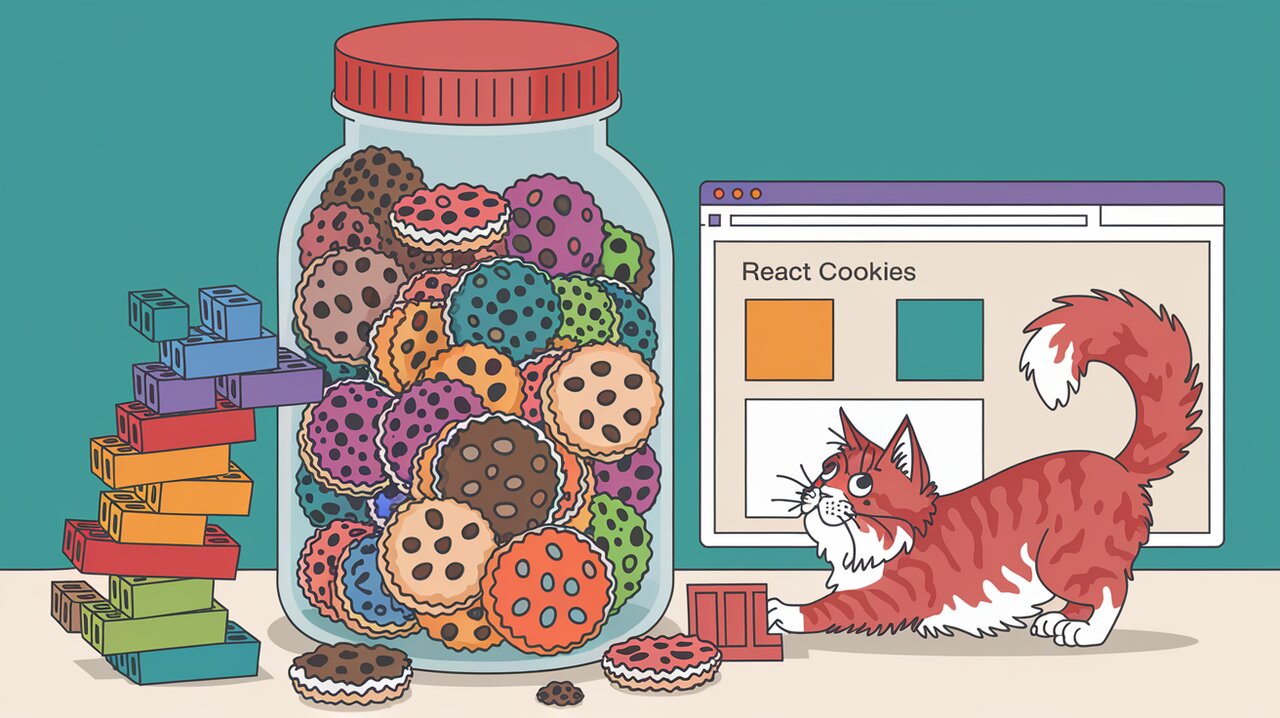
Sweet Tooth for React: Mastering Cookies with react-cookie
In the world of web development, managing user data efficiently is crucial for creating responsive and personalized experiences. Enter react-cookie
, a powerful library that brings the sweetness of cookie management to your React applications. Whether you’re handling user preferences, maintaining authentication states, or simply need to store small pieces of information client-side, react-cookie
provides a delicious solution that’s easy to implement and works seamlessly across both client and server environments.
Features
- Universal cookie handling for both client and server-side rendering
- Simple API for getting, setting, and removing cookies
- React hooks for easy integration with functional components
- Support for cookie options like expiration, domain, and security settings
- TypeScript support for enhanced development experience
Installation
Getting started with react-cookie
is as easy as adding your favorite cookie to your shopping cart. You can install it using npm or yarn:
Using npm:
npm install react-cookie
Using yarn:
yarn add react-cookie
Basic Usage
Setting Up the CookiesProvider
Before you can start munching on cookies in your React app, you need to set up the CookiesProvider
. This component should wrap your entire application to ensure that all child components have access to the cookie functionality.
import React from 'react';
import { CookiesProvider } from 'react-cookie';
import App from './App';
const Root: React.FC = () => {
return (
<CookiesProvider>
<App />
</CookiesProvider>
);
};
export default Root;
The CookiesProvider
acts as the cookie jar for your application, allowing all nested components to access and modify cookies through the provided hooks and HOCs.
Using the useCookies Hook
Now that we’ve set up our cookie jar, let’s grab some cookies! The useCookies
hook is the simplest way to interact with cookies in your functional components.
import React from 'react';
import { useCookies } from 'react-cookie';
const UserPreferences: React.FC = () => {
const [cookies, setCookie, removeCookie] = useCookies(['theme']);
const toggleTheme = () => {
const newTheme = cookies.theme === 'dark' ? 'light' : 'dark';
setCookie('theme', newTheme, { path: '/' });
};
return (
<div>
<p>Current theme: {cookies.theme || 'light'}</p>
<button onClick={toggleTheme}>Toggle Theme</button>
</div>
);
};
In this example, we’re using the useCookies
hook to manage a user’s theme preference. The hook returns an array with three elements: the current cookies, a function to set cookies, and a function to remove cookies. We’re specifying ‘theme’ as a dependency, which means our component will re-render when the ‘theme’ cookie changes.
Advanced Usage
Working with Cookie Options
When setting cookies, you often need more control over their behavior. react-cookie
allows you to specify various options when setting a cookie:
import React from 'react';
import { useCookies } from 'react-cookie';
const SessionManager: React.FC = () => {
const [cookies, setCookie, removeCookie] = useCookies(['session']);
const login = (token: string) => {
setCookie('session', token, {
path: '/',
maxAge: 3600, // Expires after 1hr
secure: true,
sameSite: 'strict'
});
};
const logout = () => {
removeCookie('session', { path: '/' });
};
return (
<div>
{cookies.session ? (
<button onClick={logout}>Logout</button>
) : (
<button onClick={() => login('example-token')}>Login</button>
)}
</div>
);
};
This example demonstrates setting a session cookie with specific security options. The maxAge
option sets the cookie to expire after one hour, secure
ensures it’s only sent over HTTPS, and sameSite: 'strict'
provides additional protection against CSRF attacks.
Server-Side Rendering with Cookies
One of the great features of react-cookie
is its support for server-side rendering. This is particularly useful when you need to access cookies during the initial render on the server.
import React from 'react';
import { CookiesProvider } from 'react-cookie';
import { renderToString } from 'react-dom/server';
const serverRender = (req, res) => {
const cookies = new Cookies(req.headers.cookie);
const html = renderToString(
<CookiesProvider cookies={cookies}>
<App />
</CookiesProvider>
);
res.send(`<!DOCTYPE html>${html}`);
};
In this server-side rendering example, we’re creating a Cookies
instance from the request headers and passing it to the CookiesProvider
. This ensures that the initial render on the server has access to the same cookies that would be available on the client.
Using withCookies HOC
While hooks are the preferred method in modern React development, react-cookie
also provides a Higher-Order Component (HOC) for class components or situations where you might prefer this pattern:
import React from 'react';
import { withCookies, ReactCookieProps } from 'react-cookie';
interface Props extends ReactCookieProps {
name: string;
}
class Greeting extends React.Component<Props> {
render() {
const { cookies, name } = this.props;
const lastVisit = cookies.get('lastVisit') || 'first time';
cookies.set('lastVisit', new Date().toISOString(), { path: '/' });
return (
<div>
Welcome {name}! Your last visit was: {lastVisit}
</div>
);
}
}
export default withCookies(Greeting);
The withCookies
HOC injects the cookies
prop into your component, providing methods to get, set, and remove cookies. This approach can be particularly useful when working with existing class components or when you need to access cookies in lifecycle methods.
Conclusion
react-cookie
brings a delightful simplicity to cookie management in React applications. Its universal nature makes it an excellent choice for both client-side and server-side rendering scenarios, while its intuitive API ensures that working with cookies is as easy as enjoying your favorite sweet treat.
Whether you’re building a complex application with authentication and personalized user experiences, or simply need a way to remember user preferences, react-cookie
provides the tools you need. Its support for TypeScript, comprehensive documentation, and active community make it a robust choice for developers of all skill levels.
So go ahead, take a bite out of react-cookie
, and watch as your React applications become more stateful, personalized, and delicious than ever before!