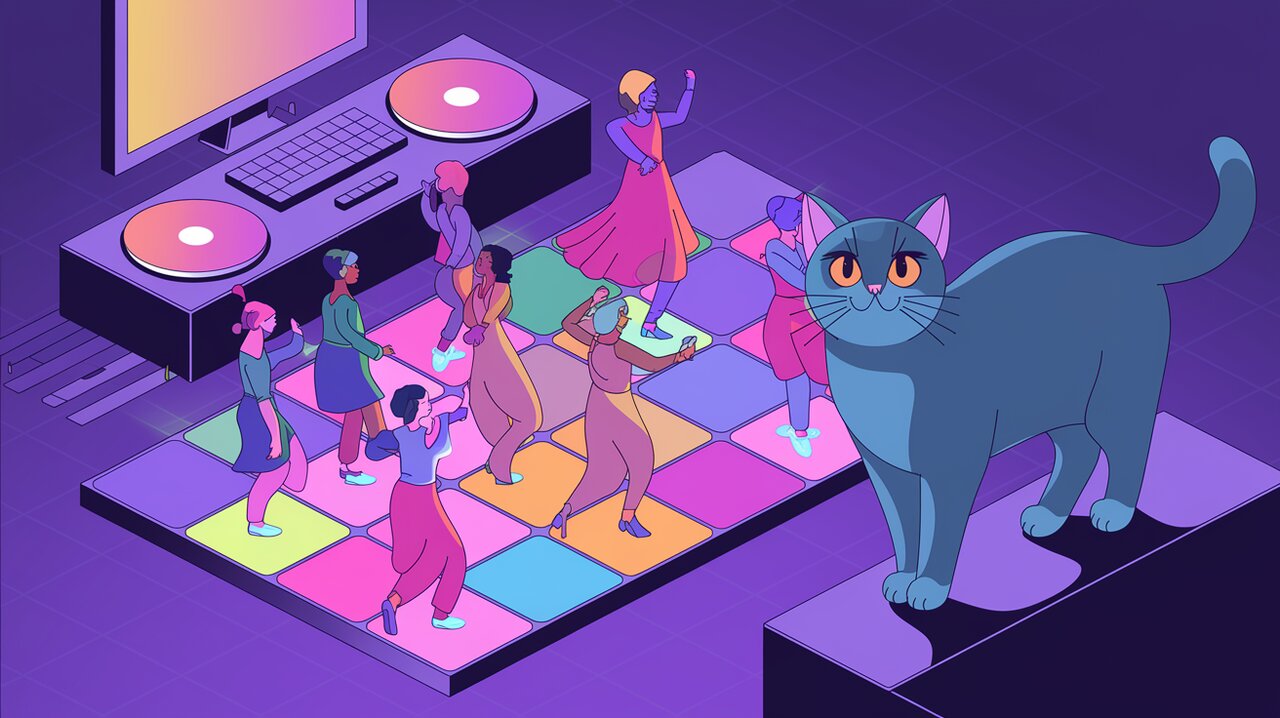
Token Tango: Dancing with react-customize-token-input in React
React-customize-token-input is a powerful and flexible React component that allows developers to create token-based input fields with ease. This library is perfect for scenarios where you need to handle multiple values within a single input, such as tag systems, recipient lists, or any other use case that requires grouping discrete pieces of information. With its customizable nature, React-customize-token-input adapts to your specific needs, allowing you to tailor both the appearance and behavior of your token input component.
Tokenizing Features
React-customize-token-input comes packed with a variety of features that make it stand out:
- Customizable Data Structure: Adapt the token values to fit your application’s data model.
- Flexible Appearance: Customize the look and feel of tokens, including labels and delete buttons.
- Separator Characters: Define custom characters to separate user input into tokens.
- Inline Editing: Allow users to edit existing tokens directly within the input field.
- Paste Support: Seamlessly handle pasted values, converting them into tokens.
- Input Preprocessing: Normalize and validate user input before creating tokens.
- Accessibility: Ensure your token input is usable for all users, including those relying on assistive technologies.
Setting Up Your Token Stage
To begin your token tango, you’ll need to install the react-customize-token-input
package. You can do this using either npm or yarn:
Using npm:
npm install react-customize-token-input
Using yarn:
yarn add react-customize-token-input
Basic Steps of the Token Dance
Let’s start with a simple implementation of React-customize-token-input to get you moving:
The Opening Move
First, import the component and set up a basic state to manage your tokens:
import React, { useState } from 'react';
import TokenInput from 'react-customize-token-input';
const TokenInputExample: React.FC = () => {
const [tokens, setTokens] = useState<string[]>([]);
const handleTokenAdd = (newTokens: string[]) => {
setTokens([...tokens, ...newTokens]);
};
return (
<TokenInput
tokens={tokens}
onTokenAdd={handleTokenAdd}
placeholder="Enter tags..."
/>
);
};
export default TokenInputExample;
In this basic setup, we’re using the TokenInput
component with a simple array of strings as our tokens. The onTokenAdd
callback updates our state with new tokens as they’re added.
Customizing the Rhythm
Now, let’s add some custom styling to our tokens:
import React, { useState } from 'react';
import TokenInput from 'react-customize-token-input';
const TokenInputExample: React.FC = () => {
const [tokens, setTokens] = useState<string[]>([]);
const handleTokenAdd = (newTokens: string[]) => {
setTokens([...tokens, ...newTokens]);
};
const customTokenComponent = ({ token, onDelete }: { token: string; onDelete: () => void }) => (
<span className="custom-token">
{token}
<button onClick={onDelete}>×</button>
</span>
);
return (
<TokenInput
tokens={tokens}
onTokenAdd={handleTokenAdd}
placeholder="Enter tags..."
customizeTokenComponent={customTokenComponent}
/>
);
};
export default TokenInputExample;
Here, we’ve added a customTokenComponent
that defines how each token should be rendered. This allows you to create tokens that match your application’s design language.
Advanced Choreography
As you become more comfortable with the basic steps, it’s time to explore some of the more advanced moves in our token tango.
The Preprocessing Pirouette
Sometimes, you may want to preprocess user input before creating tokens. Here’s how you can implement a custom onBuildTokenValue
function:
import React, { useState } from 'react';
import TokenInput from 'react-customize-token-input';
interface CustomToken {
id: string;
value: string;
}
const TokenInputExample: React.FC = () => {
const [tokens, setTokens] = useState<CustomToken[]>([]);
const handleTokenAdd = (newTokens: CustomToken[]) => {
setTokens([...tokens, ...newTokens]);
};
const onBuildTokenValue = (inputString: string): CustomToken => {
return {
id: Date.now().toString(),
value: inputString.trim().toLowerCase()
};
};
return (
<TokenInput
tokens={tokens}
onTokenAdd={handleTokenAdd}
onBuildTokenValue={onBuildTokenValue}
placeholder="Enter tags..."
/>
);
};
export default TokenInputExample;
In this example, we’re creating custom token objects with an ID and a normalized value. The onBuildTokenValue
function trims whitespace and converts the input to lowercase before creating the token.
The Validation Waltz
Adding validation to your token input ensures that only appropriate values become tokens. Here’s how you can implement a custom validation function:
import React, { useState } from 'react';
import TokenInput from 'react-customize-token-input';
const TokenInputExample: React.FC = () => {
const [tokens, setTokens] = useState<string[]>([]);
const handleTokenAdd = (newTokens: string[]) => {
setTokens([...tokens, ...newTokens]);
};
const validateToken = (value: string): boolean => {
// Only allow tokens that are at least 3 characters long
return value.length >= 3;
};
return (
<TokenInput
tokens={tokens}
onTokenAdd={handleTokenAdd}
onValidateToken={validateToken}
placeholder="Enter tags (min 3 characters)..."
/>
);
};
export default TokenInputExample;
This validation function ensures that only tokens with at least three characters are accepted. You can customize this function to implement any validation logic your application requires.
The Separator Samba
React-customize-token-input allows you to define custom separator characters. This is useful when you want to allow users to input multiple tokens at once:
import React, { useState } from 'react';
import TokenInput from 'react-customize-token-input';
const TokenInputExample: React.FC = () => {
const [tokens, setTokens] = useState<string[]>([]);
const handleTokenAdd = (newTokens: string[]) => {
setTokens([...tokens, ...newTokens]);
};
return (
<TokenInput
tokens={tokens}
onTokenAdd={handleTokenAdd}
placeholder="Enter tags (separate with comma or semicolon)..."
tokenSeparators={[',', ';']}
/>
);
};
export default TokenInputExample;
In this example, users can separate tokens using either a comma or a semicolon, allowing for more flexible input methods.
The Grand Finale
React-customize-token-input provides a powerful and flexible solution for implementing token-based inputs in your React applications. From basic setups to advanced customizations, this library offers the tools you need to create intuitive and user-friendly token input components.
By leveraging features like custom token components, input preprocessing, validation, and custom separators, you can tailor the token input experience to match your specific requirements. Whether you’re building a tagging system, a recipient list for messaging, or any other interface that requires grouping discrete pieces of information, React-customize-token-input offers the flexibility and functionality to bring your vision to life.
As you continue to explore the capabilities of this library, remember that the key to a great user experience lies in finding the right balance between functionality and simplicity. With React-customize-token-input, you have the power to create token input components that are both powerful and intuitive, enhancing the overall usability of your React applications.
For more insights on enhancing your React applications with powerful components, check out our articles on Autocomplete Wizardry: react-autocomplete-input and Animate Text Transitions: react-text-transition. These complementary tools can further enrich your development toolkit and streamline your workflow.