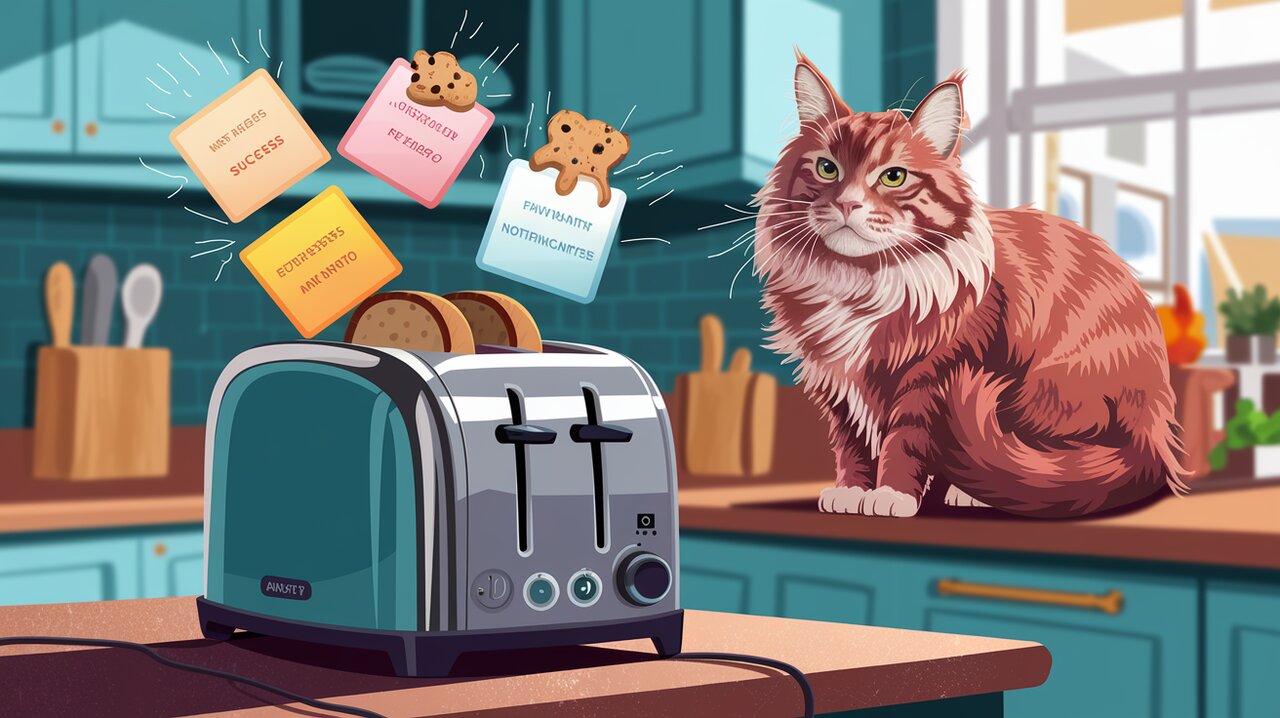
Spice Up Your React App with Toast Notifications: A Flavorful Guide
Serving Up Notifications: An Introduction to react-toast-notifications
In the world of web development, providing users with timely and non-intrusive feedback is crucial for creating a smooth and engaging user experience. Enter React Toast Notifications, a lightweight and flexible library that allows developers to easily implement toast notifications in their React applications. These notifications, named after the way they pop up like toast from a toaster, are perfect for displaying short messages, alerts, or confirmations without disrupting the user’s workflow.
Key Ingredients: Features of react-toast-notifications
React Toast Notifications comes packed with a variety of features that make it a go-to choice for developers:
- Easy Integration: Seamlessly integrates with React applications using hooks or higher-order components.
- Customizable Appearance: Offers flexibility in styling and positioning of notifications.
- Multiple Notification Types: Supports different types of notifications such as success, error, and warning.
- Auto-dismiss: Automatically removes notifications after a specified duration.
- Accessibility: Ensures notifications are accessible to screen readers.
- Stacking: Handles multiple notifications by stacking them neatly.
Preparing the Kitchen: Installation
Before we start cooking up some notifications, let’s get the library installed in your project. You can use either npm or yarn to add react-toast-notifications
to your dependencies.
Using npm:
npm install react-toast-notifications
Using yarn:
yarn add react-toast-notifications
The Basic Recipe: Getting Started with react-toast-notifications
Let’s start with a simple example to get you familiar with the basic usage of React Toast Notifications.
Setting the Table: Wrapping Your App
First, you need to wrap your application (or the part of it where you want to use toast notifications) with the ToastProvider
component. This provider gives context to all the toast notifications in your app.
import React from 'react';
import { ToastProvider } from 'react-toast-notifications';
const App: React.FC = () => {
return (
<ToastProvider>
{/* Your app components go here */}
</ToastProvider>
);
};
export default App;
This setup ensures that toast notifications can be used anywhere within the wrapped components.
Serving the First Toast: Using the useToasts Hook
Now that we’ve set the table, let’s serve our first toast notification. We’ll use the useToasts
hook to add notifications in our functional components.
import React from 'react';
import { useToasts } from 'react-toast-notifications';
const MyComponent: React.FC = () => {
const { addToast } = useToasts();
const handleClick = () => {
addToast('This is a toast notification!', {
appearance: 'success',
autoDismiss: true,
});
};
return <button onClick={handleClick}>Show Toast</button>;
};
In this example, clicking the button will display a success toast notification that automatically dismisses itself. The addToast
function takes two arguments: the message and an options object.
Gourmet Toasts: Advanced Usage and Customization
Now that we’ve mastered the basics, let’s explore some more advanced features and customizations offered by React Toast Notifications.
Flavoring Your Toasts: Customizing Appearance
You can customize the appearance of your toast notifications by passing different options to the addToast
function.
import React from 'react';
import { useToasts } from 'react-toast-notifications';
const AdvancedToastDemo: React.FC = () => {
const { addToast } = useToasts();
const showCustomToast = () => {
addToast('Your file has been successfully uploaded!', {
appearance: 'success',
autoDismiss: true,
autoDismissTimeout: 5000,
placement: 'top-center',
});
};
return <button onClick={showCustomToast}>Upload File</button>;
};
This example shows a success toast that appears at the top-center of the screen and automatically dismisses after 5 seconds.
A Toast for Every Occasion: Different Notification Types
React Toast Notifications supports various types of notifications to convey different kinds of messages.
import React from 'react';
import { useToasts } from 'react-toast-notifications';
const NotificationTypes: React.FC = () => {
const { addToast } = useToasts();
const showSuccessToast = () => {
addToast('Operation successful!', { appearance: 'success' });
};
const showErrorToast = () => {
addToast('An error occurred.', { appearance: 'error' });
};
const showInfoToast = () => {
addToast('Did you know? Toasts are delicious!', { appearance: 'info' });
};
const showWarningToast = () => {
addToast('Caution: Hot surface!', { appearance: 'warning' });
};
return (
<div>
<button onClick={showSuccessToast}>Success</button>
<button onClick={showErrorToast}>Error</button>
<button onClick={showInfoToast}>Info</button>
<button onClick={showWarningToast}>Warning</button>
</div>
);
};
This example demonstrates how to create different types of toast notifications, each with its own appearance to convey the nature of the message.
Custom Toast Recipes: Creating Your Own Toast Components
For even more control over the appearance and behavior of your toast notifications, you can create custom toast components.
import React from 'react';
import { DefaultToast, ToastProvider } from 'react-toast-notifications';
const MyCustomToast: React.FC<any> = ({ children, ...props }) => (
<DefaultToast {...props}>
<div style={{ padding: '8px', border: '1px solid #ccc' }}>
{children}
</div>
</DefaultToast>
);
const App: React.FC = () => (
<ToastProvider components={{ Toast: MyCustomToast }}>
{/* Your app components */}
</ToastProvider>
);
This example shows how to create a custom toast component that wraps the default toast with additional styling. You can then provide this custom component to the ToastProvider
to use it throughout your application.
The Perfect Toast: Best Practices and Tips
To make the most of React Toast Notifications, consider these best practices:
- Keep your messages concise and clear.
- Use appropriate notification types to convey the right level of importance.
- Don’t overuse notifications; reserve them for important information.
- Consider the placement of your notifications to ensure they don’t obstruct important UI elements.
- Test your notifications for accessibility to ensure all users can benefit from them.
Wrapping Up: A Toast to Better User Experience
React Toast Notifications provides a simple yet powerful way to enhance your React applications with informative and stylish notifications. By following this guide, you’ve learned how to integrate toast notifications into your app, customize their appearance, and create different types of notifications to suit various scenarios.
Remember, the key to effective toast notifications is balance – use them judiciously to inform and guide your users without overwhelming them. With React Toast Notifications, you have a versatile tool at your disposal to create a more interactive and user-friendly experience in your React applications.
So go ahead, add some flavor to your app with toast notifications, and watch as your user engagement rises like well-toasted bread!