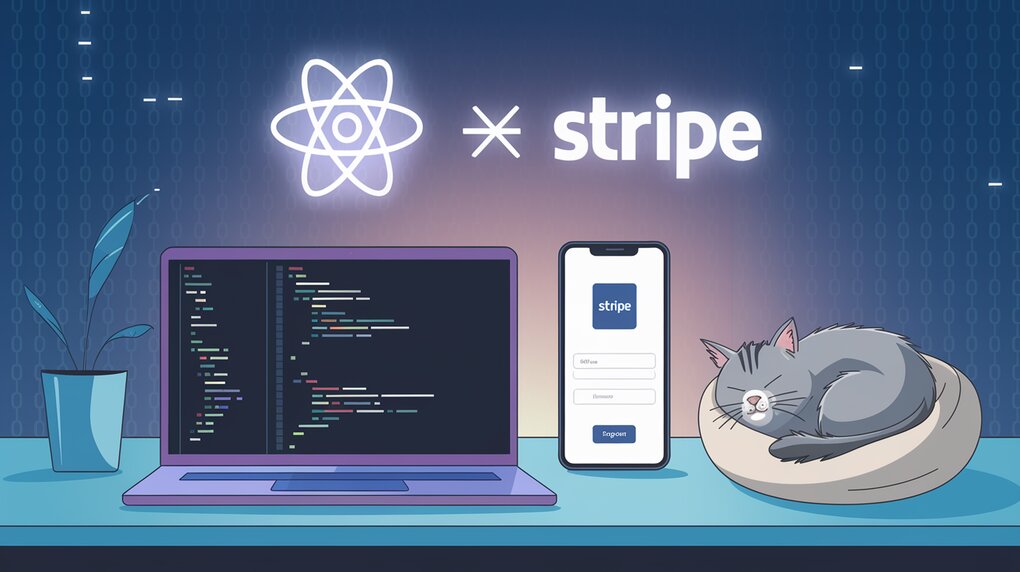
Supercharge Your React App with Stripe Payments: A Deep Dive into react-stripe-elements
React Stripe Elements is a powerful library that simplifies the integration of Stripe payments into your React applications. By providing a set of React components that wrap Stripe.js and Stripe Elements, it allows developers to easily create customizable and secure payment forms.
Features
- Seamless integration with React applications
- Pre-built UI components for common payment fields
- Support for various payment methods, including credit cards and digital wallets
- Easy customization of payment form styles
- Automatic handling of sensitive payment data
Installation
To get started with react-stripe-elements, you need to install the package in your React project. You can do this using npm or yarn.
Using npm:
npm install --save react-stripe-elements
Using yarn:
yarn add react-stripe-elements
After installation, you need to include the Stripe.js script in your HTML file:
<script src="https://js.stripe.com/v3/"></script>
Basic Usage
To use react-stripe-elements in your application, you need to set up the Stripe context and wrap your payment form with the necessary components.
Setting up the Stripe Context
First, wrap your root component with the StripeProvider
component:
import React from 'react';
import {StripeProvider} from 'react-stripe-elements';
const App = () => {
return (
<StripeProvider apiKey="your_stripe_public_key">
{/* Your app components */}
</StripeProvider>
);
};
export default App;
Creating a Payment Form
Next, create a payment form component using the Elements
wrapper and individual Stripe Element components:
import React from 'react';
import {Elements, CardElement, injectStripe} from 'react-stripe-elements';
const CheckoutForm = ({stripe}) => {
const handleSubmit = async (event) => {
event.preventDefault();
const {token} = await stripe.createToken();
// Send the token to your server for processing
};
return (
<form onSubmit={handleSubmit}>
<CardElement />
<button type="submit">Pay</button>
</form>
);
};
const InjectedCheckoutForm = injectStripe(CheckoutForm);
const PaymentForm = () => {
return (
<Elements>
<InjectedCheckoutForm />
</Elements>
);
};
export default PaymentForm;
In this example, we use the CardElement
component to render a complete credit card input field. The injectStripe
higher-order component provides the stripe
prop, which gives access to Stripe.js functions.
Advanced Usage
React Stripe Elements offers more advanced features for complex payment scenarios.
Using Individual Elements
Instead of using the all-in-one CardElement
, you can use individual elements for more granular control:
import React from 'react';
import {CardNumberElement, CardExpiryElement, CardCVCElement} from 'react-stripe-elements';
const CustomForm = () => {
return (
<div>
<CardNumberElement />
<CardExpiryElement />
<CardCVCElement />
</div>
);
};
Handling Payment Intents
For more complex payment flows, you can use Payment Intents:
const handlePayment = async () => {
const {paymentMethod, error} = await stripe.createPaymentMethod('card', cardElement);
if (error) {
console.error(error);
} else {
const response = await fetch('/create-payment-intent', {
method: 'POST',
headers: {'Content-Type': 'application/json'},
body: JSON.stringify({payment_method_id: paymentMethod.id}),
});
const {clientSecret} = await response.json();
const result = await stripe.confirmCardPayment(clientSecret);
if (result.error) {
console.error(result.error);
} else {
// Payment successful
}
}
};
Customizing Element Styles
You can customize the appearance of Stripe Elements to match your application’s design:
const elementStyles = {
base: {
fontSize: '16px',
color: '#424770',
'::placeholder': {
color: '#aab7c4',
},
},
invalid: {
color: '#9e2146',
},
};
<CardElement style={elementStyles} />
Conclusion
React Stripe Elements provides a powerful and flexible way to integrate Stripe payments into your React applications. By offering pre-built components and easy customization options, it simplifies the process of creating secure and user-friendly payment forms. Whether you’re building a simple checkout or a complex payment system, react-stripe-elements has the tools you need to create a seamless payment experience for your users.
Remember to always handle sensitive payment data securely and comply with PCI DSS requirements when processing payments. With react-stripe-elements, you can focus on building great user experiences while leaving the complexities of payment processing to Stripe.