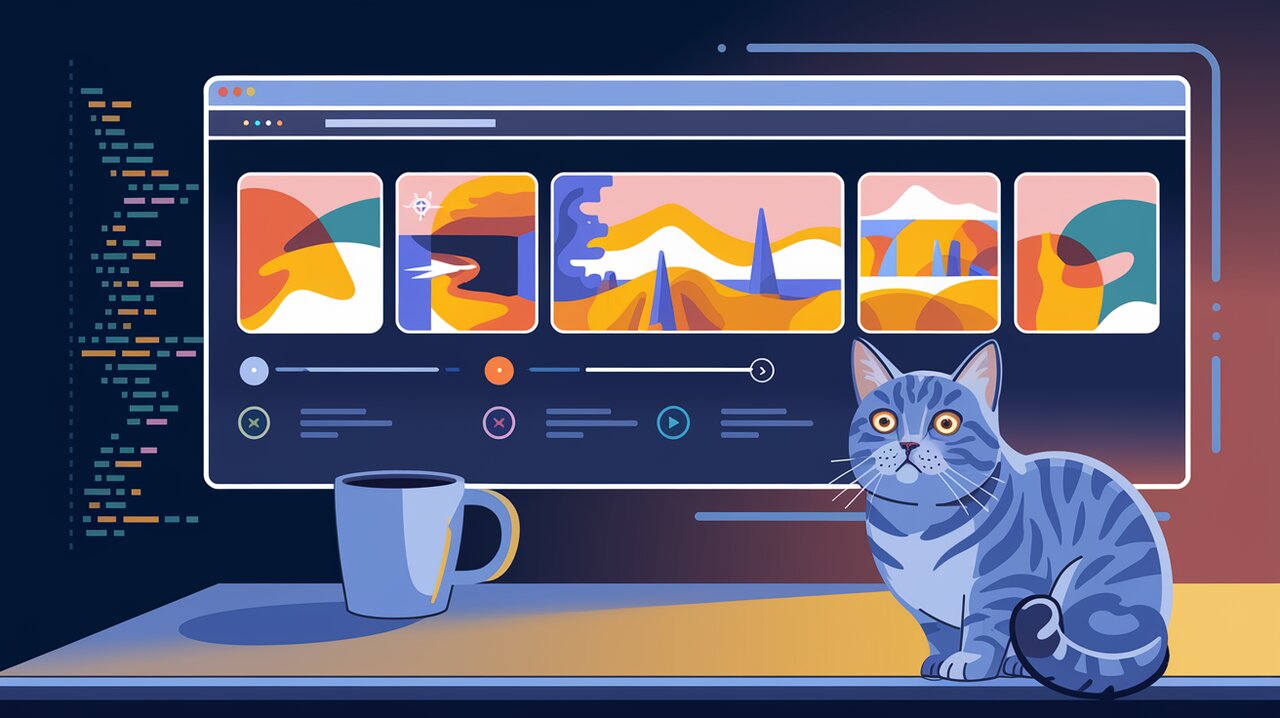
Snapping Into Action: Building Smooth Carousels with React Snap Carousel
React Snap Carousel is a lightweight, DOM-first library that enables developers to create responsive and performant carousels in React applications. By leveraging native browser scrolling and CSS snap points, this library offers a smooth user experience while providing developers with the flexibility to build custom carousel components.
Features
React Snap Carousel boasts several key features that set it apart from other carousel libraries:
- Utilizes native browser scrolling and CSS snap points for optimal performance
- Computes responsive page state based on DOM layout and scroll position
- Dynamically renders page-based CSS snap points
- Provides a headless design with a React Hooks API for full UI control
- Written in TypeScript for improved type safety
- Lightweight (approximately 1KB) with zero dependencies
Installation
To get started with React Snap Carousel, you can install it using npm or yarn:
npm install react-snap-carousel
or
yarn add react-snap-carousel
Basic Usage
Let’s create a simple carousel component using React Snap Carousel. We’ll start by importing the necessary hooks and setting up the basic structure.
Creating a Basic Carousel
import React from 'react';
import { useSnapCarousel } from 'react-snap-carousel';
const Carousel: React.FC = () => {
const { scrollRef, pages, activePageIndex, next, prev } = useSnapCarousel();
return (
<div>
<ul ref={scrollRef} style={{ display: 'flex', overflow: 'auto', scrollSnapType: 'x mandatory' }}>
{[1, 2, 3, 4, 5].map((item) => (
<li key={item} style={{ flexShrink: 0, width: '100%', scrollSnapAlign: 'start' }}>
<img src={`https://picsum.photos/id/${item}/400/300`} alt={`Item ${item}`} />
</li>
))}
</ul>
<button onClick={() => prev()}>Previous</button>
<button onClick={() => next()}>Next</button>
<p>Page {activePageIndex + 1} of {pages.length}</p>
</div>
);
};
In this example, we’re using the useSnapCarousel
hook to manage the carousel state. The scrollRef
is attached to the scrollable container, while pages
, activePageIndex
, next
, and prev
are used to control navigation and display the current page information.
Advanced Usage
Now let’s explore some more advanced features of React Snap Carousel to create a more customized and interactive carousel component.
Custom Snap Points
React Snap Carousel allows you to define custom snap points for your carousel items. This is particularly useful when you want to create a carousel with items of varying widths.
import React from 'react';
import { useSnapCarousel } from 'react-snap-carousel';
const CustomSnapCarousel: React.FC = () => {
const { scrollRef, snapPointIndexes, pages, activePageIndex, next, prev } = useSnapCarousel();
return (
<div>
<ul ref={scrollRef} style={{ display: 'flex', overflow: 'auto', scrollSnapType: 'x mandatory' }}>
{[1, 2, 3, 4, 5].map((item, index) => (
<li
key={item}
style={{
flexShrink: 0,
width: index % 2 === 0 ? '60%' : '40%',
scrollSnapAlign: snapPointIndexes.has(index) ? 'start' : ''
}}
>
<img src={`https://picsum.photos/id/${item}/400/300`} alt={`Item ${item}`} />
</li>
))}
</ul>
<button onClick={() => prev()}>Previous</button>
<button onClick={() => next()}>Next</button>
<p>Page {activePageIndex + 1} of {pages.length}</p>
</div>
);
};
In this example, we’re using the snapPointIndexes
to determine which items should be snap points. This allows us to create a carousel with alternating item widths while maintaining smooth scrolling behavior.
Responsive Carousel
React Snap Carousel automatically adjusts to changes in the viewport size. Let’s create a responsive carousel that changes the number of visible items based on the screen width.
import React, { useState, useEffect } from 'react';
import { useSnapCarousel } from 'react-snap-carousel';
const ResponsiveCarousel: React.FC = () => {
const [itemWidth, setItemWidth] = useState('100%');
const { scrollRef, pages, activePageIndex, next, prev } = useSnapCarousel();
useEffect(() => {
const handleResize = () => {
if (window.innerWidth >= 1024) {
setItemWidth('33.33%');
} else if (window.innerWidth >= 768) {
setItemWidth('50%');
} else {
setItemWidth('100%');
}
};
handleResize();
window.addEventListener('resize', handleResize);
return () => window.removeEventListener('resize', handleResize);
}, []);
return (
<div>
<ul ref={scrollRef} style={{ display: 'flex', overflow: 'auto', scrollSnapType: 'x mandatory' }}>
{[1, 2, 3, 4, 5, 6].map((item) => (
<li key={item} style={{ flexShrink: 0, width: itemWidth, scrollSnapAlign: 'start' }}>
<img src={`https://picsum.photos/id/${item}/400/300`} alt={`Item ${item}`} />
</li>
))}
</ul>
<button onClick={() => prev()}>Previous</button>
<button onClick={() => next()}>Next</button>
<p>Page {activePageIndex + 1} of {pages.length}</p>
</div>
);
};
This responsive carousel adjusts the number of visible items based on the screen width, providing a better user experience across different devices.
Conclusion
React Snap Carousel offers a powerful and flexible solution for creating carousels in React applications. By leveraging native browser scrolling and CSS snap points, it provides smooth performance while giving developers full control over the UI.
We’ve explored the basic usage of the library, as well as some advanced techniques for creating custom and responsive carousels. With its lightweight footprint and zero dependencies, React Snap Carousel is an excellent choice for developers looking to implement efficient and customizable carousel components in their React projects.
As you continue to work with React Snap Carousel, remember to explore its documentation for more advanced features and optimizations. Happy carousel building!