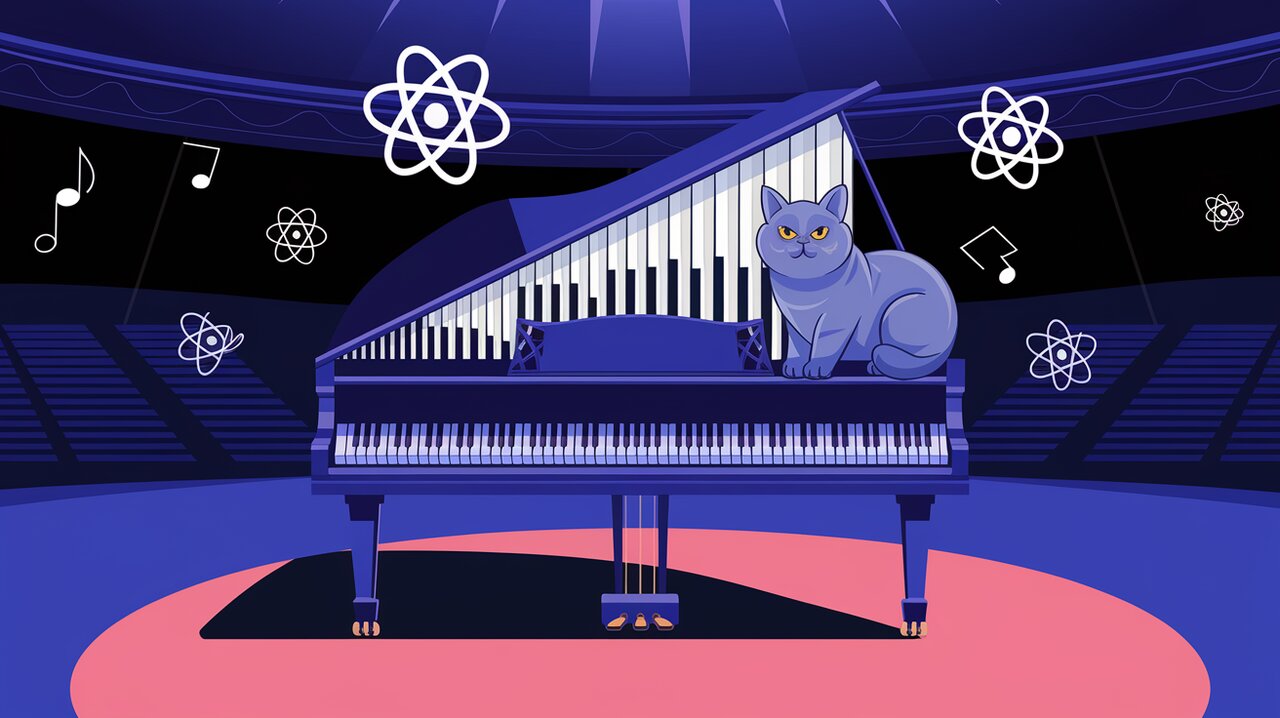
Tabbordion: Orchestrating React's Accordion Symphony
React developers often find themselves in need of versatile components that can handle various UI patterns with ease. Enter react-tabbordion, a powerful library that orchestrates the creation of accordions, tabs, and other interactive elements with the finesse of a symphony conductor. This component not only manages state but also provides tools for complete CSS-based styling and adheres to WAI-ARIA standards, ensuring both flexibility and accessibility.
Composing Your UI Symphony
react-tabbordion is designed to be the maestro of your user interface, allowing you to compose intricate UI patterns with minimal effort. Whether you’re looking to create a simple accordion, a tab component, or even a multi-select list, this library has you covered.
Key Features
- Flexible state management
- Complete CSS-based styling control
- WAI-ARIA compliance for accessibility
- Server-side rendering support
- Keyboard navigation similar to native radio buttons
Setting Up Your Tabbordion Ensemble
To begin using react-tabbordion in your project, you’ll need to install it via npm:
npm install react-tabbordion
Once installed, you can import the necessary components:
import { Tabbordion, TabPanel, TabLabel, TabContent } from 'react-tabbordion'
Basic Usage: Your First Tabbordion Performance
Let’s start with a simple example to showcase how easy it is to create a basic accordion:
import React from 'react'
import { Tabbordion, TabPanel, TabLabel, TabContent } from 'react-tabbordion'
const MyAccordion = () => {
return (
<Tabbordion className="my-accordion" name="example-accordion">
<TabPanel>
<TabLabel>First Movement</TabLabel>
<TabContent>
<h2>Allegro</h2>
<p>The opening movement, fast and lively.</p>
</TabContent>
</TabPanel>
<TabPanel>
<TabLabel>Second Movement</TabLabel>
<TabContent>
<h2>Adagio</h2>
<p>A slower, more expressive movement.</p>
</TabContent>
</TabPanel>
</Tabbordion>
)
}
This code creates a simple accordion with two panels. The Tabbordion
component acts as the container, while TabPanel
, TabLabel
, and TabContent
components structure each accordion item.
Advanced Techniques: Customizing Your Tabbordion
Styling Your Tabbordion
react-tabbordion gives you complete control over styling. You can pass custom class names to each component:
const blockElements = {
content: 'accordion-content',
panel: 'accordion-panel',
label: 'accordion-label'
}
<Tabbordion blockElements={blockElements} className="custom-accordion" name="styled-accordion">
{/* TabPanel components */}
}
</Tabbordion>
State Management: Conducting Your Tabbordion
For more complex scenarios, you might want to control the state of your Tabbordion externally:
import React, { useState } from 'react'
import { Tabbordion, TabPanel, TabLabel, TabContent } from 'react-tabbordion'
const ControlledTabbordion = () => {
const [activePanel, setActivePanel] = useState(0)
const handlePanelChange = (index: number) => {
setActivePanel(index)
}
return (
<Tabbordion
className="controlled-tabbordion"
name="controlled-example"
panels={[activePanel]}
onPanelChange={handlePanelChange}
>
{/* TabPanel components */}
</Tabbordion>
)
}
This example demonstrates how to create a controlled Tabbordion where the active panel is managed by the parent component’s state.
Multiple Selection: Orchestrating Complex Interactions
react-tabbordion also supports multiple selections, perfect for creating multi-select lists or option components:
<Tabbordion
className="multi-select"
name="multi-select-example"
mode="multiple"
initialPanels={[0, 2]}
>
<TabPanel>
<TabLabel>Option 1</TabLabel>
<TabContent>Details for Option 1</TabContent>
</TabPanel>
<TabPanel>
<TabLabel>Option 2</TabLabel>
<TabContent>Details for Option 2</TabContent>
</TabPanel>
<TabPanel>
<TabLabel>Option 3</TabLabel>
<TabContent>Details for Option 3</TabContent>
</TabPanel>
</Tabbordion>
This configuration allows multiple panels to be open simultaneously, with the first and third panels initially selected.
Accessibility: Ensuring Your Tabbordion Resonates with All Users
One of the standout features of react-tabbordion is its built-in accessibility support. The component automatically manages WAI-ARIA attributes, ensuring that your accordion or tabs are navigable and understandable by assistive technologies.
The keyboard navigation works similarly to native radio buttons or checkboxes, depending on the mode you’re using. This means users can navigate through your Tabbordion using familiar keyboard interactions, enhancing the overall user experience.
Performance: A Well-Tuned Instrument
react-tabbordion is designed with performance in mind. All Tabbordion components extend PureComponent, which helps minimize unnecessary renders. Additionally, when using the animateContent
prop, elements are updated when the window is resized, ensuring smooth animations across different viewport sizes.
Conclusion: Your Tabbordion Masterpiece
react-tabbordion provides a powerful and flexible solution for creating accordions, tabs, and other interactive UI elements in React applications. Its focus on customization, accessibility, and performance makes it a valuable addition to any developer’s toolkit.
By leveraging the features of react-tabbordion, you can create rich, interactive user interfaces that are both visually appealing and functionally robust. Whether you’re building a simple accordion or a complex multi-select component, react-tabbordion gives you the tools to orchestrate your UI with precision and flair.
As you continue to explore the capabilities of react-tabbordion, you might also be interested in other React UI components. Check out our articles on React Tabs Simplified Guide and Unleashing Accordion Power: React Accessible Accordion for more insights into creating interactive UI elements in React.
With react-tabbordion in your repertoire, you’re well-equipped to compose stunning, accessible, and performant user interfaces that will delight your users and stand the test of time.