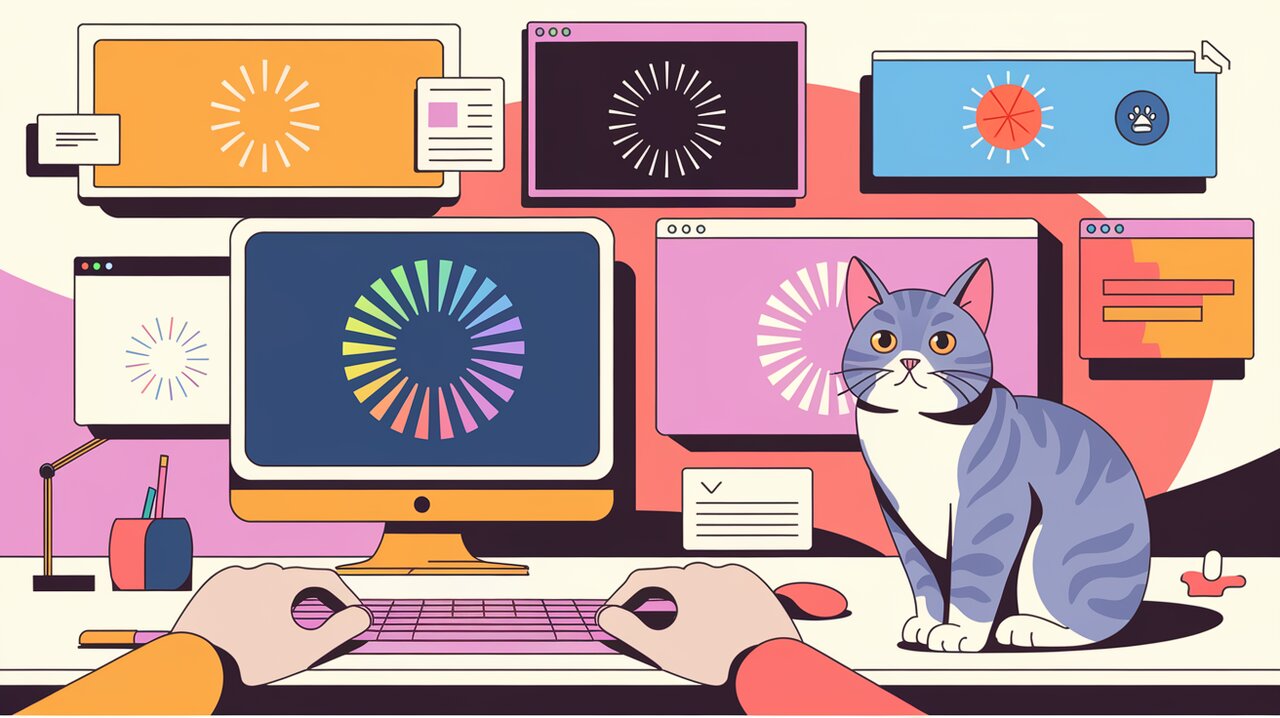
Spinning Up Your React App: The Whimsical World of react-spinners-css
React developers often find themselves in a spin when it comes to creating engaging loading indicators. Enter react-spinners-css, a whimsical library that brings a touch of magic to your applications with its collection of pure CSS spinners. Let’s dive into this zero-dependency wonder and discover how it can transform your loading screens from mundane to marvelous.
Spinning Features That’ll Make Your Head Turn
react-spinners-css isn’t just another run-of-the-mill spinner library. It’s a treasure trove of delightful animations that will keep your users mesmerized while your content loads. Here’s what makes it stand out:
- Pure CSS magic: No extra CSS imports needed
- Zero dependencies: Lightweight and efficient
- Customizable components: Tailor spinners to your design
- Individual imports: Use only what you need
- TypeScript support: For type-safe development
Installing the Carousel of Spinners
Getting started with react-spinners-css is as easy as pie. You can install it using your favorite package manager:
npm install react-spinners-css
Or if you prefer yarn:
yarn add react-spinners-css
Basic Usage: Let the Spinning Begin
Once installed, you can start using the spinners right away. Here’s a simple example to get you spinning:
import React from 'react';
import { Circle } from 'react-spinners-css';
const LoadingComponent: React.FC = () => {
return (
<div>
<h2>Loading...</h2>
<Circle />
</div>
);
};
export default LoadingComponent;
This code will display a default circle spinner with a lovely purple hue. But why stop there when you can customize it?
Customizing Your Spinning Spectacle
Colorful Twirls
Want to match your spinner to your brand colors? No problem! You can easily change the color of any spinner:
import React from 'react';
import { Heart } from 'react-spinners-css';
const CustomColorSpinner: React.FC = () => {
return <Heart color="#ff6b6b" />;
};
export default CustomColorSpinner;
Size Matters
Sometimes you need a spinner that’s larger than life, or perhaps one that’s more subtle. react-spinners-css has you covered:
import React from 'react';
import { Default } from 'react-spinners-css';
const BigSpinner: React.FC = () => {
return <Default size={150} />;
};
export default BigSpinner;
Advanced Spinning Techniques
The Spinner Ensemble
Why settle for one spinner when you can have a whole performance? Let’s create a loading screen with multiple spinners:
import React from 'react';
import { Circle, Ellipsis, Grid } from 'react-spinners-css';
const SpinnerEnsemble: React.FC = () => {
return (
<div style={{ display: 'flex', justifyContent: 'space-around' }}>
<Circle color="#3498db" />
<Ellipsis color="#e74c3c" />
<Grid color="#2ecc71" />
</div>
);
};
export default SpinnerEnsemble;
Conditional Spinning
In real-world applications, you’ll want to show spinners conditionally. Here’s how you can toggle spinners based on a loading state:
import React, { useState, useEffect } from 'react';
import { DualRing } from 'react-spinners-css';
const ConditionalSpinner: React.FC = () => {
const [isLoading, setIsLoading] = useState(true);
useEffect(() => {
// Simulate an API call
setTimeout(() => {
setIsLoading(false);
}, 3000);
}, []);
return (
<div>
{isLoading ? (
<DualRing />
) : (
<p>Content loaded successfully!</p>
)}
</div>
);
};
export default ConditionalSpinner;
Spinning in Harmony with Other React Libraries
While react-spinners-css is fantastic on its own, it plays well with others too. For instance, you could combine it with a state management library like Redux to handle global loading states. If you’re interested in exploring more React libraries, check out our articles on Redux-cycles for reactive side effects or React-beautiful-dnd for drag-and-drop functionality.
Conclusion: Keep on Spinning
react-spinners-css offers a delightful way to add flair to your loading screens without the bloat of additional dependencies. Its pure CSS approach ensures smooth performance, while the variety of spinners gives you the creative freedom to match your application’s style.
Remember, a well-designed loading indicator can make the difference between a user patiently waiting or bouncing from your site. So why not give your users something mesmerizing to look at while your content loads?
As you continue to explore the world of React libraries, keep react-spinners-css in your toolkit for those moments when you need to add a touch of whimsy to your loading states. Happy spinning!