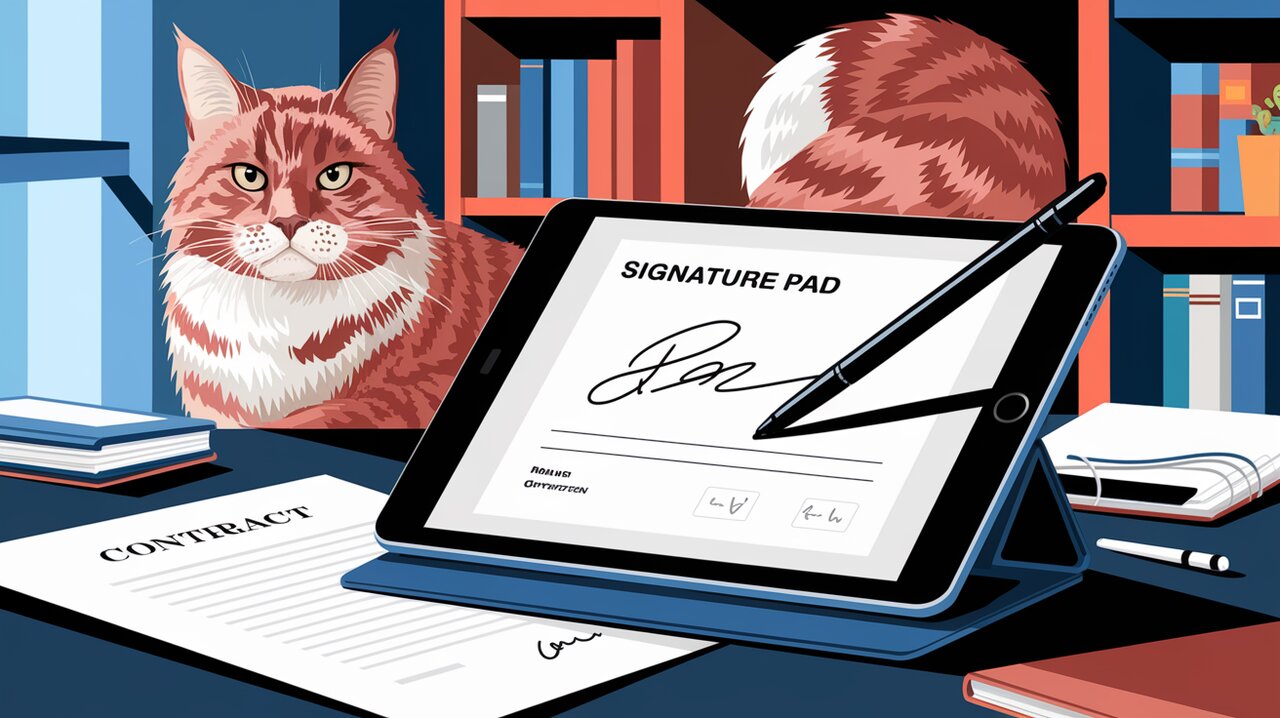
Sign on the Dotted Line: Mastering React Signature Pad Wrapper
In the era of digital transformation, the ability to capture and process signatures electronically has become a crucial feature for many web applications. Whether you’re building an e-commerce platform, a document management system, or any application that requires user consent, integrating a signature pad can significantly streamline your processes. Enter React Signature Pad Wrapper, a powerful library that brings the functionality of signature capture to your React applications with ease and flexibility.
Unveiling React Signature Pad Wrapper
React Signature Pad Wrapper is a React component that wraps the popular signature_pad
library, providing a seamless integration for React developers. This wrapper maintains the full functionality of the original library while adding React-specific features and optimizations.
Key Features
React Signature Pad Wrapper comes packed with features that make it a go-to choice for developers:
- Easy Integration: Seamlessly integrates into React applications
- Responsive Design: Adapts to different screen sizes and orientations
- Customizable: Offers various options to tailor the signature pad to your needs
- Touch and Mouse Support: Works with both touch devices and mouse input
- Data Export: Allows saving signatures as images or raw data
Getting Started
To begin using React Signature Pad Wrapper in your project, you first need to install it via npm:
npm install --save react-signature-pad-wrapper
Once installed, you can import and use the component in your React application:
import React from 'react';
import SignaturePad from 'react-signature-pad-wrapper';
const SignatureComponent = () => {
return <SignaturePad />;
};
export default SignatureComponent;
This basic implementation will render a signature pad with default settings.
Customizing Your Signature Pad
React Signature Pad Wrapper allows for extensive customization through props. Here’s how you can tailor the signature pad to your specific needs:
import React, { useRef } from 'react';
import SignaturePad from 'react-signature-pad-wrapper';
const CustomSignaturePad = () => {
const signaturePadRef = useRef(null);
return (
<SignaturePad
ref={signaturePadRef}
options={{
minWidth: 5,
maxWidth: 10,
penColor: 'rgb(66, 133, 244)'
}}
width={500}
height={200}
/>
);
};
In this example, we’ve set custom dimensions for the pad and adjusted the pen properties. The ref
allows us to access the signature pad’s methods imperatively.
Handling Signature Data
One of the most powerful features of React Signature Pad Wrapper is the ability to save and clear signatures programmatically. Here’s how you can implement these functionalities:
import React, { useRef } from 'react';
import SignaturePad from 'react-signature-pad-wrapper';
const SignatureWithControls = () => {
const signaturePadRef = useRef(null);
const handleClear = () => {
signaturePadRef.current.clear();
};
const handleSave = () => {
if (signaturePadRef.current.isEmpty()) {
alert('Please provide a signature first.');
} else {
const dataURL = signaturePadRef.current.toDataURL();
console.log(dataURL);
// You can now send this dataURL to your server or use it as needed
}
};
return (
<div>
<SignaturePad ref={signaturePadRef} />
<button onClick={handleClear}>Clear</button>
<button onClick={handleSave}>Save</button>
</div>
);
};
This setup allows users to clear their signature and save it as a data URL, which can then be processed or stored as needed.
Responsive Signature Pads
React Signature Pad Wrapper shines when it comes to creating responsive signature pads. By omitting the width
and height
props, the component becomes responsive:
import React from 'react';
import SignaturePad from 'react-signature-pad-wrapper';
const ResponsiveSignaturePad = () => {
return <SignaturePad redrawOnResize />;
};
The redrawOnResize
prop ensures that the signature is redrawn when the window is resized, maintaining the integrity of the signature across different screen sizes.
Advanced Usage: Custom Styling
While React Signature Pad Wrapper provides great out-of-the-box styling, you might want to customize its appearance further. You can achieve this by wrapping the component and applying custom CSS:
import React from 'react';
import SignaturePad from 'react-signature-pad-wrapper';
import './CustomSignaturePad.css';
const CustomStyledSignaturePad = () => {
return (
<div className="signature-pad-container">
<SignaturePad
options={{
backgroundColor: 'rgb(255, 255, 240)',
penColor: 'rgb(0, 0, 255)'
}}
/>
</div>
);
};
In your CSS file, you can then style the container and even add a border or other decorative elements:
.signature-pad-container {
border: 2px solid #ccc;
border-radius: 4px;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.1);
margin: 20px auto;
max-width: 500px;
}
Conclusion
React Signature Pad Wrapper offers a robust solution for integrating signature functionality into React applications. Its ease of use, customization options, and responsive design make it an excellent choice for developers looking to add digital signature capabilities to their projects.
By leveraging this library, you can create user-friendly, legally compliant signature interfaces that work across devices. Whether you’re building a contract signing platform, a delivery confirmation system, or any application that requires user signatures, React Signature Pad Wrapper provides the tools you need to get the job done efficiently and effectively.
As you continue to explore the world of React libraries, you might also be interested in other input-related components. For instance, the article on mastering React Input Masking could provide valuable insights into handling formatted input fields. Additionally, if you’re working on forms that require various types of user input, the guide on Uniforms: React Form Building Made Easy could be a great complementary read.
Remember, the key to building great React applications lies in choosing the right tools for the job and understanding how to use them effectively. With React Signature Pad Wrapper in your toolkit, you’re well-equipped to handle digital signature requirements in your next project.