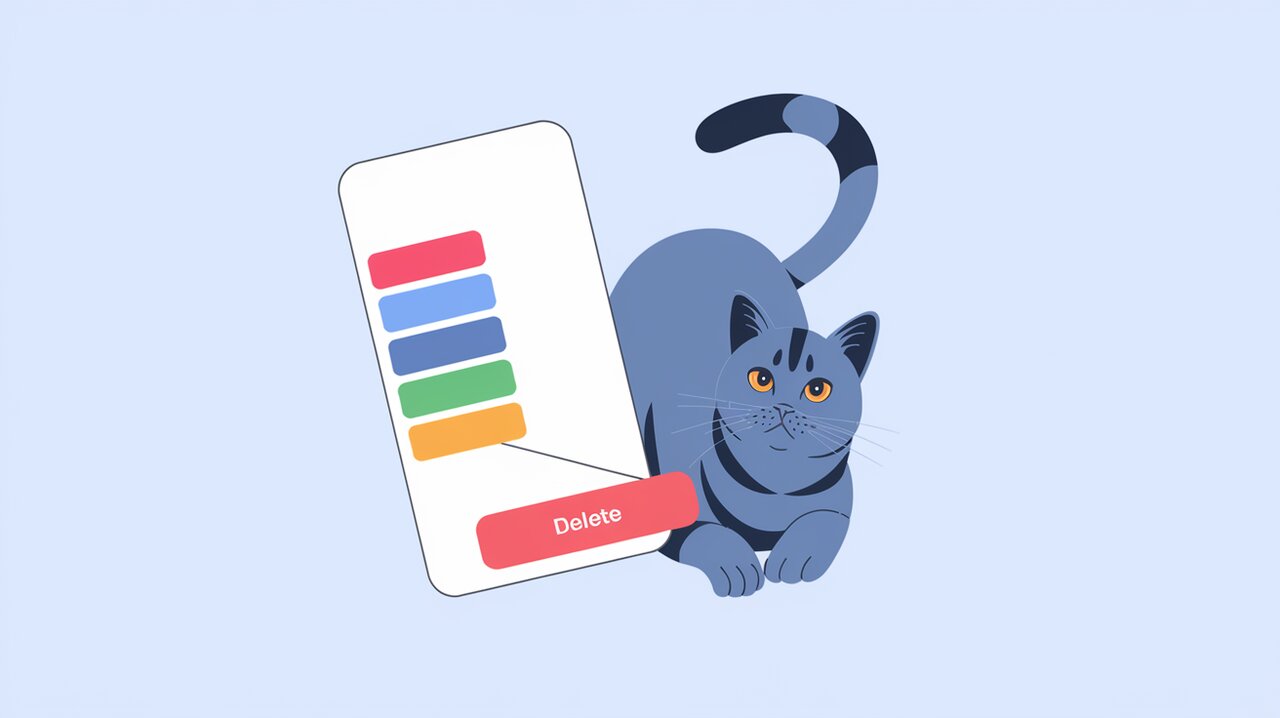
Swipe Away React Elements with iOS Flair: Mastering react-swipe-to-delete-ios
In the world of mobile-first design, intuitive gestures have become a cornerstone of user interaction. One of the most recognizable patterns is the swipe-to-delete functionality popularized by iOS. Now, React developers can easily bring this smooth, satisfying interaction to their web applications with the react-swipe-to-delete-ios
library.
Unveiling the Power of Swipe
react-swipe-to-delete-ios
is a lightweight, zero-dependency React component that mimics the iOS swipe-to-delete behavior. It’s designed to enhance list items in your application, providing users with a familiar and efficient way to remove elements.
Key Features
- iOS-like Behavior: Replicates the smooth, spring-like animation of iOS swipe gestures.
- Customizable: Allows for easy styling and behavior modifications.
- TypeScript Support: Fully typed for enhanced development experience.
- Zero Dependencies: Keeps your project lean without additional bloat.
Getting Started
Let’s dive into how you can integrate this sleek functionality into your React project.
Installation
You can install react-swipe-to-delete-ios
using npm or yarn:
npm install react-swipe-to-delete-ios
Or if you prefer yarn:
yarn add react-swipe-to-delete-ios
Basic Implementation
Once installed, you can start using the component in your React application. Here’s a simple example to get you started:
Creating a Swipeable List Item
import React from 'react';
import SwipeToDelete from 'react-swipe-to-delete-ios';
const ListItem: React.FC = () => {
return (
<SwipeToDelete onDelete={() => console.log('Item deleted')}>
<div>Swipe me to delete!</div>
</SwipeToDelete>
);
};
export default ListItem;
In this basic example, we wrap our list item content with the SwipeToDelete
component. The onDelete
prop is a callback function that will be triggered when the delete action is completed.
Customizing the Delete Button
You can easily customize the appearance of the delete button:
import React from 'react';
import SwipeToDelete from 'react-swipe-to-delete-ios';
const CustomListItem: React.FC = () => {
return (
<SwipeToDelete
onDelete={() => console.log('Item deleted')}
deleteButtonLabel="Remove"
deleteButtonColor="#ff0000"
>
<div>Swipe me to delete!</div>
</SwipeToDelete>
);
};
Here, we’ve changed the label of the delete button to “Remove” and set its color to red. This allows you to match the component’s appearance to your application’s design language.
Advanced Usage
Now that we’ve covered the basics, let’s explore some more advanced features of react-swipe-to-delete-ios
.
Controlling Swipe Behavior
You can fine-tune the swipe behavior by adjusting various props:
import React from 'react';
import SwipeToDelete from 'react-swipe-to-delete-ios';
const AdvancedListItem: React.FC = () => {
return (
<SwipeToDelete
onDelete={() => console.log('Item deleted')}
deleteThreshold={75}
deleteWidth={100}
transitionDuration={250}
>
<div>Advanced swipe settings</div>
</SwipeToDelete>
);
};
In this example:
deleteThreshold
sets the percentage of swipe required to trigger deletion.deleteWidth
determines the width of the delete button area.transitionDuration
controls the speed of the swipe animation.
These settings allow you to create a swipe experience that feels just right for your application.
Implementing Custom Delete Confirmation
For critical actions, you might want to add a confirmation step:
import React, { useState } from 'react';
import SwipeToDelete from 'react-swipe-to-delete-ios';
const ConfirmDeleteItem: React.FC = () => {
const [isDeleting, setIsDeleting] = useState(false);
const handleDelete = () => {
setIsDeleting(true);
// Simulate an API call
setTimeout(() => {
console.log('Item deleted');
setIsDeleting(false);
}, 1000);
};
return (
<SwipeToDelete
onDelete={handleDelete}
deleteButtonLabel={isDeleting ? 'Deleting...' : 'Delete'}
disabled={isDeleting}
>
<div>Swipe to delete with confirmation</div>
</SwipeToDelete>
);
};
This advanced example demonstrates how to handle asynchronous delete operations and provide visual feedback to the user during the process.
Wrapping Up
The react-swipe-to-delete-ios
library offers a seamless way to incorporate iOS-style swipe-to-delete functionality in your React applications. From basic implementation to advanced customization, it provides the flexibility needed to create intuitive and engaging user interfaces.
By leveraging this component, you can enhance the interactivity of your lists, improve user experience, and bring a touch of native mobile feel to your web applications. Whether you’re building a task manager, an email client, or any application with dynamic lists, react-swipe-to-delete-ios
can help you create smooth, satisfying delete interactions that users will love.
Remember to consider the context of your application when implementing swipe-to-delete functionality. While it’s a powerful tool, it’s important to use it judiciously and always provide clear visual cues and feedback to your users.
With react-swipe-to-delete-ios
in your toolkit, you’re well-equipped to create more interactive and user-friendly React applications. Happy coding, and may your lists be ever swipeable!