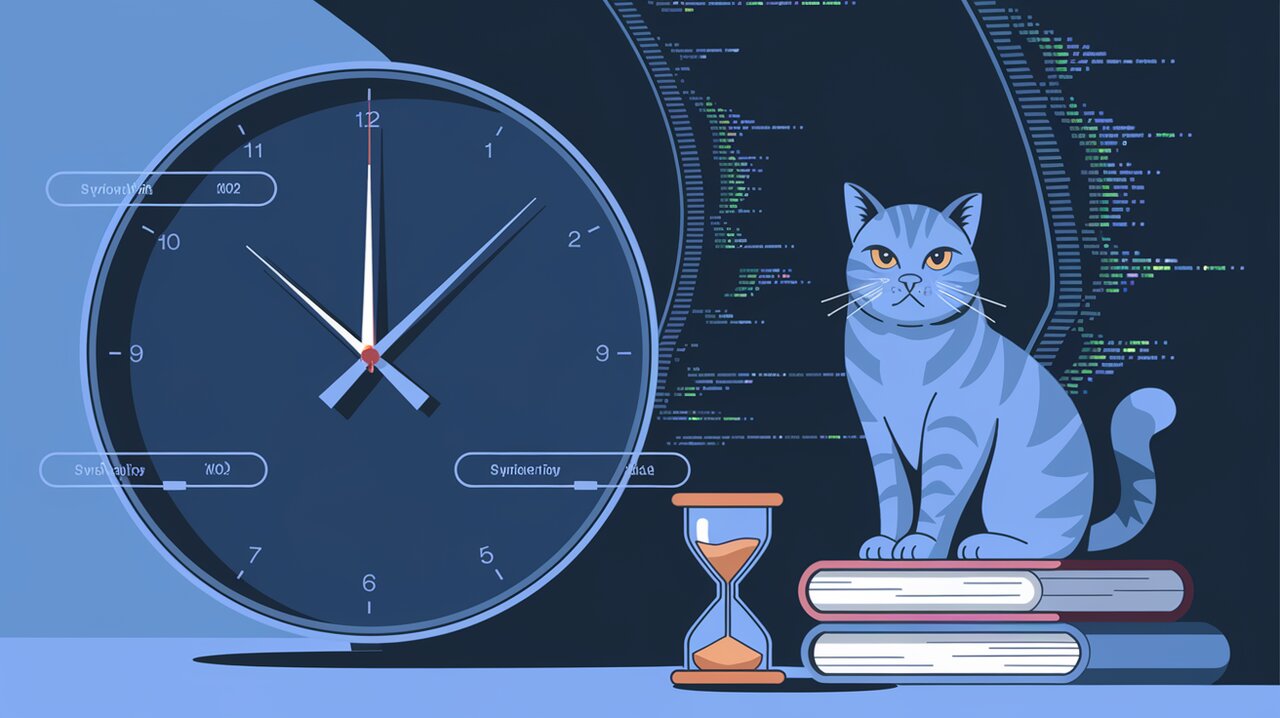
Timefield Tango: Dancing with react-simple-timefield
In the intricate ballet of web development, managing time inputs can often feel like a complex choreography. Enter react-simple-timefield, a nimble partner that pirouettes through the challenges of time entry with grace and precision. This lightweight yet powerful React component transforms the potentially clumsy steps of time input into a smooth, elegant performance.
Stepping into the Timefield
Before we dive into the grand performance, let’s warm up with the basics. react-simple-timefield is designed to simplify time input in React applications. It provides a user-friendly interface for entering time values, complete with format validation and customization options. Whether you’re building a scheduling app, a time tracker, or any interface that requires time input, this library promises to keep you in perfect rhythm.
Features that Keep the Beat
- Format Flexibility: Supports both ‘HH:MM’ and ‘HH:MM:SS’ time formats.
- Customizable Separators: Change the colon to any character you prefer.
- Seconds Support: Optionally include seconds in your time input.
- Input Customization: Use your own input component for a tailored look.
- Controlled Component: Fully controllable for seamless state management.
Setting the Stage: Installation
Let’s begin our performance by setting up react-simple-timefield in your project. You can install it using npm or yarn:
npm install react-simple-timefield
# or if you prefer yarn
yarn add react-simple-timefield
For those working with older versions of React (pre-16), you’ll need to use an earlier version:
npm install react-simple-timefield@1
The Opening Act: Basic Usage
Now that we’ve set the stage, let’s start with a simple routine. Here’s how you can implement a basic time input field:
import React, { useState } from 'react';
import TimeField from 'react-simple-timefield';
const BasicTimeInput: React.FC = () => {
const [time, setTime] = useState('12:00');
const handleTimeChange = (event: React.ChangeEvent<HTMLInputElement>, value: string) => {
setTime(value);
};
return (
<TimeField
value={time}
onChange={handleTimeChange}
/>
);
};
export default BasicTimeInput;
In this simple choreography, we create a controlled component that manages the time state. The TimeField
component takes care of formatting and validating the input, ensuring our performance remains flawless.
Advanced Choreography
Customizing the Separator
Let’s add some flair to our routine by changing the separator:
import React, { useState } from 'react';
import TimeField from 'react-simple-timefield';
const CustomSeparatorTimeInput: React.FC = () => {
const [time, setTime] = useState('12.00');
const handleTimeChange = (event: React.ChangeEvent<HTMLInputElement>, value: string) => {
setTime(value);
};
return (
<TimeField
value={time}
onChange={handleTimeChange}
colon="."
/>
);
};
export default CustomSeparatorTimeInput;
By setting the colon
prop to a dot, we’ve changed the rhythm of our time display. This can be particularly useful for international audiences or specific design requirements.
Including Seconds in the Performance
For those performances that require precision timing, we can include seconds in our time input:
import React, { useState } from 'react';
import TimeField from 'react-simple-timefield';
const TimeWithSecondsInput: React.FC = () => {
const [time, setTime] = useState('12:00:00');
const handleTimeChange = (event: React.ChangeEvent<HTMLInputElement>, value: string) => {
setTime(value);
};
return (
<TimeField
value={time}
onChange={handleTimeChange}
showSeconds
/>
);
};
export default TimeWithSecondsInput;
By adding the showSeconds
prop, we extend our time input to include seconds, perfect for applications that need that extra level of detail.
Customizing the Input Element
For the grand finale, let’s showcase how we can use a custom input element to truly make our time input stand out:
import React, { useState } from 'react';
import TimeField from 'react-simple-timefield';
const CustomInputTimeField: React.FC = () => {
const [time, setTime] = useState('12:00');
const handleTimeChange = (event: React.ChangeEvent<HTMLInputElement>, value: string) => {
setTime(value);
};
const CustomInput: React.FC<React.InputHTMLAttributes<HTMLInputElement>> = (props) => (
<input
{...props}
style={{
fontSize: '1.5em',
padding: '0.5em',
border: '2px solid #007bff',
borderRadius: '4px',
}}
/>
);
return (
<TimeField
value={time}
onChange={handleTimeChange}
input={<CustomInput />}
/>
);
};
export default CustomInputTimeField;
In this spectacular finish, we’ve created a custom input component with unique styling and passed it to the TimeField
component. This allows for complete control over the appearance of our time input while still benefiting from the formatting and validation logic of react-simple-timefield.
The Final Bow
As the curtain falls on our exploration of react-simple-timefield, we’ve seen how this nimble library can transform the often cumbersome task of time input into a graceful performance. From basic implementations to advanced customizations, it provides the flexibility and ease-of-use that modern React developers crave.
Whether you’re orchestrating a simple scheduling interface or composing a complex time-based application, react-simple-timefield offers the tools to keep your user interactions in perfect time. Its simplicity belies its power, making it an invaluable addition to any React developer’s repertoire.
As you continue your journey through the world of React development, consider how react-simple-timefield might complement other time-related functionalities in your applications. For instance, you might pair it with a date picker for full datetime selection, or integrate it into a larger form system for comprehensive data entry.
Remember, in the grand performance of web development, it’s the small, elegant components like react-simple-timefield that often make the difference between a standing ovation and a lackluster reception. So go forth and let your time inputs dance with the grace and precision that your users deserve!