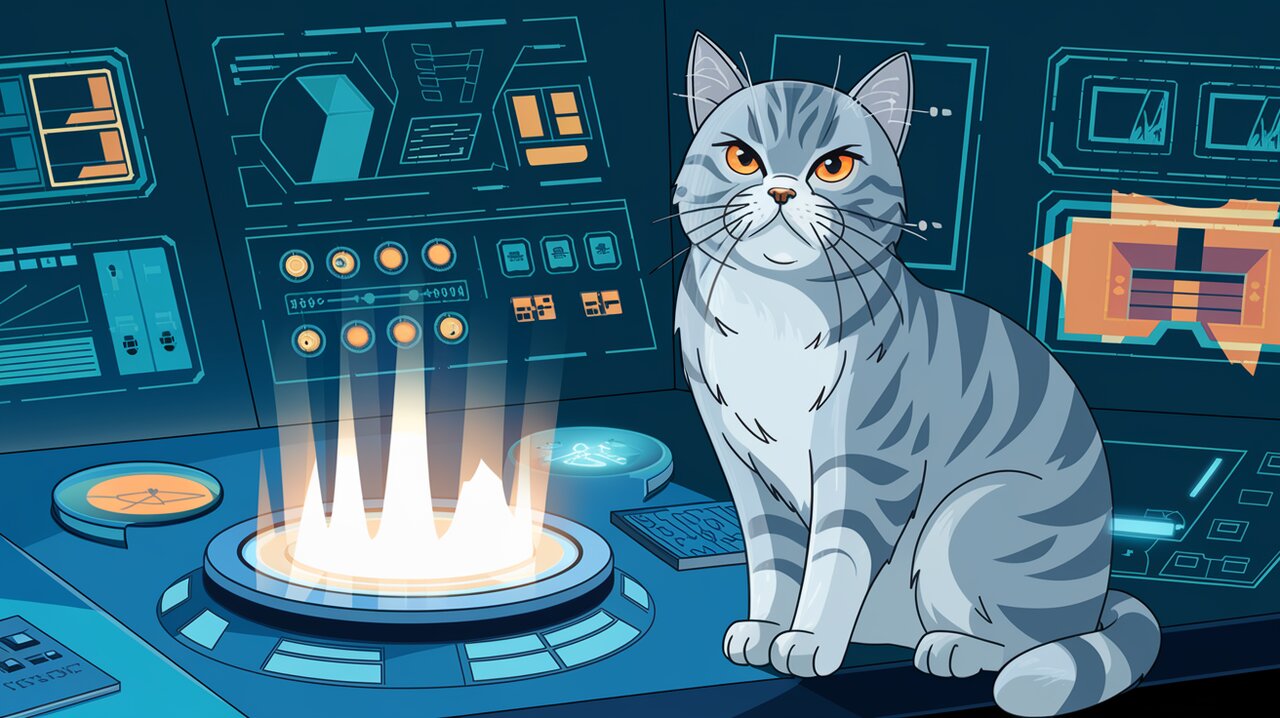
Taming Async Actions with Redux Promise Middleware
In the world of React and Redux applications, handling asynchronous operations can often become a complex and cumbersome task. Enter Redux Promise Middleware, a powerful tool designed to simplify the management of async action creators in Redux. This middleware transforms a single action with an asynchronous payload into separate pending, fulfilled, and rejected actions, providing a clean and intuitive way to handle the different states of asynchronous operations.
Simplifying Async Workflows
Redux Promise Middleware offers several key features that make it an invaluable addition to your Redux toolkit:
- Automatic Action Splitting: The middleware automatically splits a single async action into multiple actions representing different states of the promise.
- Promise Integration: Seamlessly works with JavaScript Promises, allowing you to use async/await syntax in your action creators.
- Thunk Compatibility: Can be combined with Redux Thunk for more complex action chaining scenarios.
- Customizable Action Types: Allows you to customize the suffixes used for pending, fulfilled, and rejected actions.
- Error Handling: Provides built-in error handling for rejected promises.
Getting Started with Redux Promise Middleware
Before we dive into the usage, let’s set up Redux Promise Middleware in your project. You can install it using npm or yarn:
npm install redux-promise-middleware
or
yarn add redux-promise-middleware
Once installed, you need to apply the middleware to your Redux store:
import { createStore, applyMiddleware } from 'redux';
import promiseMiddleware from 'redux-promise-middleware';
import rootReducer from './reducers';
const store = createStore(
rootReducer,
applyMiddleware(promiseMiddleware)
);
Basic Usage Patterns
Creating Async Actions
With Redux Promise Middleware, you can create async actions by returning an object with a payload
property that contains a promise:
const fetchUser = (userId: string) => ({
type: 'FETCH_USER',
payload: api.fetchUser(userId)
});
The middleware will automatically dispatch three actions:
FETCH_USER_PENDING
when the promise startsFETCH_USER_FULFILLED
when the promise resolves successfullyFETCH_USER_REJECTED
if the promise is rejected
Handling Actions in Reducers
Your reducers can now handle these different action types to update the state accordingly:
const initialState = {
user: null,
loading: false,
error: null
};
const userReducer = (state = initialState, action) => {
switch (action.type) {
case 'FETCH_USER_PENDING':
return { ...state, loading: true };
case 'FETCH_USER_FULFILLED':
return { ...state, loading: false, user: action.payload };
case 'FETCH_USER_REJECTED':
return { ...state, loading: false, error: action.payload };
default:
return state;
}
};
This pattern allows for a clean separation of concerns and makes it easy to manage loading states and error handling.
Advanced Techniques
Customizing Action Types
If you prefer different suffixes for your action types, you can customize them when applying the middleware:
import promiseMiddleware from 'redux-promise-middleware';
const customizedPromiseMiddleware = promiseMiddleware({
promiseTypeSuffixes: ['LOADING', 'SUCCESS', 'ERROR']
});
const store = createStore(
rootReducer,
applyMiddleware(customizedPromiseMiddleware)
);
Now your actions will be suffixed with _LOADING
, _SUCCESS
, and _ERROR
instead of the default suffixes.
Combining with Redux Thunk
Redux Promise Middleware plays well with Redux Thunk, allowing for more complex action creators:
import { Dispatch } from 'redux';
const complexAction = (userId: string) => {
return async (dispatch: Dispatch) => {
const userResponse = await dispatch(fetchUser(userId));
if (userResponse.value.role === 'admin') {
dispatch(fetchAdminDashboard());
}
};
};
This example demonstrates how you can chain actions based on the result of a previous async action.
Optimistic Updates
You can implement optimistic updates by dispatching an action before the async operation and then updating it based on the result:
const optimisticUpdate = (data: any) => ({
type: 'OPTIMISTIC_UPDATE',
payload: {
data,
promise: api.updateData(data)
}
});
// In your reducer
case 'OPTIMISTIC_UPDATE':
return { ...state, data: action.payload.data };
case 'OPTIMISTIC_UPDATE_FULFILLED':
return { ...state, data: action.payload };
case 'OPTIMISTIC_UPDATE_REJECTED':
return { ...state, data: action.payload.data }; // Revert to original data
This pattern allows for a more responsive user interface by immediately updating the UI and then confirming or reverting based on the server response.
Wrapping Up
Redux Promise Middleware offers a powerful and flexible way to handle asynchronous operations in your Redux applications. By automatically managing the lifecycle of promise-based actions, it significantly reduces boilerplate code and makes your async logic more readable and maintainable.
Whether you’re building a small React application or a large-scale enterprise system, integrating Redux Promise Middleware into your workflow can streamline your state management and help you focus on building great features rather than wrestling with async code.
As you continue to explore the capabilities of this middleware, you’ll discover even more ways to optimize your Redux code and create more robust, responsive React applications. Happy coding!