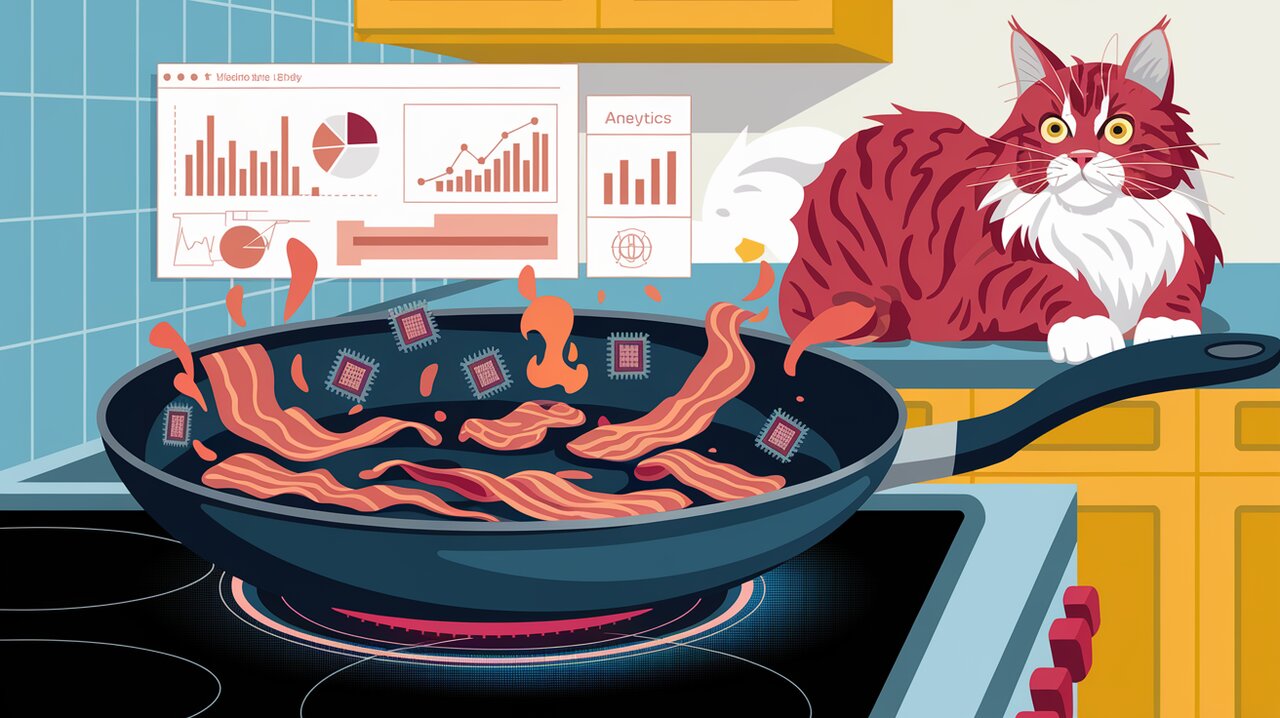
Sizzling State Management with Redux Bacon: Fry Up Your Analytics Game
Redux Bacon is a mouthwatering addition to the Redux ecosystem, designed to help developers easily track and analyze user actions and state changes in their React applications. This lightweight middleware acts as a perfect seasoning for your state management, allowing you to map dispatched actions to analytics events that can be consumed by various analytics services. Let’s dive into this sizzling library and see how it can spice up your development process!
Crispy Features of Redux Bacon
Redux Bacon comes with a smorgasbord of delectable features that make it stand out:
- Lightweight: The core module is tiny, weighing in at just about 1KB.
- Framework Agnostic: While it’s commonly used with React, Redux Bacon can be paired with any JavaScript framework.
- Flexible Targeting: Send your analytics data to popular services or create custom targets.
- Offline Support: Keep tracking even when the internet connection goes cold.
- Extensible: A buffet of extensions and utilities to enhance your analytics tracking.
Serving Up Redux Bacon
Let’s start by adding Redux Bacon to your project’s menu. You can install it using npm or yarn:
## Using npm
npm install redux-bacon
## Using yarn
yarn add redux-bacon
Cooking Up Basic Usage
Now that we’ve got our ingredients ready, let’s whip up a basic Redux Bacon recipe. We’ll start by creating an events map and a middleware:
import { createMiddleware } from 'redux-bacon';
import GoogleAnalytics, { trackPageView } from '@redux-bacon/google-analytics';
const eventsMap = {
'ROUTE_CHANGED': trackPageView(action => ({
page: action.payload.routerState.url,
})),
};
const gaMiddleware = createMiddleware(eventsMap, GoogleAnalytics());
In this example, we’re tracking page views whenever a ROUTE_CHANGED
action is dispatched. The trackPageView
function is an event definition provided by the Google Analytics target.
Sizzling Advanced Techniques
Let’s turn up the heat and explore some more advanced Redux Bacon recipes.
Multiple Events per Action
Sometimes you want to track multiple events for a single action. Redux Bacon makes this easy with the combineEvents
utility:
import { combineEvents } from 'redux-bacon';
const eventsMap = {
'VIDEO_PLAYED': combineEvents(
trackTiming(action => ({
category: 'Videos',
action: 'load',
value: action.payload.loadTime,
})),
trackEvent(() => ({
category: 'Videos',
action: 'play'
})),
),
};
This code will track both the video load time and the play event when a video is played.
Debouncing Events
For actions that might fire rapidly, like search inputs, you can use the debounceEvent
utility to prevent flooding your analytics service:
import { debounceEvent } from 'redux-bacon';
const eventsMap = {
'VIDEO_SEARCHED': debounceEvent(300,
trackEvent(action => ({
category: 'Videos',
action: 'search',
label: action.payload.searchTerm,
}))
),
};
This will ensure that the search event is only sent once every 300 milliseconds, even if the user types quickly.
Custom Event Mapping
If you prefer more control over your event mapping, you can use a function instead of an object:
const eventsMapper = (action) => {
switch(action.type) {
case 'ROUTE_CHANGED':
return trackPageView(action => ({
page: action.payload.routerState.url,
}));
case 'VIDEO_PLAYED':
return [
trackTiming(action => ({
category: 'Videos',
action: 'load',
value: action.payload.loadTime,
})),
trackEvent(() => ({
category: 'Videos',
action: 'play'
})),
];
default:
return [];
}
};
const gaMiddleware = createMiddleware(eventsMapper, GoogleAnalytics());
This approach gives you the flexibility to return different events based on complex conditions or return multiple events for a single action.
Serving Analytics to Multiple Targets
Redux Bacon allows you to send your delicious analytics data to multiple services simultaneously. Simply create multiple middlewares:
import Amplitude from '@redux-bacon/amplitude';
import GoogleAnalytics from '@redux-bacon/google-analytics';
const amplitudeMiddleware = createMiddleware(amplitudeEvents, Amplitude());
const gaMiddleware = createMiddleware(gaEvents, GoogleAnalytics());
// Apply both middlewares to your Redux store
Crafting Your Own Analytics Target
For those with a taste for customization, Redux Bacon lets you create your own analytics target. Here’s a simple recipe:
function myCustomTarget(events) {
events.forEach(event => {
switch (event.type) {
case 'page_view':
window.myAnalyticsSDK.trackPageView(event.page);
break;
case 'custom_event':
window.myAnalyticsSDK.trackEvent(event.category, event.action);
break;
default:
console.log('Unhandled event type:', event.type);
}
});
}
const customMiddleware = createMiddleware(eventsMap, myCustomTarget);
This custom target could integrate with your own analytics service or a third-party SDK not officially supported by Redux Bacon.
Conclusion
Redux Bacon offers a delectable way to enhance your React and Redux applications with powerful analytics capabilities. Its lightweight nature, flexibility, and rich feature set make it an appetizing choice for developers looking to gain deeper insights into user behavior and application state.
By leveraging Redux Bacon, you can effortlessly track page views, user interactions, and state changes, all while keeping your codebase clean and maintainable. Whether you’re using popular analytics services or cooking up your own custom solution, Redux Bacon provides the perfect recipe for success.
So go ahead, sprinkle some Redux Bacon into your next project, and watch as your analytics capabilities sizzle to new heights. Your data-hungry stakeholders will surely come back for seconds!