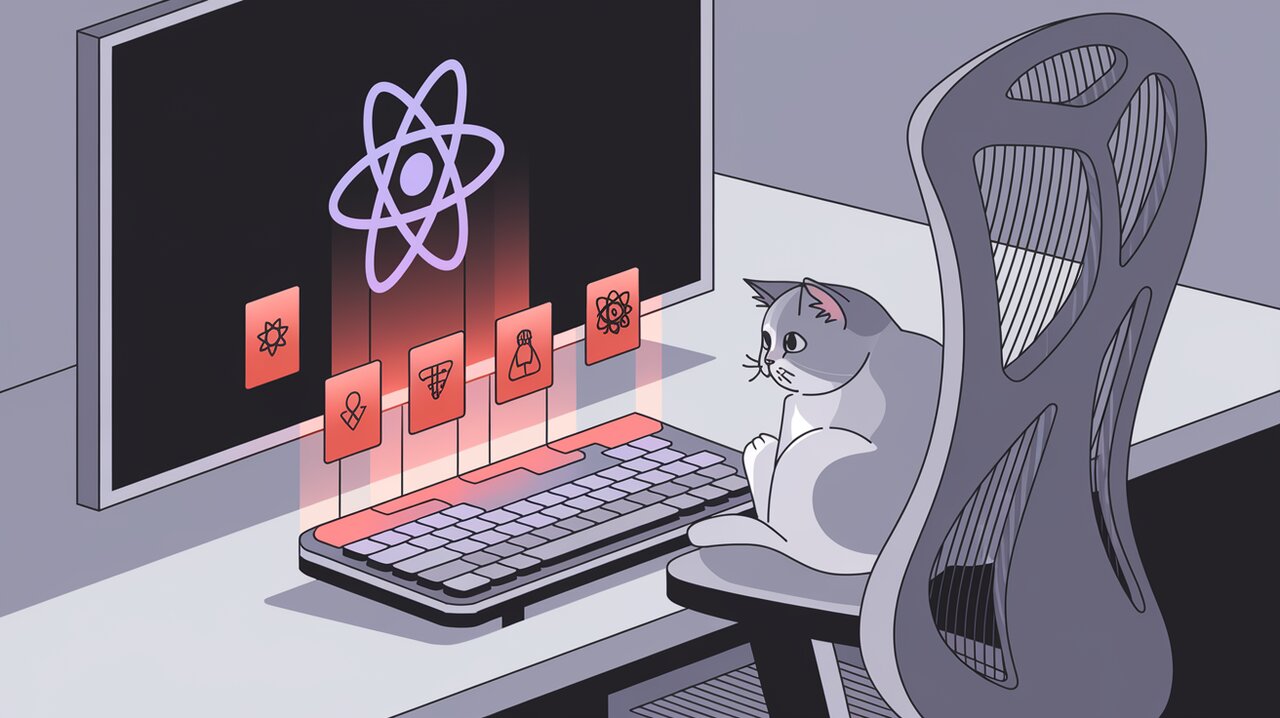
Supercharge Your React Apps with React Hotkeys Hook
Introduction
In the ever-evolving landscape of web development, creating intuitive and efficient user interfaces is crucial. One powerful way to enhance user experience is through the implementation of keyboard shortcuts. Enter react-hotkeys-hook, a lightweight and flexible library that brings the power of keyboard shortcuts to your React applications with remarkable ease and efficiency.
Features
react-hotkeys-hook
offers a range of features that make it an excellent choice for implementing keyboard shortcuts in your React projects:
- Declarative API: Define hotkeys using a simple, intuitive syntax.
- Scoped Hotkeys: Group and manage hotkeys to prevent conflicts.
- Customizable Options: Fine-tune behavior with various configuration options.
- TypeScript Support: Enjoy full type safety and autocompletion.
- Focus Trapping: Limit hotkey functionality to specific components.
- Conditional Activation: Enable or disable hotkeys based on custom logic.
Installation
Getting started with react-hotkeys-hook
is straightforward. You can install it using npm or yarn:
npm install react-hotkeys-hook
or
yarn add react-hotkeys-hook
Basic Usage
Let’s dive into some basic examples to demonstrate how easy it is to use react-hotkeys-hook in your React components.
Simple Hotkey
Here’s a simple example of how to increment a counter using the ‘Ctrl+K’ hotkey:
import React, { useState } from 'react';
import { useHotkeys } from 'react-hotkeys-hook';
const SimpleCounter = () => {
const [count, setCount] = useState(0);
useHotkeys('ctrl+k', () => setCount(prevCount => prevCount + 1));
return <div>Counter: {count}</div>;
};
In this example, we import the useHotkeys
hook from the library. We then use it to listen for the ‘Ctrl+K’ key combination, which increments our counter when pressed. The hook takes two main arguments: the key combination as a string and a callback function to execute when the keys are pressed.
Multiple Hotkeys
You can also define multiple hotkeys for the same action:
import React, { useState } from 'react';
import { useHotkeys } from 'react-hotkeys-hook';
const MultipleHotkeys = () => {
const [message, setMessage] = useState('Press a hotkey');
useHotkeys(['ctrl+s', 'meta+s'], (event) => {
event.preventDefault();
setMessage('Saved!');
});
return <div>{message}</div>;
};
This component listens for both ‘Ctrl+S’ and ‘Meta+S’ (Command+S on Mac) to trigger the same action. We also prevent the default browser behavior using event.preventDefault()
. By passing an array of key combinations, we can easily handle multiple shortcuts with a single hook call.
Advanced Usage
Now that we’ve covered the basics, let’s explore some more advanced features of react-hotkeys-hook.
Scoped Hotkeys
Scopes allow you to group hotkeys together and prevent conflicts between different parts of your application:
import React from 'react';
import { useHotkeys } from 'react-hotkeys-hook';
import { HotkeysProvider, useHotkeysContext } from 'react-hotkeys-hook';
const ScopedComponent = () => {
const { enableScope, disableScope } = useHotkeysContext();
useHotkeys('ctrl+s', () => console.log('Saved in scope'), { scopes: ['editor'] });
return (
<div>
<button onClick={() => enableScope('editor')}>Enable Editor Hotkeys</button>
<button onClick={() => disableScope('editor')}>Disable Editor Hotkeys</button>
</div>
);
};
const App = () => (
<HotkeysProvider initiallyActiveScopes={['editor']}>
<ScopedComponent />
</HotkeysProvider>
);
In this example, we use the HotkeysProvider
to set up scopes and the useHotkeysContext
hook to manage them. The ‘Ctrl+S’ hotkey will only work when the ‘editor’ scope is active. This is particularly useful in complex applications where different sections might use the same key combinations for different purposes.
Focus Trapping
You can limit hotkeys to specific elements using the focus trap feature:
import React, { useState } from 'react';
import { useHotkeys } from 'react-hotkeys-hook';
const FocusTrapExample = () => {
const [count, setCount] = useState(0);
const ref = useHotkeys<HTMLDivElement>('ctrl+k', () => setCount(prevCount => prevCount + 1));
return (
<div ref={ref} tabIndex={-1}>
<p>Focus this div and press Ctrl+K</p>
<p>Count: {count}</p>
</div>
);
};
By using the ref
returned from useHotkeys
, we ensure that the hotkey only works when the div is focused. This is particularly useful for creating modal dialogs or other focused interactions within your application.
Conditional Hotkeys
You can enable or disable hotkeys based on certain conditions:
import React, { useState } from 'react';
import { useHotkeys } from 'react-hotkeys-hook';
const ConditionalHotkey = () => {
const [enabled, setEnabled] = useState(true);
const [count, setCount] = useState(0);
useHotkeys('ctrl+k', () => setCount(prevCount => prevCount + 1), {
enabled,
enableOnFormTags: ['INPUT', 'TEXTAREA'],
});
return (
<div>
<button onClick={() => setEnabled(!enabled)}>
{enabled ? 'Disable' : 'Enable'} Hotkey
</button>
<p>Count: {count}</p>
<input type="text" placeholder="Hotkey works here too" />
</div>
);
};
This example demonstrates how to toggle a hotkey’s functionality and enable it on form elements. The enabled
option allows you to dynamically control whether the hotkey is active, while enableOnFormTags
specifies which form elements should respond to the hotkey.
Custom Key Combinations
react-hotkeys-hook supports a wide range of key combinations:
import React from 'react';
import { useHotkeys } from 'react-hotkeys-hook';
const CustomCombinations = () => {
useHotkeys('shift+a', () => console.log('Shift+A pressed'));
useHotkeys('ctrl+alt+delete', () => console.log('Ctrl+Alt+Delete pressed'));
useHotkeys('command+k,command+m', () => console.log('Command+K then Command+M pressed'));
return <div>Check the console for hotkey presses</div>;
};
This example shows how you can use modifier keys, multiple key presses, and even sequences of key combinations. The library supports a wide range of key names and modifiers, making it flexible for various use cases.
Best Practices and Considerations
When implementing keyboard shortcuts in your React applications using react-hotkeys-hook, keep these best practices in mind:
-
Accessibility: Always provide visual cues or alternative methods for users who may not be able to use keyboard shortcuts.
-
Consistency: Try to use standard shortcuts where possible (e.g., Ctrl+S for save) to maintain consistency with user expectations.
-
Documentation: Provide a list of available shortcuts in your application, perhaps in a help menu or settings page.
-
Performance: Be mindful of the number of hotkeys you’re registering, especially in large applications. Use scopes to manage and organize your shortcuts effectively.
-
Testing: Implement unit and integration tests for your hotkey functionality to ensure they work as expected across different scenarios.
Conclusion
react-hotkeys-hook
provides a powerful and flexible way to implement keyboard shortcuts in your React applications. By leveraging its declarative API and advanced features like scoping and focus trapping, you can create more intuitive and efficient user interfaces.
From simple key presses to complex combinations and conditional activations, this library offers a comprehensive solution for managing keyboard interactions in React. As you integrate these shortcuts into your applications, remember to consider accessibility, consistency, and user experience to create truly polished and professional interfaces.
With react-hotkeys-hook in your toolkit, you’re well-equipped to enhance your React applications and provide a superior, keyboard-friendly user experience that will delight both power users and those seeking efficient navigation options.