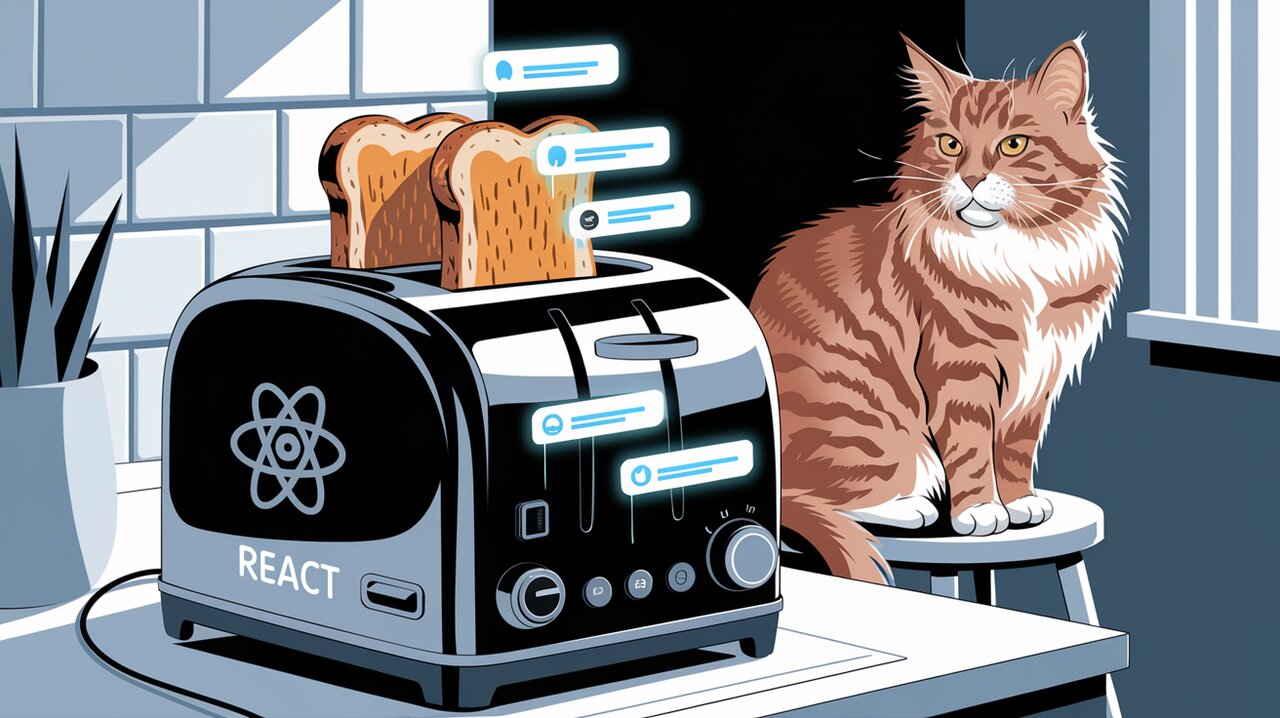
Toast Your React App: Serving Up Notifications with react-toast
React applications often require a way to display brief, non-intrusive messages to users. Enter react-toast
, a lightweight and flexible library that makes implementing toast notifications a breeze. In this article, we’ll explore how to integrate react-toast into your React projects and leverage its features to enhance your user interface.
Features
react-toast
comes packed with several features that make it an attractive choice for developers:
- Minimal setup required
- Customizable positioning
- Adjustable display duration
- Support for different notification types (success, error, info, etc.)
- Easy integration with existing React components
Installation
Getting started with react-toast is straightforward. You can install it using either yarn or npm.
Using yarn:
yarn add react-toast
Using npm:
npm install react-toast
Basic Usage
Let’s dive into how you can start using react-toast
in your React application.
Setting Up the Toast Container
First, you need to add the ToastContainer
component to your app. This component acts as a wrapper for all toast notifications.
import React from 'react';
import { ToastContainer } from 'react-toast';
const App = () => {
return (
<div>
{/* Your app content */}
<ToastContainer />
</div>
);
};
export default App;
Triggering Toast Notifications
Now that we have the container set up, let’s look at how to trigger toast notifications.
import React from 'react';
import { toast } from 'react-toast';
const MyComponent = () => {
const showToast = () => {
toast('Hello, World!');
};
return (
<button onClick={showToast}>
Show Toast
</button>
);
};
export default MyComponent;
This simple example demonstrates how to show a basic toast notification when a button is clicked.
Customizing Toast Appearance
react-toast allows you to customize the appearance of your notifications. Here’s how you can create toasts with different styles:
import React from 'react';
import { toast } from 'react-toast';
const NotificationExamples = () => {
const showSuccessToast = () => {
toast.success('Operation completed successfully!');
};
const showErrorToast = () => {
toast.error('An error occurred. Please try again.');
};
const showInfoToast = () => {
toast.info('Did you know? Toast notifications are awesome!');
};
return (
<div>
<button onClick={showSuccessToast}>Success Toast</button>
<button onClick={showErrorToast}>Error Toast</button>
<button onClick={showInfoToast}>Info Toast</button>
</div>
);
};
export default NotificationExamples;
Advanced Usage
Now that we’ve covered the basics, let’s explore some more advanced features of react-toast.
Customizing Toast Position
You can control where toast notifications appear on the screen:
import React from 'react';
import { ToastContainer, toast } from 'react-toast';
const PositionedToasts = () => {
const showTopRightToast = () => {
toast('I'm at the top right!', { position: 'top-right' });
};
return (
<div>
<button onClick={showTopRightToast}>Top Right Toast</button>
<ToastContainer position="top-right" />
</div>
);
};
export default PositionedToasts;
Adjusting Toast Duration
Control how long a toast notification stays visible:
import React from 'react';
import { toast } from 'react-toast';
const LongLastingToast = () => {
const showLongToast = () => {
toast('I'll stick around for a while', { delay: 10000 });
};
return (
<button onClick={showLongToast}>Show Long-lasting Toast</button>
);
};
export default LongLastingToast;
Custom Styling
You can apply custom styles to your toast notifications for a unique look:
import React from 'react';
import { toast } from 'react-toast';
const StyledToast = () => {
const showStyledToast = () => {
toast('Check out my style!', {
backgroundColor: '#8329C5',
color: '#ffffff',
});
};
return (
<button onClick={showStyledToast}>Show Styled Toast</button>
);
};
export default StyledToast;
Using Toast with Promises
react-toast can be particularly useful when working with asynchronous operations:
import React from 'react';
import { toast } from 'react-toast';
const AsyncToast = () => {
const fetchData = async () => {
try {
const response = await fetch('https://api.example.com/data');
const data = await response.json();
toast.success('Data fetched successfully!');
return data;
} catch (error) {
toast.error('Failed to fetch data');
throw error;
}
};
return (
<button onClick={fetchData}>Fetch Data</button>
);
};
export default AsyncToast;
Conclusion
react-toast
provides a simple yet powerful way to add toast notifications to your React applications. Its ease of use, customization options, and flexibility make it an excellent choice for developers looking to enhance their user interfaces with informative and non-intrusive notifications.
By incorporating react-toast
into your projects, you can keep users informed about the state of their actions, provide feedback, and improve overall user experience. Whether you’re building a small application or a large-scale project, react-toast
offers the tools you need to create effective and stylish notifications.
Remember to explore the official documentation for more advanced features and customization options. Happy toasting!