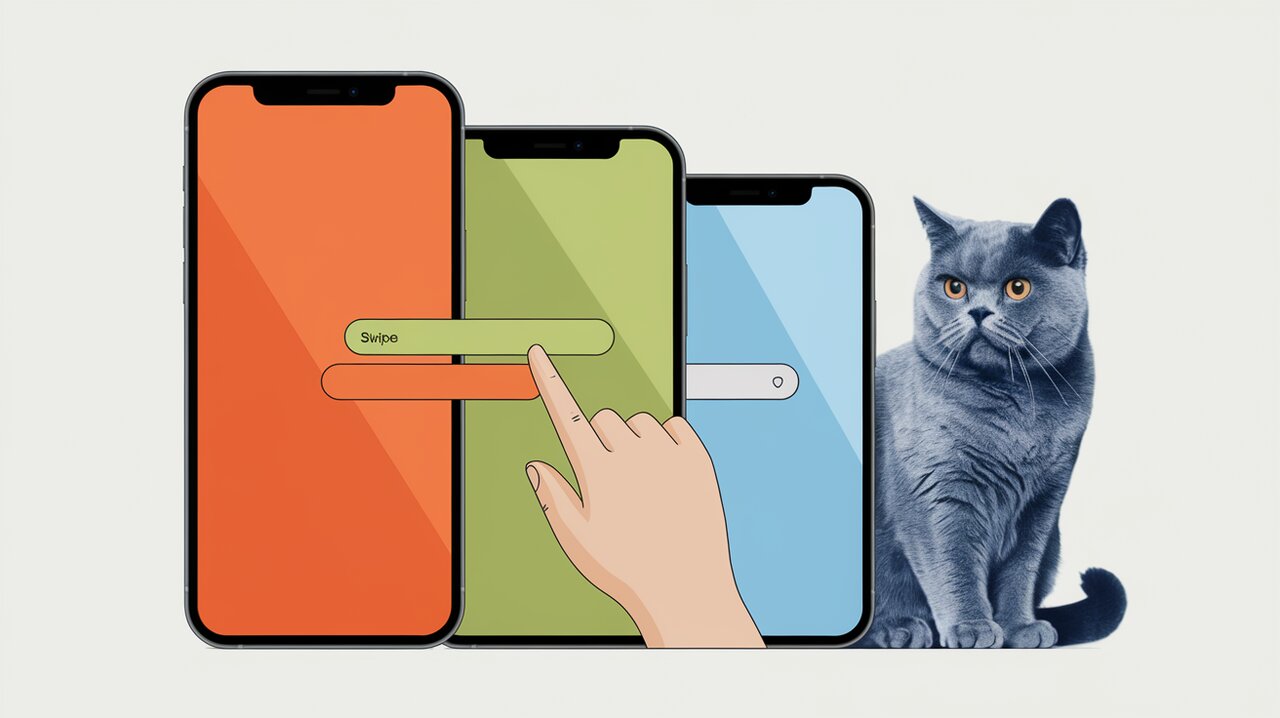
Swipe Your Way to Smooth UX with React Swipeable Views
React Swipeable Views is a powerful React component that enables developers to create smooth, touch-friendly swipeable interfaces. This library is perfect for implementing carousels, slideshows, and other interactive elements that require horizontal swiping functionality. With its small footprint and efficient rendering, react-swipeable-views
is an excellent choice for both web and mobile applications.
Features
- Smooth, hardware-accelerated animations
- Support for both web and React Native (experimental)
- Customizable transitions and animations
- Lazy loading of slides for improved performance
- Responsive design with support for different screen sizes
- TypeScript definitions included
Installation
To get started with react-swipeable-views
, you’ll need to install it in your project. You can use either npm or yarn for installation.
Using npm:
npm install --save react-swipeable-views
Using yarn:
yarn add react-swipeable-views
For React Native projects (experimental support):
npm install --save react-swipeable-views-native
Basic Usage
Let’s dive into the basic usage of react-swipeable-views
with some simple examples.
Creating a Simple Swipeable View
Here’s a basic example of how to create a swipeable view with three slides:
import React from 'react';
import SwipeableViews from 'react-swipeable-views';
const styles = {
slide: {
padding: 15,
minHeight: 100,
color: '#fff',
},
slide1: {
background: '#FEA900',
},
slide2: {
background: '#B3DC4A',
},
slide3: {
background: '#6AC0FF',
},
};
const MyComponent: React.FC = () => (
<SwipeableViews>
<div style={{...styles.slide, ...styles.slide1}}>slide n°1</div>
<div style={{...styles.slide, ...styles.slide2}}>slide n°2</div>
<div style={{...styles.slide, ...styles.slide3}}>slide n°3</div>
</SwipeableViews>
);
export default MyComponent;
In this example, we import the SwipeableViews
component and create three slides with different background colors. The component automatically handles touch and mouse events, allowing users to swipe between slides.
Customizing the Swipeable View
You can customize the behavior of the swipeable view by passing props to the SwipeableViews
component:
import React, { useState } from 'react';
import SwipeableViews from 'react-swipeable-views';
const MyCustomComponent: React.FC = () => {
const [index, setIndex] = useState(0);
const handleChangeIndex = (index: number) => {
setIndex(index);
};
return (
<SwipeableViews
index={index}
onChangeIndex={handleChangeIndex}
enableMouseEvents
resistance
>
<div>Slide 1</div>
<div>Slide 2</div>
<div>Slide 3</div>
</SwipeableViews>
);
};
export default MyCustomComponent;
In this example, we’ve added some custom behavior:
index
andonChangeIndex
are used to control the current slide programmatically.enableMouseEvents
allows desktop users to swipe using their mouse.resistance
adds a resistance effect when trying to swipe past the first or last slide.
Advanced Usage
Now let’s explore some more advanced features of react-swipeable-views
.
Implementing Circular Swiping
To create a circular swipeable view where the last slide loops back to the first, you can use the circular
prop:
import React from 'react';
import SwipeableViews from 'react-swipeable-views';
const CircularSwipeableView: React.FC = () => (
<SwipeableViews circular>
<div>Slide 1</div>
<div>Slide 2</div>
<div>Slide 3</div>
</SwipeableViews>
);
export default CircularSwipeableView;
This creates an infinite loop of slides, allowing users to continuously swipe in either direction.
Custom Animation
You can customize the animation of the swipeable view using the springConfig
prop:
import React from 'react';
import SwipeableViews from 'react-swipeable-views';
const CustomAnimationSwipeableView: React.FC = () => (
<SwipeableViews
springConfig={{
duration: '0.5s',
easeFunction: 'cubic-bezier(0.15, 0.3, 0.25, 1)',
delay: '0s',
}}
>
<div>Slide 1</div>
<div>Slide 2</div>
<div>Slide 3</div>
</SwipeableViews>
);
export default CustomAnimationSwipeableView;
This example uses a custom spring configuration to adjust the animation duration and easing function.
Lazy Loading Slides
For performance optimization, especially with complex slide content, you can implement lazy loading:
import React from 'react';
import SwipeableViews from 'react-swipeable-views';
const LazyLoadingSwipeableView: React.FC = () => (
<SwipeableViews>
<div>Slide 1 (Always rendered)</div>
{({ isActive }) => (
<div style={{ height: '100%' }}>
{isActive ? 'Slide 2 (Rendered when active)' : null}
</div>
)}
{({ isActive }) => (
<div style={{ height: '100%' }}>
{isActive ? 'Slide 3 (Rendered when active)' : null}
</div>
)}
</SwipeableViews>
);
export default LazyLoadingSwipeableView;
In this example, slides 2 and 3 are only rendered when they become active, reducing initial load time and improving performance.
React Native Usage
For React Native projects, you can use the experimental react-swipeable-views-native
package:
import React from 'react';
import { StyleSheet, Text, View } from 'react-native';
import SwipeableViews from 'react-swipeable-views-native';
const styles = StyleSheet.create({
slideContainer: {
height: 100,
},
slide: {
padding: 15,
height: 100,
},
slide1: {
backgroundColor: '#FEA900',
},
slide2: {
backgroundColor: '#B3DC4A',
},
slide3: {
backgroundColor: '#6AC0FF',
},
text: {
color: '#fff',
fontSize: 16,
},
});
const NativeSwipeableView: React.FC = () => (
<SwipeableViews style={styles.slideContainer}>
<View style={[styles.slide, styles.slide1]}>
<Text style={styles.text}>slide n°1</Text>
</View>
<View style={[styles.slide, styles.slide2]}>
<Text style={styles.text}>slide n°2</Text>
</View>
<View style={[styles.slide, styles.slide3]}>
<Text style={styles.text}>slide n°3</Text>
</View>
</SwipeableViews>
);
export default NativeSwipeableView;
This example demonstrates how to use react-swipeable-views
in a React Native environment, providing a native feel to your mobile application.
Conclusion
React Swipeable Views is a versatile and powerful library that enables developers to create engaging, touch-friendly interfaces with ease. Whether you’re building a web application or a mobile app, this library provides the tools you need to implement smooth, responsive swipeable components.
By leveraging features like circular swiping, custom animations, and lazy loading, you can create highly optimized and user-friendly interfaces. The library’s support for both web and React Native platforms makes it a valuable asset for cross-platform development.
As you continue to explore react-swipeable-views
, you’ll discover even more ways to enhance your React applications with intuitive, swipeable interfaces that users will love.