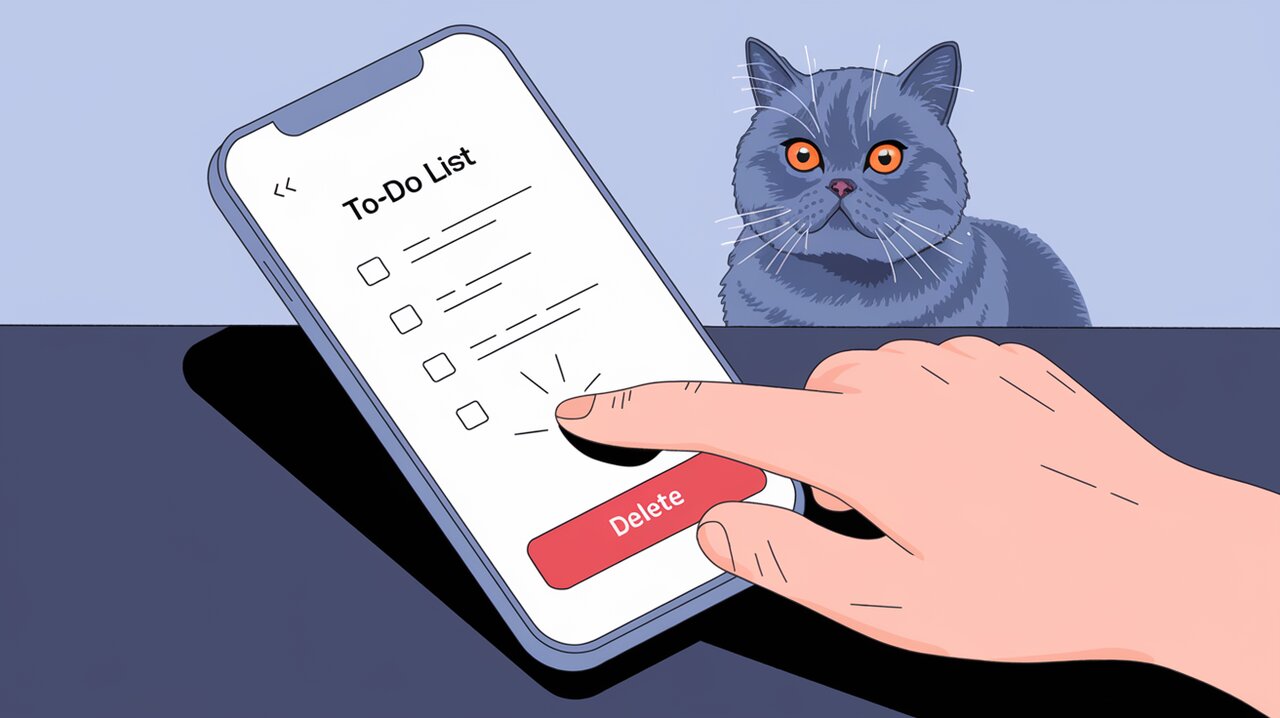
Swipe Away with React: Mastering the Art of Deletable Components
In the world of mobile-first design, intuitive user interactions are key to creating engaging applications. One such interaction that has become ubiquitous is the “swipe to delete” gesture. Today, we’ll explore how to bring this smooth, satisfying functionality to your React applications using the react-swipe-to-delete-component
library.
What is react-swipe-to-delete-component?
The react-swipe-to-delete-component
is a lightweight, easy-to-use React component that implements the “swipe to delete” UI pattern. It allows users to swipe list items horizontally, revealing a delete option underneath. This interaction is not only intuitive but also helps prevent accidental deletions by requiring a deliberate action from the user.
Getting Started
Let’s dive into how you can integrate this sleek functionality into your React project.
Installation
First, you’ll need to install the library. You can do this using npm or yarn:
npm install react-swipe-to-delete-component
or
yarn add react-swipe-to-delete-component
Basic Usage
Once installed, you can start using the component in your React application. Here’s a simple example of how to implement it:
import React from 'react';
import SwipeToDelete from 'react-swipe-to-delete-component';
import 'react-swipe-to-delete-component/dist/swipe-to-delete.css';
const MyList = () => {
const items = [
{ id: 1, text: 'Swipe me to delete!' },
{ id: 2, text: 'Another swipeable item' },
// ... more items
];
return (
<div>
{items.map(item => (
<SwipeToDelete key={item.id}>
<div className="list-item">{item.text}</div>
</SwipeToDelete>
))}
</div>
);
};
export default MyList;
In this example, we’re wrapping each list item with the SwipeToDelete
component. This automatically adds the swipe functionality to each item.
Customization Options
The react-swipe-to-delete-component
library offers several props for customization:
Changing the Root Element
By default, the component uses a div
as its root element. You can change this using the tag
prop:
<SwipeToDelete tag="li">
<div className="list-item">I'm in a list item now!</div>
</SwipeToDelete>
Custom Background
You can customize the background that appears when swiping by using the background
prop:
const customBackground = (
<div style={{ background: 'blue', height: '100%' }}>
Delete
</div>
);
<SwipeToDelete background={customBackground}>
<div className="list-item">Swipe me for a blue surprise!</div>
</SwipeToDelete>
Adjusting Swipe Sensitivity
The deleteSwipe
prop allows you to set how far the user needs to swipe before the delete action is triggered. It’s a number between 0 and 1, representing the percentage of the component’s width:
<SwipeToDelete deleteSwipe={0.7}>
<div className="list-item">You'll need to swipe me further!</div>
</SwipeToDelete>
Handling Delete Actions
To perform actions when an item is deleted, you can use the onDelete
prop:
const handleDelete = (props) => {
console.log('Item deleted:', props);
// Perform delete action here
};
<SwipeToDelete onDelete={handleDelete}>
<div className="list-item">Swipe to delete me</div>
</SwipeToDelete>
Similarly, you can use the onCancel
prop to handle cases where the user starts swiping but doesn’t complete the delete action:
const handleCancel = (props) => {
console.log('Delete cancelled:', props);
};
<SwipeToDelete onCancel={handleCancel}>
<div className="list-item">Try swiping me, but not all the way!</div>
</SwipeToDelete>
Styling
The library comes with default styles, but you can easily override them to match your application’s design. The component uses the following CSS classes:
.js-content
: The content region.js-delete
: The delete region.js-transition-delete-right
and.js-transition-delete-left
: Added when swiping past the delete threshold.js-transition-cancel
: Added when swiping less than the delete threshold
You can target these classes in your CSS to customize the appearance and animations.
Conclusion
The react-swipe-to-delete-component
library offers a simple yet powerful way to implement the popular “swipe to delete” UI pattern in your React applications. By following the examples and customization options outlined in this article, you can create intuitive, interactive list items that enhance your user experience.
Remember, while this interaction is common on mobile devices, it’s also becoming increasingly familiar on desktop applications. Consider using this component to unify your interface across different platforms and provide a consistent, modern feel to your React applications.
For more React UI enhancements, check out our articles on React animations with Framer Motion or creating responsive layouts with React Grid Layout.
Happy swiping!