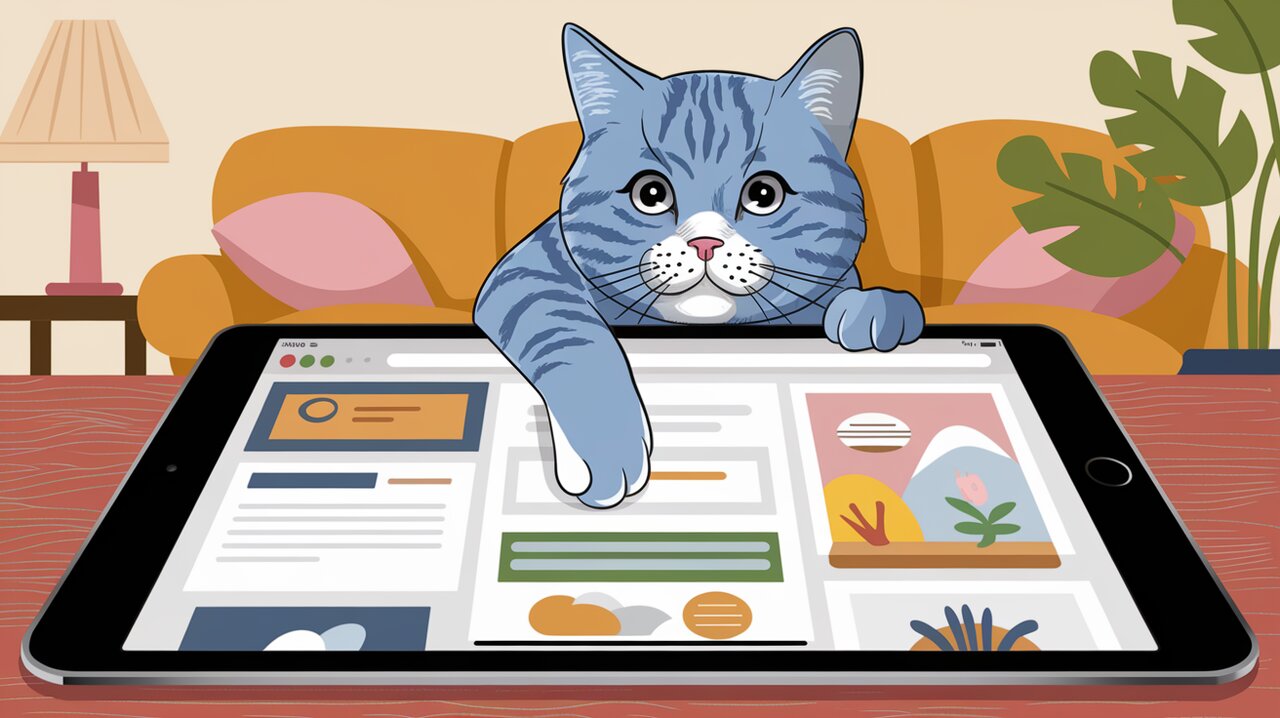
Smooth Scrolling Adventures with react-scroll: Elevate Your React Navigation
Introducing react-scroll: Elevate Your React Navigation
In the world of modern web development, creating smooth and intuitive user experiences is paramount. Enter react-scroll
, a lightweight yet powerful library that brings seamless scrolling animations to your React applications. Whether you’re building a sleek single-page website, a portfolio that wows, or a complex web application, react-scroll
provides an easy-to-use solution for implementing butter-smooth scrolling behavior.
Key Features
react-scroll
comes packed with features that make it a go-to choice for developers:
- Smooth Scrolling: Achieve silky-smooth scrolling animations between sections of your web page.
- Customization: Tailor the scroll behavior to match your application’s design and user experience requirements.
- Accessibility: Ensure your scrolling functionality is accessible to all users, including those relying on assistive technologies.
- Lightweight: Keep your bundle size trim with
react-scroll
’s minimal footprint.
Getting Started with react-scroll
Installation
To begin your smooth scrolling journey, install react-scroll
using npm or yarn:
npm install react-scroll
or
yarn add react-scroll
Basic Usage
Let’s dive into a simple example to demonstrate how easy it is to implement smooth scrolling with react-scroll
:
import React from 'react';
import { Link, Element } from 'react-scroll';
function App() {
return (
<div>
<nav>
<ul>
<li>
<Link to="section1" smooth={true} duration={500}>Section 1</Link>
</li>
<li>
<Link to="section2" smooth={true} duration={500}>Section 2</Link>
</li>
</ul>
</nav>
<Element name="section1">
<section style={{ height: '100vh', backgroundColor: 'lightblue' }}>
<h1>Section 1</h1>
<p>This is the content of section 1</p>
</section>
</Element>
<Element name="section2">
<section style={{ height: '100vh', backgroundColor: 'lightgreen' }}>
<h1>Section 2</h1>
<p>This is the content of section 2</p>
</section>
</Element>
</div>
);
}
export default App;
In this example, we’ve created a simple navigation bar with two links. When clicked, these links smoothly scroll to their respective sections.
Advanced Usage
Customizing Scroll Behavior
react-scroll
offers a variety of props to customize the scrolling experience:
<Link
activeClass="active"
to="target"
spy={true}
smooth={true}
offset={-70}
duration={500}
>
Scroll to Element
</Link>
This configuration adds an active class when the target is reached, enables scrollspy functionality, ensures smooth scrolling, applies an offset, and sets the animation duration.
Scroll Methods
react-scroll
provides several methods for programmatic scrolling:
import { animateScroll as scroll } from 'react-scroll';
// Scroll to top
scroll.scrollToTop();
// Scroll to bottom
scroll.scrollToBottom();
// Scroll to specific position
scroll.scrollTo(100);
// Scroll additional distance
scroll.scrollMore(100);
These methods allow you to control scrolling behavior dynamically within your application.
Handling Scroll Events
You can register and handle scroll events to perform actions during scrolling:
import { Events } from 'react-scroll';
Events.scrollEvent.register('begin', (to, element) => {
console.log('Scroll began', to, element);
});
Events.scrollEvent.register('end', (to, element) => {
console.log('Scroll ended', to, element);
});
// Don't forget to remove events when component unmounts
Events.scrollEvent.remove('begin');
Events.scrollEvent.remove('end');
This feature is particularly useful for triggering animations or loading content as the user scrolls.
Best Practices and Tips
To make the most of react-scroll
, consider these best practices:
- Optimize Performance: Limit the number of elements with scroll events to maintain smooth performance.
- Cross-Device Testing: Ensure your scrolling behavior works well across various devices and screen sizes.
- Customize Animations: Experiment with different easing functions to find the perfect scroll animation for your site.
- Accessibility First: Always consider keyboard navigation and screen reader compatibility when implementing scroll behaviors.
Conclusion
react-scroll
is a powerful tool that can significantly enhance the user experience of your React applications. By providing smooth, customizable scrolling behaviors, it allows you to create more engaging and interactive single-page applications. Whether you’re building a personal portfolio, a corporate website, or a complex web app, react-scroll
offers the flexibility and ease of use to elevate your project’s navigation.
As you embark on your smooth scrolling adventures with react-scroll
, remember that the key to great user experience lies in the details. Experiment with different configurations, listen to user feedback, and continuously refine your scrolling interactions. With react-scroll
in your toolkit, you’re well-equipped to create web experiences that are not just functional, but delightfully smooth and engaging.