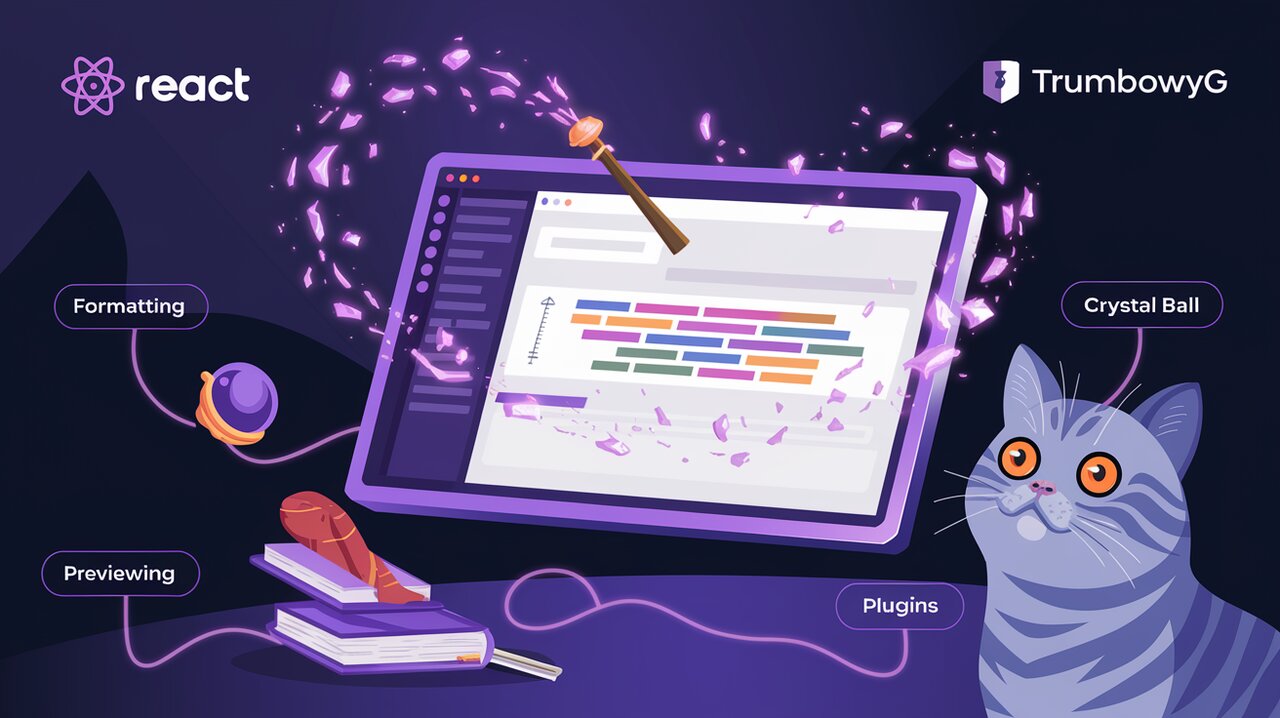
Trumbowyg Wizardry: Conjuring Rich Text Editors in React
In the realm of React development, crafting intuitive user interfaces for content creation can be a challenging feat. Enter react-trumbowyg, a powerful wrapper for the lightweight Trumbowyg WYSIWYG editor that brings the magic of rich text editing to your React applications. This enchanting library allows developers to conjure up sophisticated editing experiences with minimal effort, making it an invaluable tool in any React wizard’s spellbook.
Unveiling the Magical Features
React-trumbowyg comes packed with an array of spellbinding capabilities that set it apart from other WYSIWYG editors:
- Lightweight Enchantment: At less than 30kb, it won’t weigh down your application like a heavy grimoire.
- Semantic Sorcery: Generates clean, semantic HTML that even the most discerning search engine familiars will appreciate.
- Customizable Appearance: Easily alter the editor’s appearance to match your application’s aesthetic, like a chameleon charm.
- Plugin Potions: Extend functionality with a variety of plugins, each adding new powers to your editing arsenal.
- Multilingual Mastery: Supports over 40 languages, allowing your editor to communicate in tongues from around the world.
Summoning the Editor
To begin your journey with react-trumbowyg, you’ll need to summon it into your project. Open your terminal and chant the following incantations:
npm install react-trumbowyg
# or if you prefer the yarn school of magic
yarn add react-trumbowyg
Casting the Basic Spell
Once you’ve acquired the necessary magical components, it’s time to cast your first spell. Here’s a basic incantation to conjure a simple editor:
import React from 'react';
import Trumbowyg from 'react-trumbowyg';
import 'react-trumbowyg/dist/trumbowyg.min.css';
const SimpleEditor: React.FC = () => {
return (
<Trumbowyg
id='my-editor'
buttons={[
['viewHTML'],
['formatting'],
['strong', 'em', 'del'],
['link'],
['insertImage'],
['justifyLeft', 'justifyCenter', 'justifyRight', 'justifyFull'],
['unorderedList', 'orderedList'],
['horizontalRule'],
['removeformat'],
['fullscreen']
]}
/>
);
};
export default SimpleEditor;
This spell creates a basic editor with a selection of common formatting tools. The buttons
prop allows you to customize which editing options appear in the toolbar, much like selecting ingredients for a potion.
Advanced Incantations
Binding to the Editor’s Events
To harness the full power of react-trumbowyg, you’ll want to bind to its various events. This allows your application to react to changes in the editor’s content or state:
import React from 'react';
import Trumbowyg from 'react-trumbowyg';
const AdvancedEditor: React.FC = () => {
const handleInit = () => {
console.log('Editor initialized');
};
const handleChange = (e: Event) => {
console.log('Content changed:', e.target.innerHTML);
};
return (
<Trumbowyg
id='advanced-editor'
buttons={[
['viewHTML'],
['formatting'],
['strong', 'em', 'del'],
['link'],
['insertImage'],
['justifyLeft', 'justifyCenter', 'justifyRight', 'justifyFull'],
['unorderedList', 'orderedList'],
['horizontalRule'],
['removeformat'],
['fullscreen']
]}
onInit={handleInit}
onChange={handleChange}
/>
);
};
export default AdvancedEditor;
In this enchanted version, we’ve added onInit
and onChange
event handlers. These act like magical sensors, alerting you when the editor has been fully conjured or when its contents have been altered.
Summoning Plugins
To extend the editor’s capabilities, you can call upon additional plugins. Here’s how to invoke the table plugin, allowing users to insert and manipulate tables within the editor:
import React from 'react';
import Trumbowyg from 'react-trumbowyg';
import 'trumbowyg/dist/plugins/table/trumbowyg.table.min.js';
import 'trumbowyg/dist/plugins/table/ui/trumbowyg.table.min.css';
const TableEditor: React.FC = () => {
return (
<Trumbowyg
id='table-editor'
buttons={[
['viewHTML'],
['formatting'],
['strong', 'em', 'del'],
['link'],
['insertImage'],
['justifyLeft', 'justifyCenter', 'justifyRight', 'justifyFull'],
['unorderedList', 'orderedList'],
['table'], // Add the table button
['horizontalRule'],
['removeformat'],
['fullscreen']
]}
/>
);
};
export default TableEditor;
By importing the table plugin and adding the 'table'
button to our array, we’ve expanded the editor’s repertoire to include table creation and editing.
Customizing the Editor’s Appearance
Like a skilled illusionist, you can alter the editor’s appearance to match your application’s theme. Here’s how to apply a dark theme to your editor:
import React from 'react';
import Trumbowyg from 'react-trumbowyg';
import 'react-trumbowyg/dist/trumbowyg.min.css';
import 'react-trumbowyg/dist/ui/trumbowyg.dark.min.css';
const DarkEditor: React.FC = () => {
return (
<Trumbowyg
id='dark-editor'
buttons={[
['viewHTML'],
['formatting'],
['strong', 'em', 'del'],
['link'],
['insertImage'],
['justifyLeft', 'justifyCenter', 'justifyRight', 'justifyFull'],
['unorderedList', 'orderedList'],
['horizontalRule'],
['removeformat'],
['fullscreen']
]}
options={{
semantic: false,
resetCss: true,
removeformatPasted: true,
autogrow: true
}}
/>
);
};
export default DarkEditor;
By importing the dark theme CSS and setting some options, we’ve transformed our editor into a sleek, dark-themed version that’s perfect for night owl developers or applications with darker aesthetics.
Conclusion
React-trumbowyg is a powerful ally in the quest to create rich, interactive content editing experiences within React applications. Its lightweight nature, extensive customization options, and plugin ecosystem make it a versatile choice for developers of all skill levels. Whether you’re crafting a simple blog editor or a complex content management system, react-trumbowyg provides the tools you need to bring your vision to life.
As you continue to explore the depths of this magical library, remember that the true power lies in how you wield it. Experiment with different configurations, explore the plugin ecosystem, and don’t be afraid to push the boundaries of what’s possible. With react-trumbowyg in your arsenal, you’re well-equipped to create editing experiences that will enchant and delight your users.