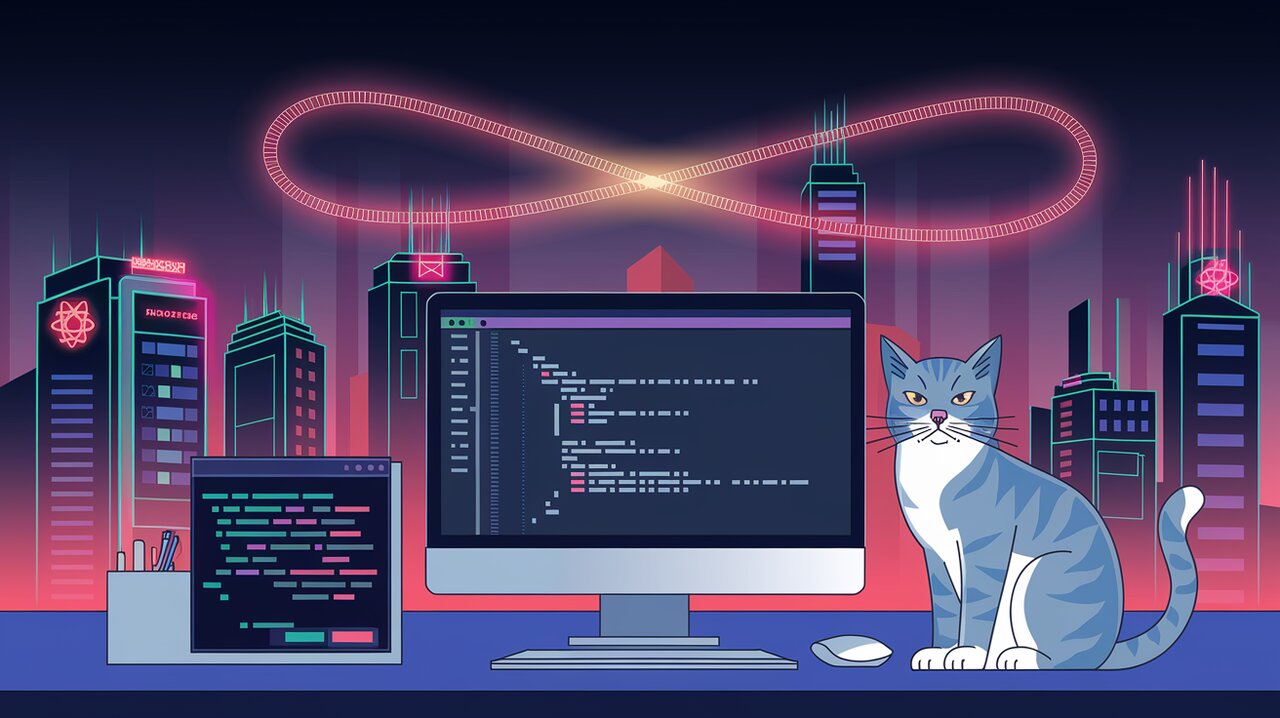
React Linkify is a powerful React component that automatically transforms plain text URLs, email addresses, and other linkable patterns into clickable links. This library simplifies the process of making your text content more interactive and user-friendly, saving developers time and effort in manually creating hyperlinks.
Features
- Automatic detection and conversion of URLs and email addresses
- Support for nested React elements
- Customizable link component and properties
- Ability to handle various link types (e.g., hashtags, mentions)
Installation
To get started with react-linkify
, you need to install it in your React project. You can use either npm or yarn for installation:
Using npm:
npm install react-linkify --save
Using yarn:
yarn add react-linkify
Basic Usage
Once installed, you can start using react-linkify
in your React components. Here’s a simple example of how to use it:
Simple Text Conversion
import React from 'react';
import Linkify from 'react-linkify';
const SimpleExample: React.FC = () => {
return (
<Linkify>
Check out our website at www.example.com or contact us at info@example.com.
</Linkify>
);
};
export default SimpleExample;
In this example, the URLs and email addresses within the text will be automatically converted into clickable links. The resulting output will have <a>
tags wrapped around “www.example.com” and “info@example.com”.
Handling Nested Elements
React Linkify can also handle nested elements, making it versatile for more complex layouts:
import React from 'react';
import Linkify from 'react-linkify';
const NestedExample: React.FC = () => {
return (
<Linkify>
<div>
<h2>Contact Information</h2>
<p>Visit our website: example.com</p>
<p>Email us: <strong>support@example.com</strong></p>
</div>
</Linkify>
);
};
export default NestedExample;
In this case, react-linkify
will traverse the nested elements and convert the URLs and email addresses into links, regardless of their position in the DOM tree.
Advanced Usage
React Linkify offers several advanced features that allow for greater customization and control over how links are rendered and behaved.
Custom Link Component
You can specify a custom component to be used for rendering links instead of the default <a>
tag:
import React from 'react';
import Linkify from 'react-linkify';
import { Link } from 'react-router-dom';
const CustomLinkExample: React.FC = () => {
return (
<Linkify component={Link}>
Check out our about page at /about or our contact page at /contact.
</Linkify>
);
};
export default CustomLinkExample;
In this example, react-linkify
will use the Link
component from react-router-dom to render internal links.
Custom Link Properties
You can add custom properties to the generated links using the properties
prop:
import React from 'react';
import Linkify from 'react-linkify';
const CustomPropertiesExample: React.FC = () => {
const linkProperties = {
target: '_blank',
rel: 'noopener noreferrer',
className: 'custom-link'
};
return (
<Linkify properties={linkProperties}>
Visit our partner site at partner.example.com for more information.
</Linkify>
);
};
export default CustomPropertiesExample;
This will add the specified properties to all generated links, making them open in a new tab and applying a custom CSS class.
Customizing Link Detection
React Linkify uses the linkify-it
library under the hood, which allows for customization of link detection. You can access the global Linkify
instance to add custom protocols or modify existing ones:
import React from 'react';
import Linkify, { linkify } from 'react-linkify';
// Add a custom protocol
linkify.registerCustomProtocol('myapp');
const CustomProtocolExample: React.FC = () => {
return (
<Linkify>
Open the app using this link: myapp://open/dashboard
</Linkify>
);
};
export default CustomProtocolExample;
This example registers a custom protocol “myapp”, allowing react-linkify
to recognize and convert links using this protocol into clickable links.
Handling Special Link Types
For special link types like hashtags or mentions, you can use a custom render function:
import React from 'react';
import Linkify from 'react-linkify';
const SpecialLinksExample: React.FC = () => {
const customRender = ({ attributes, content }) => {
const { href, ...props } = attributes;
if (content.startsWith('#')) {
return <a href={`/hashtag/${content.substr(1)}`} {...props}>{content}</a>;
} else if (content.startsWith('@')) {
return <a href={`/user/${content.substr(1)}`} {...props}>{content}</a>;
}
return <a href={href} {...props}>{content}</a>;
};
return (
<Linkify options={{ render: customRender }}>
Check out #reactjs and follow @reactjs for updates!
</Linkify>
);
};
export default SpecialLinksExample;
This example demonstrates how to handle hashtags and mentions by providing a custom render function that creates appropriate links for these special types.
Conclusion
React Linkify is a versatile and powerful tool for automatically converting plain text into interactive links in React applications. Its ease of use, coupled with advanced customization options, makes it an excellent choice for developers looking to enhance the user experience of their text-heavy components.
By leveraging react-linkify
, you can save development time, improve accessibility, and create more engaging interfaces with minimal effort. Whether you’re working on a simple blog or a complex social media application, react-linkify
provides the flexibility and functionality needed to handle a wide range of linking scenarios.
As you integrate react-linkify
into your projects, remember to consider accessibility and user experience. Always provide clear visual cues for links and ensure that the behavior of automatically generated links aligns with user expectations. With these considerations in mind, react-linkify
can significantly enhance the interactivity and usability of your React applications.