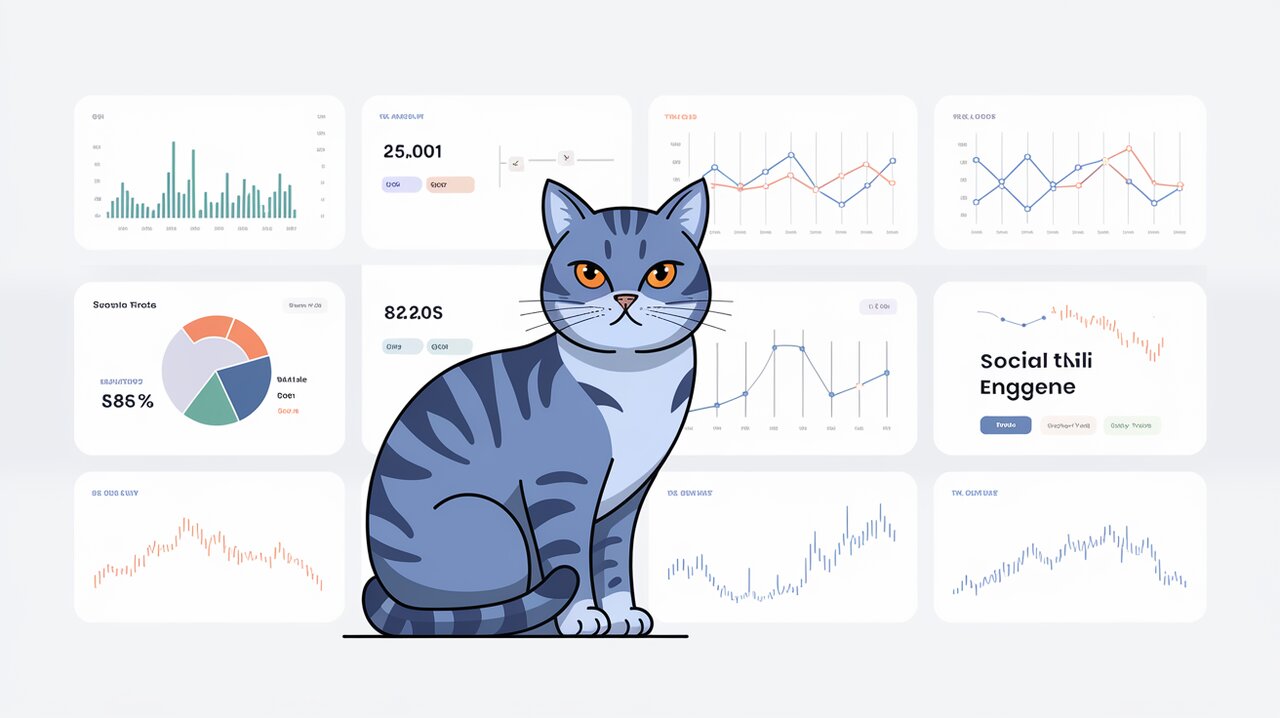
Sparkline Symphony: Orchestrating Data with React-Sparklines
React-Sparklines is a powerful and expressive library that brings the beauty of sparkline charts to your React applications. These small, high-density graphics provide a simple and elegant way to display trends in data, making them perfect for dashboards, reports, and anywhere you need to convey information at a glance.
Igniting the Spark: Installation and Basic Usage
To begin our sparkline symphony, let’s start by installing the library:
npm install react-sparklines --save
Or if you prefer yarn:
yarn add react-sparklines
With the library installed, we can create our first sparkline chart. Here’s a basic example:
import React from 'react';
import { Sparklines, SparklinesLine } from 'react-sparklines';
const SimpleSparkline: React.FC = () => {
const data = [5, 10, 5, 20, 8, 15];
return (
<Sparklines data={data} width={100} height={20} margin={5}>
<SparklinesLine color="blue" />
</Sparklines>
);
};
export default SimpleSparkline;
This code snippet creates a simple blue line sparkline chart. The Sparklines
component acts as a container, while SparklinesLine
renders the actual line chart.
Composing the Chart: Key Components
React-Sparklines offers several components to customize your charts:
SparklinesLine
The SparklinesLine
component is the most basic and commonly used. It creates a simple line chart:
<Sparklines data={[5, 10, 5, 20, 8, 15]}>
<SparklinesLine color="blue" />
</Sparklines>
SparklinesBars
For bar chart enthusiasts, SparklinesBars
creates a bar representation of your data:
<Sparklines data={[5, 10, 5, 20, 8, 15]}>
<SparklinesBars />
</Sparklines>
SparklinesSpots
To highlight specific points in your data, use SparklinesSpots
:
<Sparklines data={[5, 10, 5, 20, 8, 15]}>
<SparklinesLine style={{ fill: "none" }} />
<SparklinesSpots />
</Sparklines>
SparklinesReferenceLine
Add context to your charts with reference lines:
<Sparklines data={[5, 10, 5, 20, 8, 15]}>
<SparklinesLine />
<SparklinesReferenceLine type="mean" />
</Sparklines>
Advanced Techniques: Customization and Styling
React-Sparklines allows for extensive customization to fit your specific needs.
Limiting Data Points
You can control the number of data points displayed using the limit
prop:
<Sparklines data={[5, 10, 5, 20, 8, 15]} limit={5}>
<SparklinesLine />
</Sparklines>
Custom Dimensions
Adjust the size of your sparkline with width
and height
props:
<Sparklines data={[5, 10, 5, 20, 8, 15]} width={200} height={50}>
<SparklinesLine />
</Sparklines>
Styling Components
Each component accepts style props for fine-grained control:
<Sparklines data={[5, 10, 5, 20, 8, 15]}>
<SparklinesLine style={{ strokeWidth: 3, stroke: "#336aff" }} />
</Sparklines>
Orchestrating Complex Visualizations
For more advanced use cases, you can combine multiple components to create rich visualizations:
import React from 'react';
import { Sparklines, SparklinesLine, SparklinesSpots, SparklinesReferenceLine, SparklinesNormalBand } from 'react-sparklines';
const AdvancedSparkline: React.FC = () => {
const data = [5, 10, 5, 20, 8, 15, 30, 25, 22, 18, 28];
return (
<Sparklines data={data} width={200} height={60} margin={5}>
<SparklinesLine style={{ stroke: "#2991c8", fill: "none" }} />
<SparklinesSpots size={4} style={{ stroke: "#2991c8", fill: "white" }} />
<SparklinesReferenceLine type="mean" style={{ stroke: 'green', strokeOpacity: 0.75, strokeDasharray: '2, 2' }} />
<SparklinesNormalBand />
</Sparklines>
);
};
export default AdvancedSparkline;
This example combines a line chart with highlighted spots, a mean reference line, and a normal band to show data distribution.
Conclusion: The Power of Compact Visualization
React-Sparklines offers a symphony of possibilities for data visualization in your React applications. From simple line charts to complex, multi-component visualizations, this library provides the tools you need to create informative and visually appealing sparklines.
By leveraging the power of React-Sparklines, you can enhance your dashboards, reports, and data-driven components with compact, yet powerful charts that convey trends and insights at a glance.
As you continue to explore the world of data visualization in React, you might also be interested in other charting libraries. Check out our articles on Recharts for more comprehensive charting options, or React-ChartJS-2 for another popular charting solution in the React ecosystem.
Remember, the key to effective data visualization is choosing the right tool for the job. React-Sparklines excels at providing quick, compact representations of data trends. Use it wisely, and let your data sing!