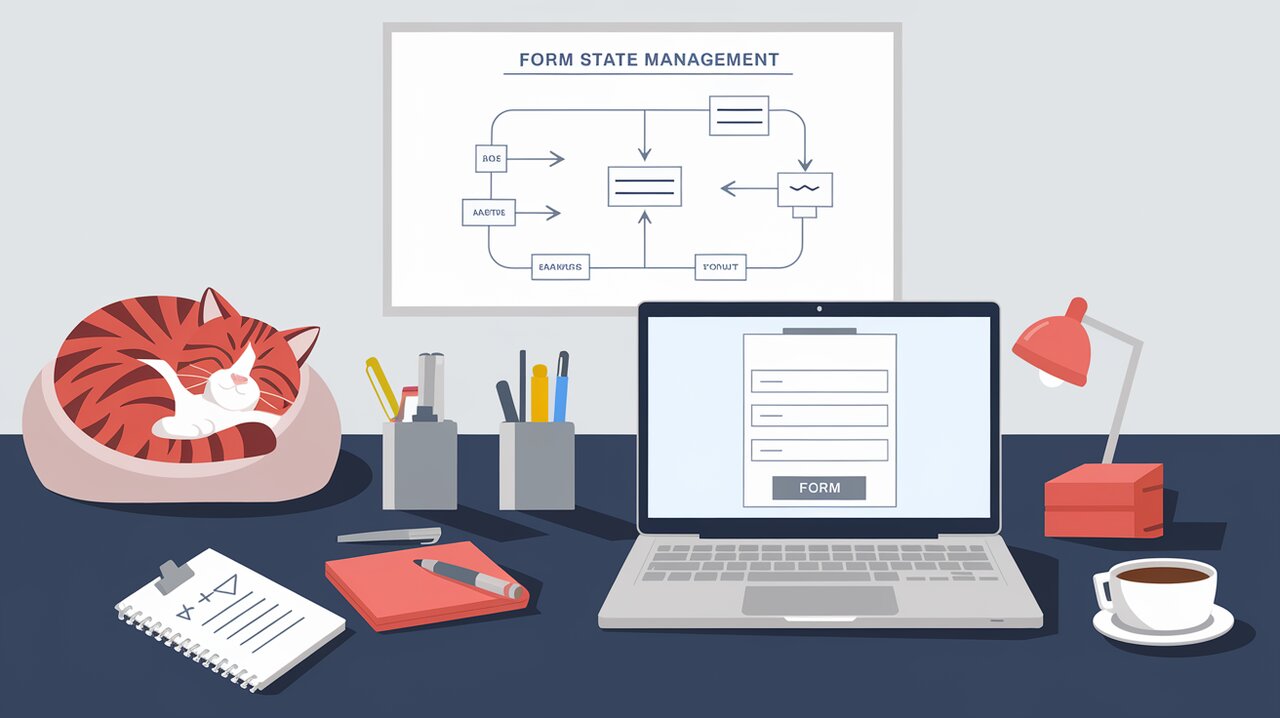
Taming React Forms with Simple Redux Form: A Lightweight Alternative
In the world of React development, managing form state can often become a complex task, especially when integrating with Redux for state management. While robust solutions like Redux Form exist, they can be overkill for smaller projects or simpler form requirements. This is where Simple Redux Form comes to the rescue, offering a lightweight alternative that simplifies form handling in React applications with Redux integration.
What’s in the Toolbox?
Simple Redux Form brings several key features to the table:
- Lightweight Design: Focused on simplicity, it’s perfect for small to medium-sized forms without the overhead of larger libraries.
- Redux Integration: Seamlessly works with your existing Redux setup.
- Field Management: Easily handle form fields with built-in props for value, onChange, error, and touched states.
- Validation Support: Implement custom validation logic with ease.
- Higher-Order Component: Wrap your form components to enhance them with form management capabilities.
Getting Started
To begin using Simple Redux Form in your project, you’ll need to install it first. You can do this using either npm or yarn:
# Using npm
npm install --save simple-redux-form
# Using yarn
yarn add simple-redux-form
Setting Up the Foundation
Integrating the Reducer
The first step in using Simple Redux Form is to integrate its reducer into your Redux store. This allows the library to manage form state within your Redux setup.
import { combineReducers } from 'redux';
import { reducer as simpleFormReducer } from 'simple-redux-form';
const rootReducer = combineReducers({
// Your other reducers
form: simpleFormReducer
});
export default rootReducer;
By adding the simpleFormReducer
to your root reducer, you’re giving Simple Redux Form the ability to handle form-related actions and state updates.
Creating Your First Form
Now that we have the reducer set up, let’s create a basic form using Simple Redux Form. We’ll start with a simple example of a user registration form:
import React from 'react';
import simpleReduxForm from 'simple-redux-form';
interface UserFormProps {
fields: {
username: any;
email: any;
};
}
const UserForm: React.FC<UserFormProps> = ({ fields: { username, email } }) => (
<form>
<div>
<label htmlFor="username">Username:</label>
<input id="username" type="text" {...username} />
</div>
<div>
<label htmlFor="email">Email:</label>
<input id="email" type="email" {...email} />
</div>
<button type="submit">Register</button>
</form>
);
const fields = ['username', 'email'];
export default simpleReduxForm(UserForm, {
form: 'userRegistration',
fields
});
In this example, we’ve created a simple form with username and email fields. The simpleReduxForm
higher-order component wraps our UserForm
, providing it with the necessary props to manage form state.
Diving Deeper: Advanced Usage
Adding Validation
One of the powerful features of Simple Redux Form is its support for custom validation. Let’s enhance our user registration form with some basic validation:
import React from 'react';
import simpleReduxForm from 'simple-redux-form';
interface UserFormProps {
fields: {
username: any;
email: any;
};
}
const UserForm: React.FC<UserFormProps> = ({ fields: { username, email } }) => (
<form>
<div>
<label htmlFor="username">Username:</label>
<input id="username" type="text" {...username} />
{username.touched && username.error && <span>{username.error}</span>}
</div>
<div>
<label htmlFor="email">Email:</label>
<input id="email" type="email" {...email} />
{email.touched && email.error && <span>{email.error}</span>}
</div>
<button type="submit">Register</button>
</form>
);
const fields = ['username', 'email'];
const validate = (values: any) => {
const errors: any = {};
if (!values.username) {
errors.username = 'Username is required';
}
if (!values.email) {
errors.email = 'Email is required';
} else if (!/^[A-Z0-9._%+-]+@[A-Z0-9.-]+\.[A-Z]{2,4}$/i.test(values.email)) {
errors.email = 'Invalid email address';
}
return errors;
};
export default simpleReduxForm(UserForm, {
form: 'userRegistration',
fields,
validate
});
In this enhanced version, we’ve added a validate
function that checks for required fields and a valid email format. The form now displays error messages when validation fails.
Custom Input Components
Simple Redux Form allows you to use custom input components easily. Let’s create a reusable input component:
import React from 'react';
interface CustomInputProps {
label: string;
type: string;
value: string;
onChange: (event: React.ChangeEvent<HTMLInputElement>) => void;
error?: string;
touched?: boolean;
}
const CustomInput: React.FC<CustomInputProps> = ({ label, type, value, onChange, error, touched }) => (
<div>
<label>{label}</label>
<input type={type} value={value} onChange={onChange} />
{touched && error && <span className="error">{error}</span>}
</div>
);
export default CustomInput;
Now, let’s use this custom input in our form:
import React from 'react';
import simpleReduxForm from 'simple-redux-form';
import CustomInput from './CustomInput';
interface UserFormProps {
fields: {
username: any;
email: any;
};
}
const UserForm: React.FC<UserFormProps> = ({ fields: { username, email } }) => (
<form>
<CustomInput label="Username" type="text" {...username} />
<CustomInput label="Email" type="email" {...email} />
<button type="submit">Register</button>
</form>
);
const fields = ['username', 'email'];
const validate = (values: any) => {
// Validation logic (same as before)
};
export default simpleReduxForm(UserForm, {
form: 'userRegistration',
fields,
validate
});
By using custom input components, you can create more reusable and maintainable form structures.
Wrapping Up
Simple Redux Form offers a streamlined approach to handling forms in React applications with Redux. Its lightweight nature makes it an excellent choice for projects that don’t require the full feature set of larger form libraries. By providing easy integration with Redux, field management, and validation support, it simplifies the often complex task of form state management.
Whether you’re building a simple contact form or a more complex user registration system, Simple Redux Form provides the tools you need without unnecessary overhead. Its focus on simplicity and efficiency makes it a valuable addition to any React developer’s toolkit, especially when working on smaller to medium-sized projects.
As you continue to explore Simple Redux Form, you’ll find that it strikes a perfect balance between functionality and simplicity, allowing you to create robust forms without the complexity often associated with form management in React and Redux applications.