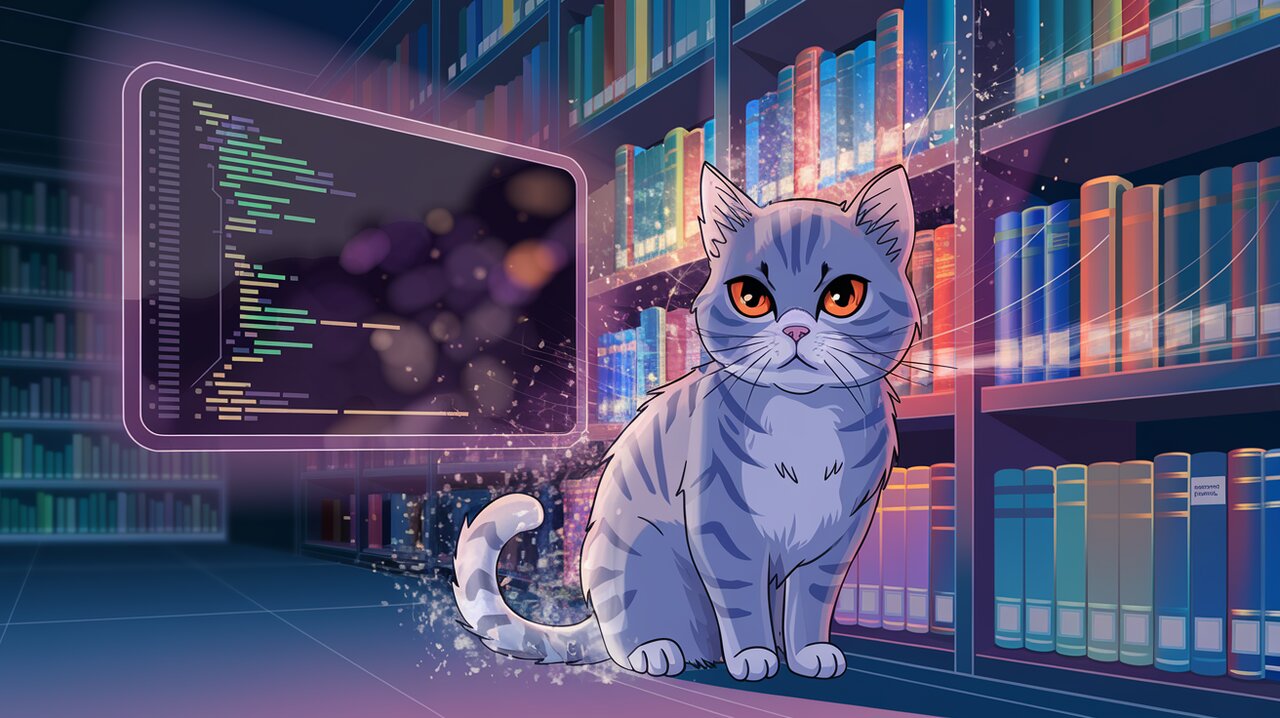
Spoiled Secrets: Unveiling React's Animated Spoiler Magic
Spoiled is a React library that brings the magic of Telegram-style spoilers to your web applications. It allows you to create animated particle clouds that cover text or elements, keeping them hidden until revealed. This library offers a unique way to add intrigue and interactivity to your content, perfect for hiding spoilers, surprises, or sensitive information.
Unveiling the Features
Spoiled comes packed with a range of features that make it stand out:
- Utilizes the CSS Painting API (Houdini) for realistic rendering of inline elements
- Provides a static image fallback for browsers that don’t support the CSS Painting API
- Supports light, dark, and system color modes
- Offers animated content transitions (fade/iris) with customization options
- Respects the user’s
prefers-reduced-motion
setting - Allows fine-tuning of performance through FPS and density controls
Setting Up the Spoiler Stage
To begin your journey with spoiled, you’ll need to install it in your React project. The library requires React 18 or higher.
Using npm:
npm install spoiled
Or if you prefer yarn:
yarn add spoiled
Basic Incantations: Using Spoiled
Once installed, you can start wrapping your content in spoilers. Let’s look at some basic usage examples.
The Simple Spell
Here’s how you can create a basic spoiler that reveals on hover:
import { Spoiler } from "spoiled";
function MyComponent() {
return (
<Spoiler>
Hogwarts is actually a high-tech <b>startup incubator</b>
</Spoiler>
);
}
This code creates a spoiler that wraps the text in a span
element and reveals the content when the user hovers over it.
Customizing the Enchantment
Spoiled allows you to customize various aspects of the spoiler. Here’s an example that changes the reveal trigger and adds some accessibility:
import { Spoiler } from "spoiled";
function SecretReveal() {
return (
<Spoiler revealOn="click" className="custom-spoiler" aria-label="Click to reveal secret">
Neo actually opens a digital wellness retreat in the Matrix
</Spoiler>
);
}
In this example, the spoiler will reveal its content on click rather than hover. We’ve also added a custom class and an aria-label for better accessibility.
Advanced Sorcery: Mastering Spoiled
Now that we’ve covered the basics, let’s delve into some more advanced uses of spoiled.
Controlled Revelations
For more complex scenarios, you might want to control the spoiler’s state from your component:
import React, { useState } from 'react';
import { Spoiler } from "spoiled";
function ControlledSpoiler() {
const [isHidden, setIsHidden] = useState(true);
return (
<div>
<button onClick={() => setIsHidden(!isHidden)}>
{isHidden ? "Reveal" : "Hide"} Secret
</button>
<Spoiler hidden={isHidden} onClick={() => setIsHidden(!isHidden)}>
Frodo's ring business becomes a billion-dollar startup
</Spoiler>
</div>
);
}
This example demonstrates a controlled spoiler where the hidden state is managed by the parent component, allowing for more complex interactions.
Theming the Mystery
Spoiled adapts to your app’s color scheme, but you can also customize its appearance:
import { Spoiler } from "spoiled";
function ThemedSpoilers() {
return (
<div>
<Spoiler theme="dark" accentColor="red">
Dark themed spoiler with red accent
</Spoiler>
<Spoiler theme="light" accentColor={["#000", "#fff"]}>
Light themed spoiler with dynamic accent
</Spoiler>
</div>
);
}
Here, we’ve created two spoilers with different themes and accent colors. The second spoiler uses an array for the accent color, allowing it to change based on the current theme.
Performance Tuning
For optimal performance, especially when dealing with many spoilers, you can adjust the FPS and density:
import { Spoiler } from "spoiled";
function PerformanceOptimizedSpoiler() {
return (
<Spoiler fps={16} density={0.1}>
This spoiler is optimized for performance
</Spoiler>
);
}
This example creates a spoiler with a lower frame rate and particle density, which can be beneficial for performance on less powerful devices.
Shaping Words
By default, spoiled tries to mimic the shape of words in a paragraph. However, you can disable this feature:
import { Spoiler } from "spoiled";
function SolidLineSpoiler() {
return (
<Spoiler mimicWords={false}>
This text will be covered by a solid line of particles
</Spoiler>
);
}
This creates a spoiler where the particle cloud forms a solid line rather than attempting to mimic word shapes.
Wrapping Up the Secrets
Spoiled offers a unique and engaging way to hide and reveal content in your React applications. From simple text spoilers to complex, themed, and performance-optimized implementations, this library provides a wide range of options for creating interactive and visually appealing content reveals.
Remember that while spoiled uses cutting-edge CSS features, it also provides fallbacks for browsers that don’t support them. This ensures that your spoilers will work across a wide range of devices and browsers, making it a versatile choice for adding a touch of mystery and interactivity to your React projects.
Whether you’re building a blog with spoiler-protected content, a quiz app with hidden answers, or just want to add an element of surprise to your UI, spoiled provides the tools you need to create captivating and functional spoilers in your React applications.