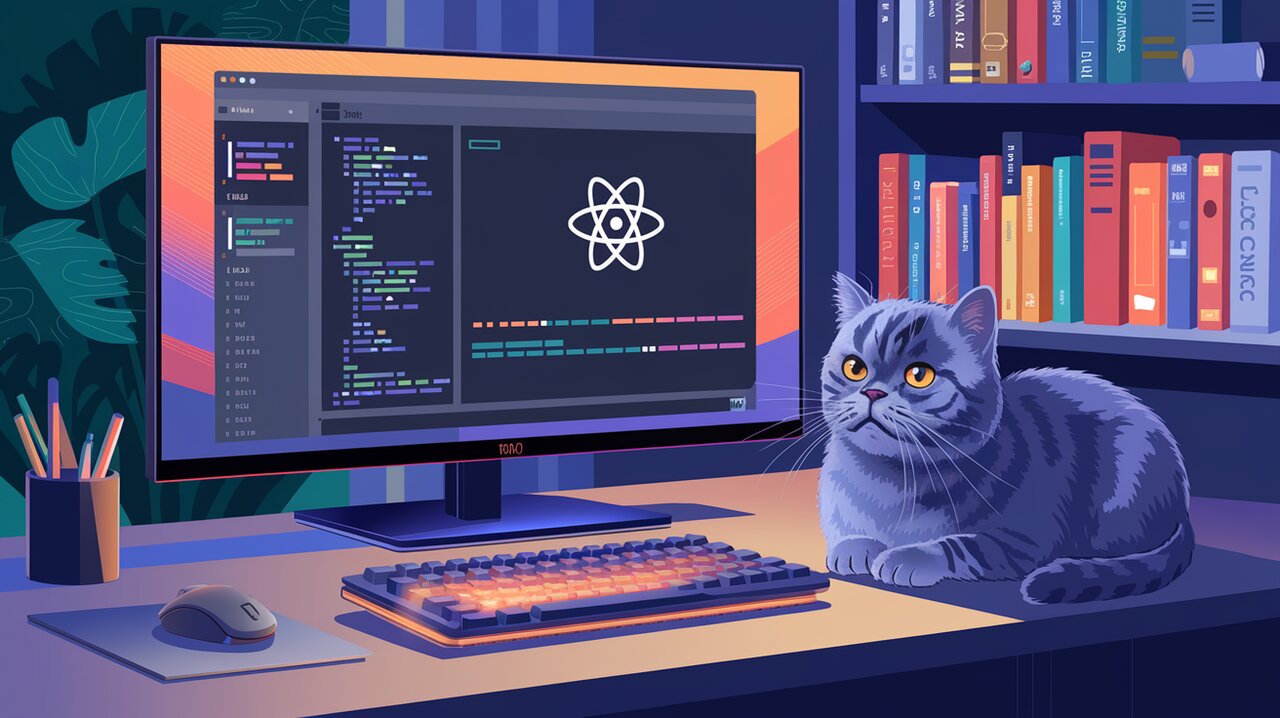
Simplify Markdown Editing in React with SimpleMDE
Elevate Your Markdown Editing Experience
In the world of web development, creating intuitive and powerful text editors is crucial for enhancing user experience. When it comes to markdown editing in React applications, the react-simplemde-editor library stands out as an excellent choice. This wrapper for EasyMDE (a popular SimpleMDE fork) brings a feature-rich markdown editor to your React projects with minimal effort.
Getting Started with react-simplemde-editor
Installation
To begin using react-simplemde-editor in your project, you’ll need to install both the main package and its peer dependency:
npm install --save react-simplemde-editor easymde
Basic Usage
Let’s dive into a simple example of how to implement the editor in your React component:
import React, { useState, useCallback } from "react";
import SimpleMDE from "react-simplemde-editor";
import "easymde/dist/easymde.min.css";
const MarkdownEditor = () => {
const [value, setValue] = useState("Initial value");
const onChange = useCallback((value: string) => {
setValue(value);
}, []);
return <SimpleMDE value={value} onChange={onChange} />;
};
export default MarkdownEditor;
This basic setup creates a controlled component where the editor’s content is managed by React state. The onChange
callback updates the state as the user types, ensuring your application always has access to the latest content.
Customizing Your Editor
Options Galore
One of the strengths of react-simplemde-editor is its extensive customization options. You can tailor the editor to your specific needs using the options
prop:
import React, { useState, useCallback, useMemo } from "react";
import SimpleMDE from "react-simplemde-editor";
import "easymde/dist/easymde.min.css";
const CustomizedEditor = () => {
const [value, setValue] = useState("Initial value");
const onChange = useCallback((value: string) => {
setValue(value);
}, []);
const options = useMemo(() => {
return {
autofocus: true,
spellChecker: false,
toolbar: ["bold", "italic", "heading", "|", "quote", "unordered-list", "ordered-list", "|", "link", "image", "|", "preview"],
};
}, []);
return <SimpleMDE value={value} onChange={onChange} options={options} />;
};
export default CustomizedEditor;
In this example, we’ve customized the toolbar and disabled the spell checker. Remember to use useMemo
to memoize your options object to prevent unnecessary re-renders.
Handling Hotkeys
Enhance user productivity by adding custom hotkeys to your editor:
const extraKeys = useMemo(() => {
return {
"Ctrl-S": (cm: any) => {
saveContent(cm.getValue());
},
"Cmd-S": (cm: any) => {
saveContent(cm.getValue());
},
};
}, []);
return <SimpleMDE value={value} onChange={onChange} extraKeys={extraKeys} />;
This setup adds a save functionality when the user presses Ctrl+S or Cmd+S.
Advanced Features
Custom Preview Rendering
For those who need more control over how markdown is rendered in the preview pane, react-simplemde-editor allows for custom preview rendering:
import ReactDOMServer from "react-dom/server";
import ReactMarkdown from "react-markdown";
const customRendererOptions = useMemo(() => {
return {
previewRender(text: string) {
return ReactDOMServer.renderToString(
<ReactMarkdown>{text}</ReactMarkdown>
);
},
};
}, []);
return <SimpleMDE options={customRendererOptions} />;
This example uses react-markdown
to render the preview, allowing for more complex markdown parsing and rendering.
Autosaving Content
Implement autosaving to prevent loss of user input:
const autosaveOptions = useMemo(() => {
return {
autosave: {
enabled: true,
uniqueId: "my-unique-id",
delay: 1000,
},
};
}, []);
return <SimpleMDE options={autosaveOptions} />;
This configuration automatically saves the editor’s content to localStorage every second.
Accessing Editor Instances
Sometimes you might need direct access to the SimpleMDE or CodeMirror instances. react-simplemde-editor provides callbacks for this purpose:
const getMdeInstance = useCallback((instance: SimpleMDE) => {
console.log("SimpleMDE instance:", instance);
}, []);
const getCodemirrorInstance = useCallback((instance: any) => {
console.log("CodeMirror instance:", instance);
}, []);
return (
<SimpleMDE
getMdeInstance={getMdeInstance}
getCodemirrorInstance={getCodemirrorInstance}
/>
);
These callbacks give you access to the underlying editor instances, allowing for more advanced manipulations when needed.
Conclusion
The react-simplemde-editor library offers a powerful and flexible solution for integrating markdown editing into your React applications. With its extensive customization options, support for advanced features, and seamless React integration, it’s an excellent choice for developers looking to provide a rich text editing experience.
Whether you’re building a blog platform, a documentation site, or any application that requires markdown input, react-simplemde-editor provides the tools you need to create a polished and user-friendly editing interface. By leveraging its features and customization options, you can tailor the editor to fit your specific requirements and enhance your users’ content creation experience.