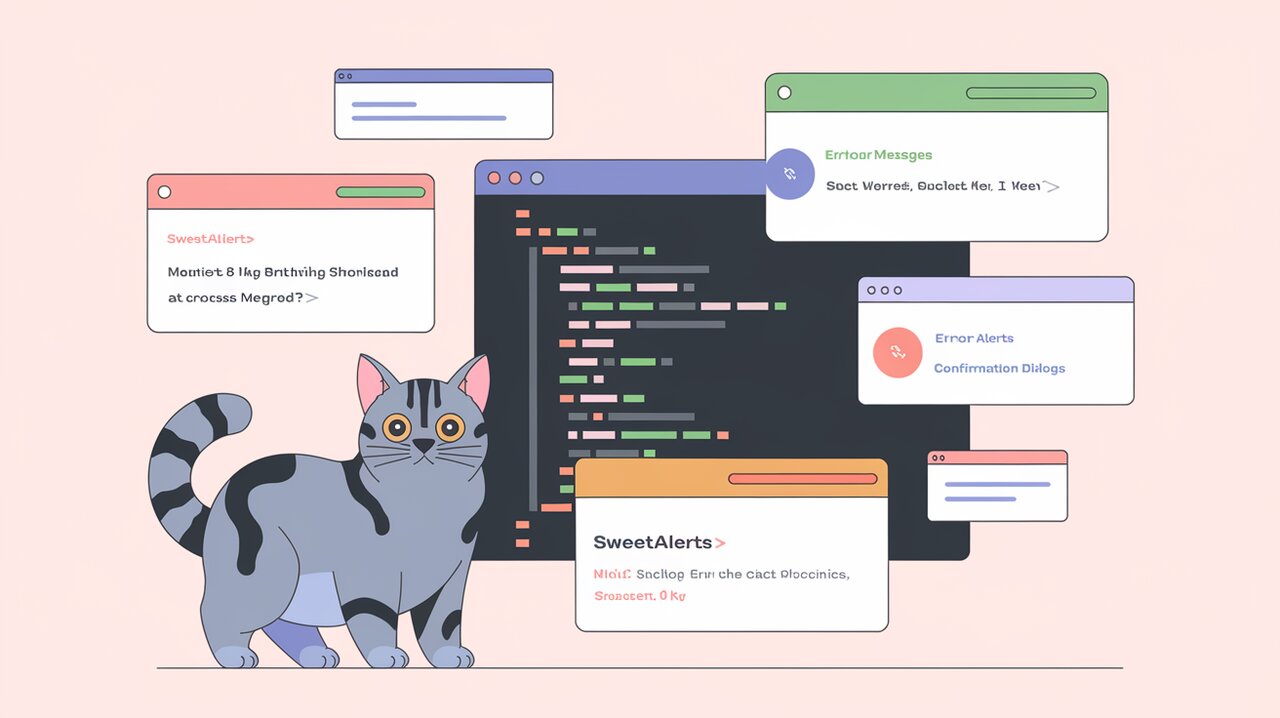
SweetAlert2 is a spellbinding library that transforms the mundane world of JavaScript popups into a realm of beauty and functionality. With zero dependencies and a focus on accessibility, SweetAlert2 offers React developers a powerful tool to create stunning, responsive, and customizable alerts that will enchant users and elevate the overall experience of your applications.
Summoning SweetAlert2 into Your React Project
Before we dive into the magical world of SweetAlert2, let’s first summon it into our React project. Open your terminal and cast the following incantation:
npm install sweetalert2
For those who prefer the mystical arts of Yarn, you can use this alternative spell:
yarn add sweetalert2
Casting Your First Alert
Now that SweetAlert2 is at your fingertips, let’s conjure a basic alert to see its power in action. Import the library into your React component and prepare to be amazed:
import Swal from 'sweetalert2'
const MyComponent = () => {
const showAlert = () => {
Swal.fire('Hello, SweetAlert2!')
}
return (
<button onClick={showAlert}>Click me!</button>
)
}
With this simple incantation, you’ve created a button that, when clicked, will summon a beautiful alert with the message “Hello, SweetAlert2!“. The Swal.fire()
method is the core spell of SweetAlert2, allowing you to create alerts with ease.
Crafting Complex Alerts
SweetAlert2 truly shines when you start to explore its more advanced features. Let’s create a more intricate alert that showcases some of its capabilities:
import Swal from 'sweetalert2'
const showComplexAlert = () => {
Swal.fire({
title: 'Are you sure?',
text: "You won't be able to revert this!",
icon: 'warning',
showCancelButton: true,
confirmButtonColor: '#3085d6',
cancelButtonColor: '#d33',
confirmButtonText: 'Yes, delete it!'
}).then((result) => {
if (result.isConfirmed) {
Swal.fire(
'Deleted!',
'Your file has been deleted.',
'success'
)
}
})
}
This spell creates a confirmation dialog with custom buttons, colors, and icons. It also demonstrates how to handle the user’s response, allowing you to perform actions based on their choice.
Customizing the Look and Feel
SweetAlert2 offers a vast array of customization options to ensure your alerts match the aesthetic of your React application. Let’s explore some of these magical properties:
Swal.fire({
title: 'Custom styled alert',
html: '<i>You can use <b>HTML</b> in your alerts</i>',
icon: 'info',
customClass: {
container: 'my-swal-container',
title: 'my-swal-title',
content: 'my-swal-content',
confirmButton: 'my-swal-confirm-button',
cancelButton: 'my-swal-cancel-button'
},
buttonsStyling: false,
showCloseButton: true,
showCancelButton: true,
focusConfirm: false,
confirmButtonText: 'Great!',
cancelButtonText: 'Not so great...'
})
This incantation demonstrates how to use custom CSS classes, HTML content, and button styling to create a truly unique alert experience.
Advanced Techniques: Input Validation and Asynchronous Operations
SweetAlert2 isn’t just about displaying information; it can also gather input from users and handle asynchronous operations with grace. Behold this advanced spell:
const performAsyncOperation = () => {
Swal.fire({
title: 'Enter your email',
input: 'email',
inputAttributes: {
autocapitalize: 'off'
},
showCancelButton: true,
confirmButtonText: 'Subscribe',
showLoaderOnConfirm: true,
preConfirm: (email) => {
return fetch(`//api.example.com/subscribe?email=${email}`)
.then(response => {
if (!response.ok) {
throw new Error(response.statusText)
}
return response.json()
})
.catch(error => {
Swal.showValidationMessage(
`Request failed: ${error}`
)
})
},
allowOutsideClick: () => !Swal.isLoading()
}).then((result) => {
if (result.isConfirmed) {
Swal.fire({
title: `${result.value.email} subscribed successfully!`
})
}
})
}
This powerful incantation creates an input field for email, validates it, performs an asynchronous API call, and handles the response—all within a single, elegant SweetAlert2 dialog.
Accessibility: Ensuring Your Alerts Are Inclusive
One of the most commendable aspects of SweetAlert2 is its commitment to accessibility. The library adheres to WAI-ARIA guidelines, ensuring that your alerts are usable by all, including those relying on assistive technologies. Here’s how you can enhance the accessibility of your alerts:
Swal.fire({
title: 'Accessible Alert',
text: 'This alert is keyboard-navigable and screen-reader friendly.',
icon: 'info',
confirmButtonText: 'Got it!',
footer: '<a href="#">Why do I have this issue?</a>',
focusConfirm: false,
didOpen: (modal) => {
const confirmButton = modal.querySelector('.swal2-confirm')
confirmButton.focus()
}
})
This spell creates an alert that is fully keyboard-navigable and provides appropriate context for screen readers, ensuring that your React application remains inclusive and accessible to all users.
Conclusion: The Magic of SweetAlert2 in React
SweetAlert2 is truly a magical library that can transform the way you handle alerts and dialogs in your React applications. From simple notifications to complex, interactive modals, SweetAlert2 offers a wide range of possibilities to enhance your user interface.
By leveraging its customization options, input handling capabilities, and commitment to accessibility, you can create popups that are not only visually appealing but also functional and inclusive. As you continue to explore the depths of SweetAlert2, you’ll discover even more ways to enchant your users and elevate your React applications to new heights.
Remember, the true power of SweetAlert2 lies in its flexibility and ease of use. Experiment with different combinations of options, styles, and interactions to find the perfect spell for your specific needs. With SweetAlert2 in your arsenal, you’re well-equipped to create React applications that are both beautiful and user-friendly.
For more React UI magic, check out our articles on React Modals and React Notifications. Happy coding, and may your alerts always be sweet!