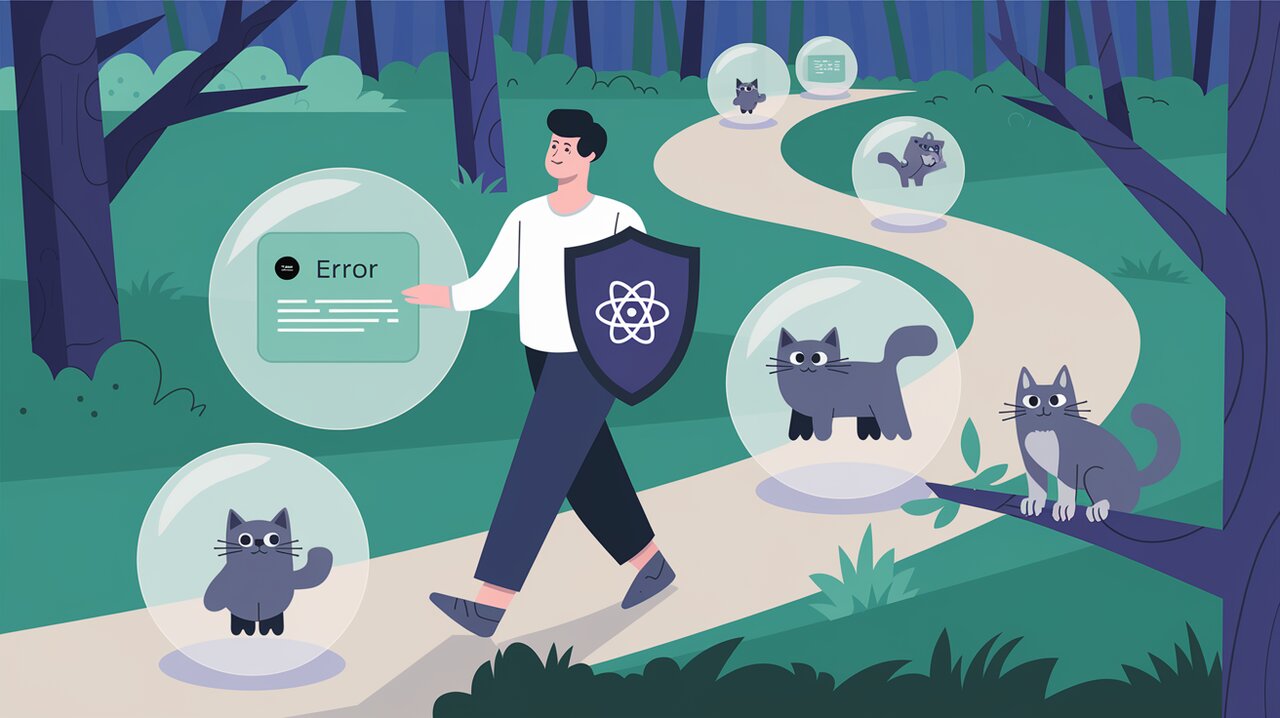
Taming the Wild Errors: A Journey with react-error-boundary
In the ever-evolving landscape of web development, handling errors gracefully is crucial for maintaining a smooth user experience. React applications, with their component-based architecture, present unique challenges when it comes to error management. Enter react-error-boundary, a powerful library designed to catch and handle errors in React applications with finesse.
Features
react-error-boundary
offers a suite of tools to enhance your error handling capabilities:
- Flexible Error Catching: Capture errors anywhere in your component tree.
- Customizable Fallback UI: Display user-friendly error messages or alternative content.
- Error Recovery: Implement retry mechanisms to recover from errors gracefully.
- Error Logging: Easily integrate with error reporting services for better debugging.
- TypeScript Support: Enjoy full type safety with built-in TypeScript definitions.
Installation
Getting started with react-error-boundary
is a breeze. Choose your preferred package manager and run one of the following commands:
# npm
npm install react-error-boundary
# pnpm
pnpm add react-error-boundary
# yarn
yarn add react-error-boundary
Basic Usage
ErrorBoundary Component
The core of react-error-boundary
is the ErrorBoundary
component. It acts as a safety net, catching errors in its child components and rendering a fallback UI when necessary.
import React from 'react';
import { ErrorBoundary } from 'react-error-boundary';
function ErrorFallback({ error }: { error: Error }) {
return (
<div role="alert">
<p>Oops! Something went wrong:</p>
<pre style={{ color: 'red' }}>{error.message}</pre>
</div>
);
}
function App() {
return (
<ErrorBoundary FallbackComponent={ErrorFallback}>
<YourApp />
</ErrorBoundary>
);
}
In this example, we wrap our main application component with ErrorBoundary
. If any error occurs within YourApp
or its children, the ErrorFallback
component will be rendered instead, displaying the error message.
Fallback with Reset Functionality
Sometimes, you might want to give users the option to retry after an error occurs. react-error-boundary
makes this simple:
function ErrorFallback({ error, resetErrorBoundary }: ErrorFallbackProps) {
return (
<div role="alert">
<p>Something went wrong:</p>
<pre style={{ color: 'red' }}>{error.message}</pre>
<button onClick={resetErrorBoundary}>Try again</button>
</div>
);
}
function App() {
return (
<ErrorBoundary
FallbackComponent={ErrorFallback}
onReset={() => {
// Reset the state of your app so the error doesn't happen again
}}
>
<YourApp />
</ErrorBoundary>
);
}
The resetErrorBoundary
function allows users to attempt recovery from the error, potentially reloading the component or resetting the application state.
Advanced Usage
Error Logging
For production applications, it’s crucial to log errors for later analysis. react-error-boundary
makes this straightforward:
import { ErrorBoundary } from 'react-error-boundary';
function logError(error: Error, info: { componentStack: string }) {
// Send error to your error tracking service
console.error('Caught an error:', error, info);
}
function App() {
return (
<ErrorBoundary FallbackComponent={ErrorFallback} onError={logError}>
<YourApp />
</ErrorBoundary>
);
}
The onError
prop allows you to define a function that will be called whenever an error is caught, perfect for integrating with error tracking services.
useErrorBoundary Hook
For more granular control, react-error-boundary
provides the useErrorBoundary
hook:
import { useErrorBoundary } from 'react-error-boundary';
function ComponentWithApiCall() {
const { showBoundary } = useErrorBoundary();
const fetchData = async () => {
try {
const data = await fetchSomeData();
// Process data
} catch (error) {
showBoundary(error);
}
};
return (
<button onClick={fetchData}>Fetch Data</button>
);
}
This hook is particularly useful for handling asynchronous errors, such as those from API calls, which aren’t automatically caught by error boundaries.
Higher-Order Component
For those who prefer a higher-order component approach, react-error-boundary
offers withErrorBoundary
:
import { withErrorBoundary } from 'react-error-boundary';
const ComponentWithErrorBoundary = withErrorBoundary(YourComponent, {
FallbackComponent: ErrorFallback,
onError: (error, info) => {
// Log the error
console.error('Error:', error, info);
},
});
This approach is excellent for adding error boundaries to specific components without modifying their internal structure.
Conclusion
react-error-boundary is a powerful tool in the React developer’s arsenal. By providing a simple yet flexible API for error handling, it allows you to create more robust and user-friendly applications. Whether you’re building a small project or a large-scale application, integrating react-error-boundary
can significantly improve your error management strategy.
Remember, effective error handling is not just about preventing crashes; it’s about creating a seamless experience for your users, even when things go wrong. With react-error-boundary, you’re well-equipped to tackle the wild errors of the web development jungle, ensuring your React applications remain stable, informative, and user-friendly.
So go forth, brave developer, and tame those errors with confidence!