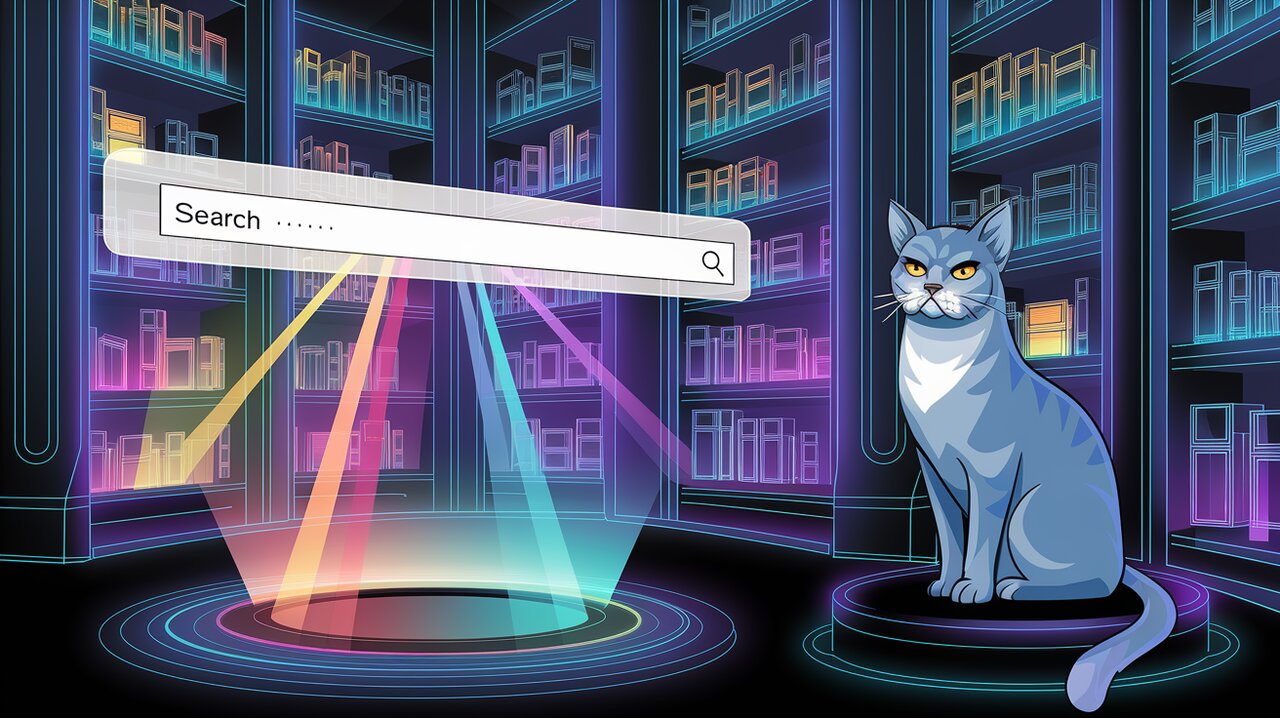
Supercharge Your React Search with Rainbow-Powered Awesomeness
In the ever-evolving landscape of React development, creating an intuitive and visually appealing search functionality is crucial for enhancing user experience. React-Searchbox-Awesome is a powerful library that simplifies this process, offering developers a feature-rich and customizable searchbox component. This library stands out with its ability to create stunning, rainbow-colored search interfaces that not only look great but also provide efficient search capabilities.
Elevate Your Search Experience
React-Searchbox-Awesome comes packed with features that set it apart from conventional search components:
- Rainbow-colored search interface for a unique visual appeal
- Customizable styling options to match your application’s design
- Responsive design that works seamlessly across devices
- Easy integration with existing React projects
Getting Started with React-Searchbox-Awesome
Installation
To begin using React-Searchbox-Awesome in your project, you can install it via npm or yarn:
npm install react-searchbox-awesome
or
yarn add react-searchbox-awesome
Basic Implementation
Let’s dive into a simple example of how to use React-Searchbox-Awesome in your React application:
import React from 'react';
import { SearchBox } from 'react-searchbox-awesome';
const App: React.FC = () => {
const handleSearch = (query: string) => {
console.log('Search query:', query);
// Implement your search logic here
};
return (
<div>
<h1>My Awesome Search App</h1>
<SearchBox onSearch={handleSearch} />
</div>
);
};
export default App;
In this basic example, we import the SearchBox
component from react-searchbox-awesome
and use it within our App
component. The onSearch
prop is a callback function that receives the search query as a parameter, allowing you to implement your custom search logic.
Customizing the Appearance
One of the standout features of React-Searchbox-Awesome is its customizable appearance. Here’s how you can modify the look of your searchbox:
import React from 'react';
import { SearchBox } from 'react-searchbox-awesome';
const App: React.FC = () => {
return (
<div>
<SearchBox
placeholder="Search for awesome things..."
backgroundColor="#f0f0f0"
textColor="#333"
borderColor="#007bff"
rainbowEffect={true}
/>
</div>
);
};
export default App;
This example demonstrates how to customize the placeholder text, background color, text color, and border color of the searchbox. The rainbowEffect
prop enables the eye-catching rainbow animation that gives React-Searchbox-Awesome its unique flair.
Advanced Usage and Features
Implementing Autocomplete
React-Searchbox-Awesome supports autocomplete functionality, enhancing the user experience by providing suggestions as they type:
import React, { useState } from 'react';
import { SearchBox } from 'react-searchbox-awesome';
const App: React.FC = () => {
const [suggestions, setSuggestions] = useState<string[]>([]);
const handleInputChange = (query: string) => {
// Simulating API call for suggestions
const mockSuggestions = ['Apple', 'Banana', 'Cherry', 'Date']
.filter(item => item.toLowerCase().includes(query.toLowerCase()));
setSuggestions(mockSuggestions);
};
return (
<div>
<SearchBox
onInputChange={handleInputChange}
suggestions={suggestions}
onSuggestionSelect={(selected) => console.log('Selected:', selected)}
/>
</div>
);
};
export default App;
This example shows how to implement a basic autocomplete feature. The onInputChange
prop is used to update suggestions based on the user’s input, while suggestions
provides the list of autocomplete options.
Integrating with External Data Sources
For more complex applications, you might want to integrate React-Searchbox-Awesome with external data sources or APIs:
import React, { useState, useEffect } from 'react';
import { SearchBox } from 'react-searchbox-awesome';
const App: React.FC = () => {
const [results, setResults] = useState<any[]>([]);
const handleSearch = async (query: string) => {
try {
const response = await fetch(`https://api.example.com/search?q=${query}`);
const data = await response.json();
setResults(data);
} catch (error) {
console.error('Search error:', error);
}
};
return (
<div>
<SearchBox
onSearch={handleSearch}
debounceTime={300}
/>
<ul>
{results.map((item, index) => (
<li key={index}>{item.title}</li>
))}
</ul>
</div>
);
};
export default App;
In this advanced example, we’re using an external API to fetch search results. The debounceTime
prop helps to optimize performance by limiting the rate of API calls as the user types.
Customizing Search Behavior
React-Searchbox-Awesome allows for fine-tuning of the search behavior to meet specific requirements:
import React from 'react';
import { SearchBox } from 'react-searchbox-awesome';
const App: React.FC = () => {
return (
<div>
<SearchBox
minQueryLength={3}
maxResults={10}
caseSensitive={false}
fuzzySearch={true}
highlightMatches={true}
/>
</div>
);
};
export default App;
This configuration sets a minimum query length, limits the number of results, enables case-insensitive and fuzzy searching, and highlights matching text in the results.
Wrapping Up
React-Searchbox-Awesome is a versatile and powerful library that can significantly enhance the search functionality in your React applications. Its combination of visual appeal and robust features makes it an excellent choice for developers looking to create engaging and efficient search interfaces.
By leveraging the customization options, integrating with external data sources, and fine-tuning the search behavior, you can create a search experience that not only looks great but also provides valuable functionality to your users. Whether you’re building a small project or a large-scale application, React-Searchbox-Awesome offers the flexibility and performance to meet your search implementation needs.
As you continue to explore the capabilities of this library, you’ll discover even more ways to optimize and enhance your search functionality, ultimately creating a more engaging and user-friendly React application.