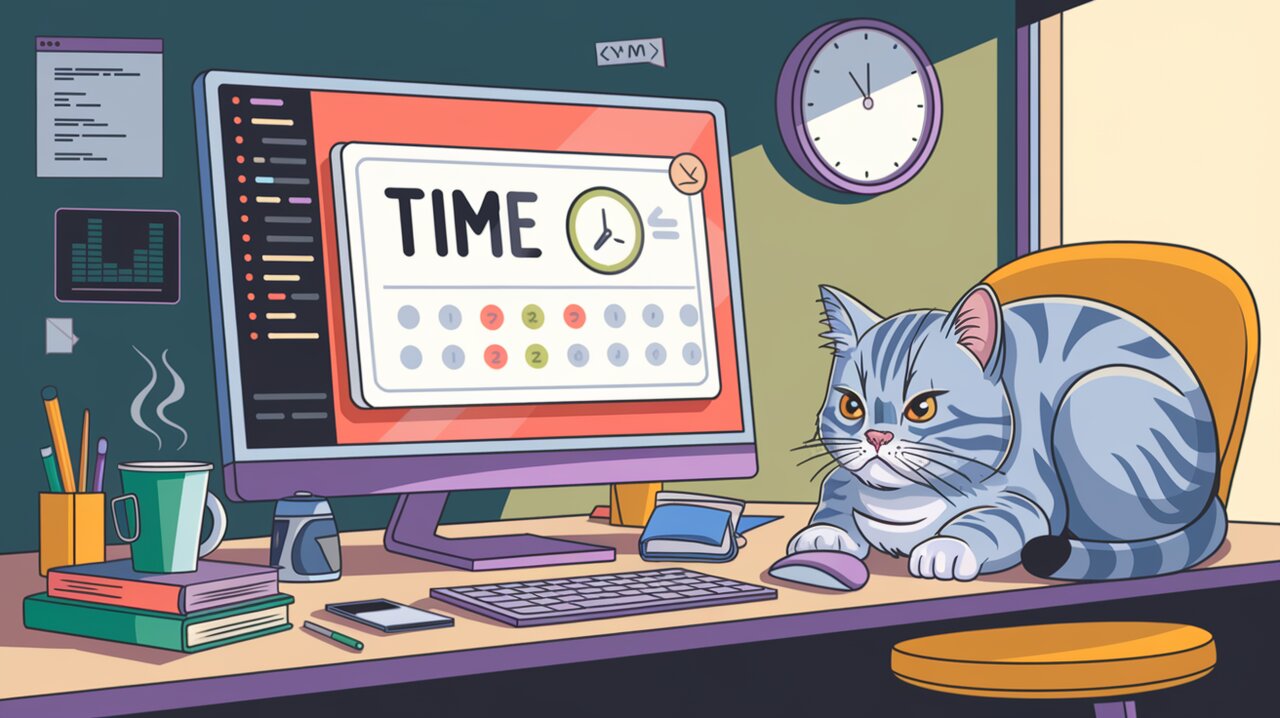
Timekeeping Made Easy: Mastering React Timekeeper
React Timekeeper is a powerful and flexible time picker component for React applications, inspired by the sleek design of Google Keep. This library offers developers an easy way to implement intuitive time selection interfaces with a modern look and feel. Whether you’re building a scheduling app, a reminder system, or any application that requires time input, React Timekeeper provides a user-friendly solution that can be quickly integrated and customized to suit your needs.
Features
React Timekeeper comes packed with a variety of features that make it stand out:
- Support for both 12-hour and 24-hour time formats
- Customizable time display formats
- Smooth animations for enhanced user experience
- Flexible styling options to match your application’s design
- Keyboard accessibility for improved usability
- Touch-friendly interface for mobile devices
Installation
To get started with React Timekeeper, you’ll need to install it in your React project. You can do this using either npm or yarn:
Using npm:
npm install react-timekeeper
Using yarn:
yarn add react-timekeeper
Basic Usage
Once installed, you can start using React Timekeeper in your components. Here’s a simple example of how to implement a basic time picker:
import React, { useState } from 'react';
import TimeKeeper from 'react-timekeeper';
const TimePicker: React.FC = () => {
const [time, setTime] = useState('12:00 pm');
return (
<div>
<TimeKeeper
time={time}
onChange={(newTime) => setTime(newTime.formatted12)}
/>
<p>Selected time: {time}</p>
</div>
);
};
export default TimePicker;
In this example, we import the TimeKeeper
component and use it with a state variable to manage the selected time. The onChange
prop is used to update the state when the user selects a new time.
Customizing Time Format
React Timekeeper allows you to easily switch between 12-hour and 24-hour formats:
import React, { useState } from 'react';
import TimeKeeper from 'react-timekeeper';
const CustomFormatTimePicker: React.FC = () => {
const [time, setTime] = useState('14:30');
return (
<div>
<TimeKeeper
time={time}
onChange={(newTime) => setTime(newTime.formatted24)}
hour24Mode
/>
<p>Selected time: {time}</p>
</div>
);
};
export default CustomFormatTimePicker;
By setting the hour24Mode
prop to true
, we switch to a 24-hour format. The onChange
callback now uses formatted24
to update the state with the 24-hour time string.
Advanced Usage
Customizing Appearance
React Timekeeper offers extensive customization options to match your application’s design:
import React, { useState } from 'react';
import TimeKeeper from 'react-timekeeper';
const StyledTimePicker: React.FC = () => {
const [time, setTime] = useState('10:15 am');
return (
<TimeKeeper
time={time}
onChange={(newTime) => setTime(newTime.formatted12)}
switchToMinuteOnHourSelect
closeOnMinuteSelect
coarseMinutes={15}
forceCoarseMinutes
hourFormat="12"
switchToMinuteOnHourDropdownSelect
closeOnMinuteDropdownSelect
doneButton={() => <button>Done</button>}
cancelButton={() => <button>Cancel</button>}
/>
);
};
export default StyledTimePicker;
This example demonstrates several advanced props:
switchToMinuteOnHourSelect
: Automatically switches to minute selection after an hour is chosencloseOnMinuteSelect
: Closes the picker after a minute is selectedcoarseMinutes
: Sets the minute increments (in this case, 15-minute intervals)forceCoarseMinutes
: Ensures only the specified minute increments can be selectedhourFormat
: Specifies the hour format (12 or 24)doneButton
andcancelButton
: Custom render functions for the action buttons
Controlled Component
For more fine-grained control, you can use React Timekeeper as a controlled component:
import React, { useState } from 'react';
import TimeKeeper from 'react-timekeeper';
const ControlledTimePicker: React.FC = () => {
const [time, setTime] = useState({ hour: 12, minute: 30 });
const handleTimeChange = (newTime: { hour: number; minute: number }) => {
setTime(newTime);
};
return (
<div>
<TimeKeeper
hour={time.hour}
minute={time.minute}
onChange={handleTimeChange}
/>
<p>
Selected time: {time.hour}:{time.minute.toString().padStart(2, '0')}
</p>
</div>
);
};
export default ControlledTimePicker;
In this setup, we manage the hour and minute separately in our component’s state, giving us more control over the time selection process.
Conclusion
React Timekeeper offers a robust solution for implementing time selection in React applications. Its Google Keep-inspired design provides a familiar and intuitive interface for users, while its extensive customization options allow developers to tailor the component to their specific needs. Whether you’re building a simple scheduling tool or a complex time management system, React Timekeeper’s flexibility and ease of use make it an excellent choice for your project.
By leveraging the features and customization options we’ve explored in this article, you can create time picker interfaces that are not only functional but also visually appealing and user-friendly. As you continue to work with React Timekeeper, you’ll discover even more ways to enhance your application’s time selection capabilities, ultimately improving the overall user experience of your React projects.