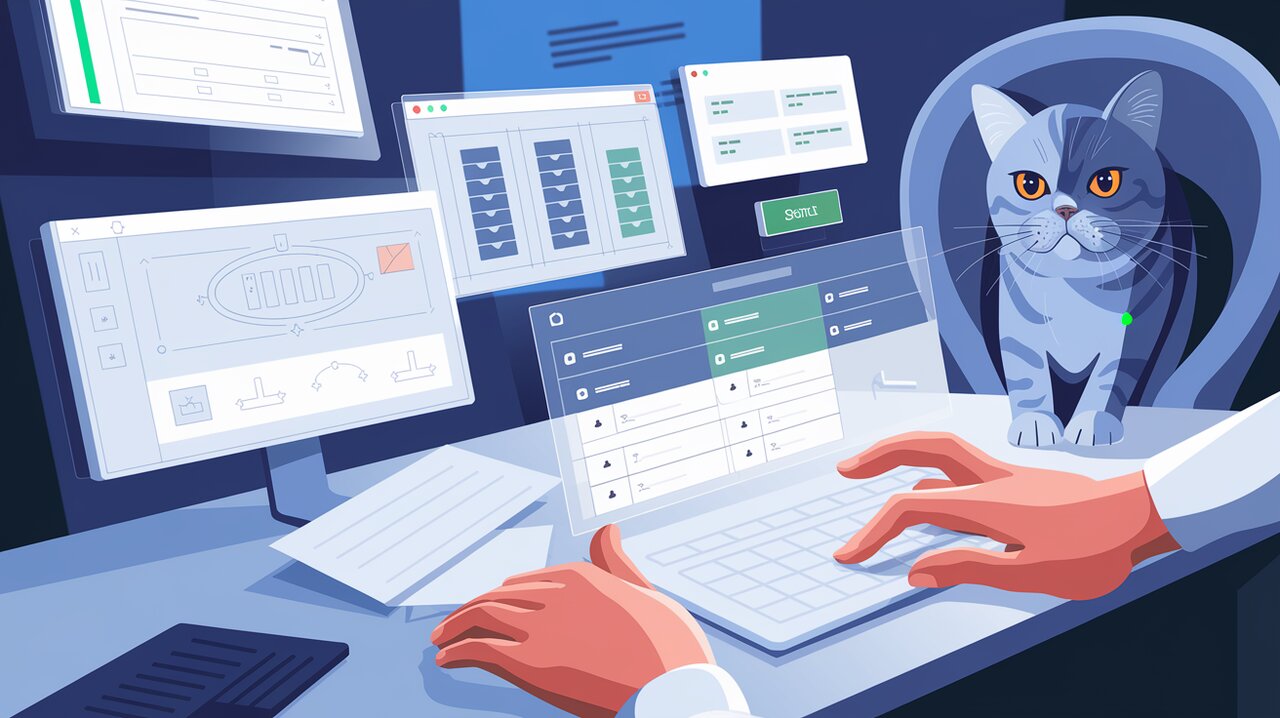
TanStack Table Tango: Mastering Data Grids in React
TanStack Table**, formerly known as React Table, is a powerful and flexible headless UI library for building tables and data grids in React applications. With its lightweight core and extensive feature set, it has become a go-to solution for developers seeking to create highly customizable and performant data displays. In this article, we’ll explore the capabilities of TanStack Table** and learn how to harness its power in your React projects.
Features That Make TanStack Table Shine
TanStack Table** comes packed with an impressive array of features that cater to various data presentation needs:
- Headless UI: The library provides complete control over markup and styles, allowing seamless integration with any UI framework or design system.
- Lightweight: With a small footprint of ~15kb (when tree-shaken), it won’t bloat your application.
- TypeScript Support: Enjoy full TypeScript support for type-safe development.
- Sorting: Implement multi-column, multi-directional sorting with ease.
- Filtering: Apply column-specific and global filters to your data.
- Pagination: Create paginated tables effortlessly.
- Row Selection: Enable users to select single or multiple rows.
- Row Expansion: Allow users to expand rows for additional details.
- Column Management: Handle column visibility, ordering, pinning, and resizing.
- Virtualization: Optimize performance for large datasets with virtualization support.
Getting Started with TanStack Table
Installation
To begin using TanStack Table in your React project, install it via npm or yarn:
npm install @tanstack/react-table
# or
yarn add @tanstack/react-table
Basic Usage
Let’s create a simple table to demonstrate the basic usage of TanStack Table:
import React from 'react'
import { useTable, Column } from '@tanstack/react-table'
interface Person {
firstName: string
lastName: string
age: number
}
const data: Person[] = [
{ firstName: 'John', lastName: 'Doe', age: 30 },
{ firstName: 'Jane', lastName: 'Smith', age: 25 },
{ firstName: 'Bob', lastName: 'Johnson', age: 45 },
]
const columns: Column<Person>[] = [
{
Header: 'First Name',
accessor: 'firstName',
},
{
Header: 'Last Name',
accessor: 'lastName',
},
{
Header: 'Age',
accessor: 'age',
},
]
function Table() {
const {
getTableProps,
getTableBodyProps,
headerGroups,
rows,
prepareRow,
} = useTable({ columns, data })
return (
<table {...getTableProps()}>
<thead>
{headerGroups.map(headerGroup => (
<tr {...headerGroup.getHeaderGroupProps()}>
{headerGroup.headers.map(column => (
<th {...column.getHeaderProps()}>{column.render('Header')}</th>
))}
</tr>
))}
</thead>
<tbody {...getTableBodyProps()}>
{rows.map(row => {
prepareRow(row)
return (
<tr {...row.getRowProps()}>
{row.cells.map(cell => (
<td {...cell.getCellProps()}>{cell.render('Cell')}</td>
))}
</tr>
)
})}
</tbody>
</table>
)
}
This example demonstrates how to create a basic table using TanStack Table. The useTable
hook is the core of the library, providing all the necessary props and functions to render your table.
Advanced Usage
Sorting
To add sorting functionality to your table, use the useSortBy
plugin:
import { useTable, useSortBy } from '@tanstack/react-table'
function Table() {
const {
getTableProps,
getTableBodyProps,
headerGroups,
rows,
prepareRow,
} = useTable(
{
columns,
data,
},
useSortBy
)
return (
<table {...getTableProps()}>
<thead>
{headerGroups.map(headerGroup => (
<tr {...headerGroup.getHeaderGroupProps()}>
{headerGroup.headers.map(column => (
<th {...column.getHeaderProps(column.getSortByToggleProps())}>
{column.render('Header')}
<span>
{column.isSorted
? column.isSortedDesc
? ' 🔽'
: ' 🔼'
: ''}
</span>
</th>
))}
</tr>
))}
</thead>
{/* ... table body ... */}
</table>
)
}
This code adds sorting indicators and functionality to your table headers.
Filtering
Implement filtering with the useFilters
plugin:
import { useTable, useFilters } from '@tanstack/react-table'
function Table() {
const {
getTableProps,
getTableBodyProps,
headerGroups,
rows,
prepareRow,
setFilter,
} = useTable(
{
columns,
data,
},
useFilters
)
return (
<>
<input
value={filterInput}
onChange={e => {
setFilter('firstName', e.target.value)
}}
placeholder={'Search by first name'}
/>
<table {...getTableProps()}>
{/* ... table structure ... */}
</table>
</>
)
}
This example adds a filter input for the “firstName” column.
Conclusion
TanStack Table offers a powerful and flexible solution for creating tables and data grids in React applications. Its headless nature provides unlimited customization possibilities, while its rich feature set covers most data presentation needs. By leveraging TanStack Table, you can build performant, accessible, and visually appealing data displays that enhance the user experience of your React applications.
For more insights on React libraries and components, check out our articles on React Grid Layout for responsive layouts and React Data Table Component for another perspective on data tables in React.