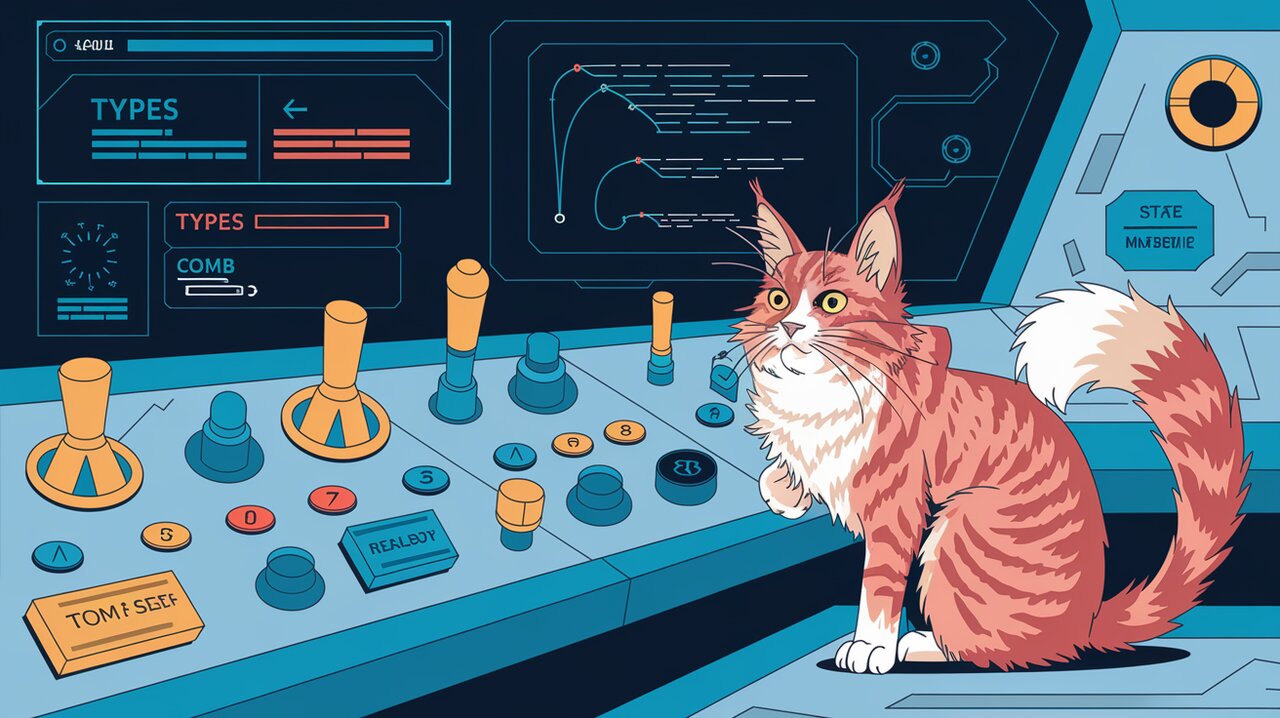
Taming Redux: Unleash the Power of Type-Checking with redux-tcomb
In the ever-evolving landscape of JavaScript development, managing state in complex applications can be a daunting task. Redux has long been a popular choice for state management, but it doesn’t inherently provide type safety or immutability guarantees. This is where redux-tcomb steps in, offering a powerful solution to enhance your Redux workflow with robust type-checking and immutability.
Unveiling the Magic of redux-tcomb
redux-tcomb is a library that seamlessly integrates with Redux to provide immutable and type-checked state and actions. Built on top of the tcomb library, it brings static type checking to your Redux applications, helping catch errors early and improve overall code quality.
Key Features
- Type-checked State: Ensure your application state always conforms to the defined structure.
- Immutable Actions: Prevent unintended modifications to action objects.
- Runtime Type Checking: Catch type errors during development and testing phases.
- Custom Action Creators: Generate type-safe action creators automatically.
- Middleware Integration: Easily add type checking to your Redux middleware stack.
Getting Started with redux-tcomb
Installation
To start using redux-tcomb in your project, you can install it via npm or yarn:
npm install redux-tcomb tcomb
Or if you prefer yarn:
yarn add redux-tcomb tcomb
Basic Usage: Defining Types and Actions
Let’s dive into a simple example to demonstrate how redux-tcomb can be used to create type-safe Redux applications.
Setting Up Types
First, we’ll define our state and action types:
import t from 'tcomb';
import { createActionType } from 'redux-tcomb';
// Define the state type
const State = t.Integer;
// Define a custom type for positive integers
const PositiveInteger = t.refinement(t.Integer, n => n >= 0, 'PositiveInteger');
// Define action types
const Action = createActionType({
INCREMENT: t.interface({ delta: PositiveInteger }),
DECREMENT: t.interface({ delta: PositiveInteger })
});
In this example, we’ve defined our state as an integer and created a custom type for positive integers. We’ve also defined two actions, INCREMENT
and DECREMENT
, both of which expect a delta
of type PositiveInteger
.
Creating a Type-Safe Reducer
Now, let’s create a reducer that uses these types:
import { createCheckedReducer } from 'redux-tcomb';
function counterReducer(state = 0, action) {
switch(action.type) {
case 'INCREMENT':
return state + action.delta;
case 'DECREMENT':
return state - action.delta;
default:
return state;
}
}
const checkedReducer = createCheckedReducer(counterReducer, State);
The createCheckedReducer
function wraps our regular reducer with type checking, ensuring that the state always matches our defined State
type.
Advanced Usage: Middleware and Store Configuration
Type-Checked Middleware
redux-tcomb allows you to add type checking to your Redux middleware stack:
import { createStore, applyMiddleware } from 'redux';
import { createCheckedMiddleware } from 'redux-tcomb';
import logger from 'redux-logger';
const store = createStore(
checkedReducer,
applyMiddleware(
createCheckedMiddleware(Action),
logger
)
);
The createCheckedMiddleware
function creates a middleware that checks all dispatched actions against our defined Action
type.
Dispatching Actions
With our store configured, we can now dispatch actions with confidence:
// This will work fine
store.dispatch({ type: 'INCREMENT', delta: 2 });
// This will throw a type error
store.dispatch({ type: 'INCREMENT', delta: -2 });
// This will also throw an error due to the typo in the action type
store.dispatch({ type: 'INCRE', delta: 1 });
Leveraging Custom Action Creators
redux-tcomb provides a way to create type-safe action creators:
const incrementAction = Action.INCREMENT({ delta: 5 });
store.dispatch(incrementAction);
This approach not only ensures type safety but also provides a more declarative way of creating actions.
Best Practices and Tips
Refining Types for Better Precision
You can create more specific types to catch potential errors:
const MaxValue = t.refinement(PositiveInteger, n => n <= 100, 'MaxValue');
const RefinedAction = createActionType({
INCREMENT: t.interface({ delta: MaxValue }),
DECREMENT: t.interface({ delta: MaxValue })
});
This ensures that the delta
value is not only positive but also doesn’t exceed 100.
Combining with TypeScript
While redux-tcomb provides runtime type checking, combining it with TypeScript can give you the best of both worlds:
interface CounterState {
value: number;
}
const TypedState = t.interface({
value: t.Number
});
// Use TypeScript interfaces with redux-tcomb
const typedReducer: Reducer<CounterState> = createCheckedReducer(
(state: CounterState, action) => {
// Reducer logic here
},
TypedState as any
);
This approach provides both compile-time and runtime type checking, significantly reducing the chances of type-related bugs.
Conclusion
redux-tcomb brings a new level of type safety and immutability to Redux applications. By leveraging its features, developers can catch errors earlier, write more robust code, and improve the overall reliability of their applications. While it does add an extra layer to your Redux setup, the benefits in terms of code quality and developer experience are substantial.
As you continue to explore redux-tcomb, you’ll discover more ways to leverage its power in your Redux applications. Whether you’re building a small project or a large-scale application, the type safety and immutability guarantees provided by redux-tcomb can be a game-changer in your development process.