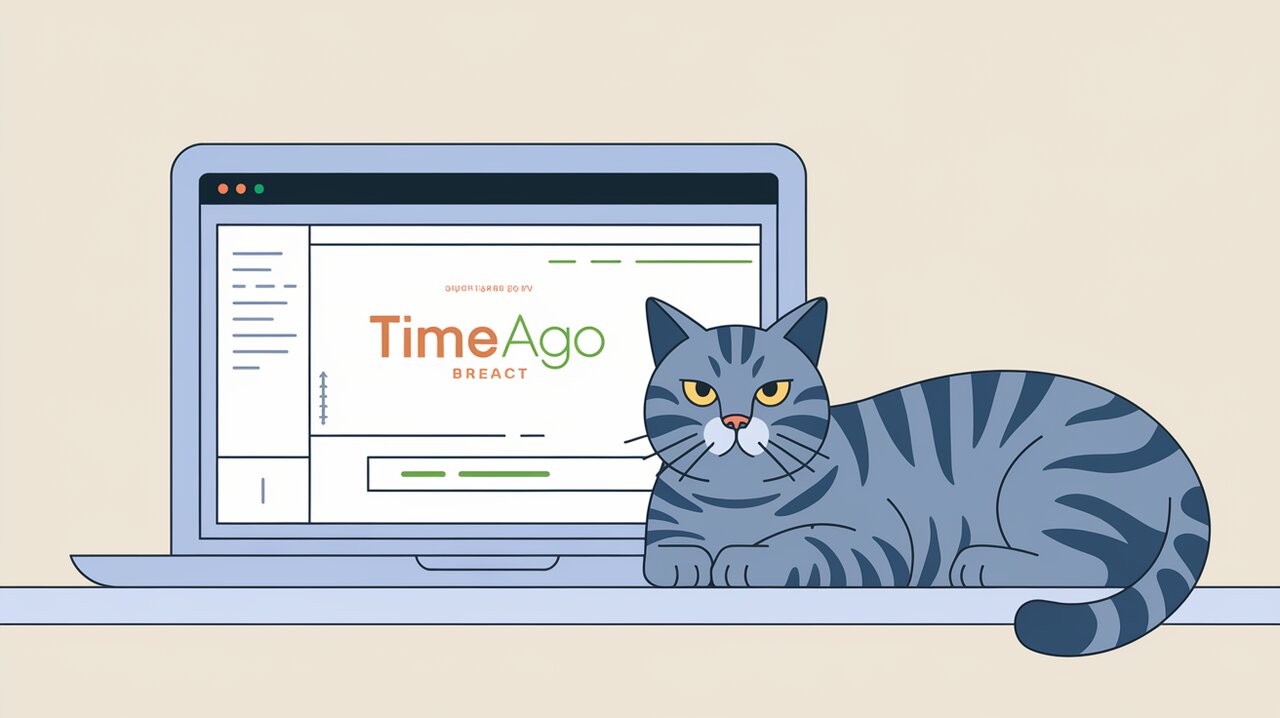
Introduction
In today’s digital landscape, providing users with clear and concise information is paramount. One common requirement is to display timestamps in a way that is both informative and user-friendly. The timeago-react library addresses this need by allowing developers to present dates in a ‘time ago’ format, such as ‘3 hours ago’ or ‘2 days ago’. This library is particularly useful in applications where real-time updates are essential, such as social media platforms, blogs, or news sites.
Key Features of Timeago-React
-
Lightweight: At just around 2kb, it won’t bloat your application.
-
Real-time Rendering: Automatically updates the displayed time as it changes.
-
Locale Support: Easily switch between different languages for internationalization.
Getting Started with Installation
To get started with timeago-react, you can install it using either npm or yarn. Here’s how:
npm install timeago-react
or
yarn add timeago-react
Basic Usage
Simple Integration
Integrating timeago-react into your React application is straightforward. Here’s a basic example of how to use it:
import * as React from 'react';
import TimeAgo from 'timeago-react';
const MyComponent = () => {
return (
<div>
<TimeAgo datetime={'2024-12-20T12:00:00Z'} />
</div>
);
};
In this example, the TimeAgo
component takes a datetime
prop formatted as an ISO string. It will automatically render how long ago that date was from the current time.
Customizing Locale
You can customize the locale used for displaying time by specifying the locale
prop:
import * as React from 'react';
import TimeAgo from 'timeago-react';
const MyComponent = () => {
return (
<div>
<TimeAgo datetime={'2024-12-20T12:00:00Z'} locale='fr' />
</div>
);
};
This will render the time in French, making your application more accessible to French-speaking users.
Advanced Usage
Live Updates
One of the standout features of timeago-react is its ability to update the displayed time automatically. You can enable or disable live rendering using the live
prop:
import * as React from 'react';
import TimeAgo from 'timeago-react';
const MyComponent = () => {
return (
<div>
<TimeAgo datetime={'2024-12-20T12:00:00Z'} live={true} />
</div>
);
};
Setting live
to true
allows the component to refresh every minute, ensuring that users always see the most current information.
Custom Styling
You can also apply custom styles to your TimeAgo
component by using the className
prop:
import * as React from 'react';
import TimeAgo from 'timeago-react';
const MyComponent = () => {
return (
<div>
<TimeAgo datetime={'2024-12-20T12:00:00Z'} className="custom-time" />
</div>
);
};
By adding a class name, you can style your timestamps according to your application’s design guidelines.
Conclusion
The timeago-react library is an excellent choice for developers looking to enhance their applications with user-friendly date formatting. Its lightweight nature, real-time rendering capabilities, and support for multiple locales make it versatile for various use cases. Whether you’re building a social media platform or a blog, integrating this library can significantly improve user experience by providing clear and concise time information.
For further reading on similar libraries and tools that can enhance your React applications, check out articles like React Big Calendar Guide and React Date Picker Wizardry.